?
?
相關函數列表
//passwd結構體 /etc/passwd struct passwd { char *pw_name; //用戶名 char *pw_passwd; //加密口令 uid_t pw_uid; //數值用戶ID gid_t pw_gid; //數值組ID char *pw_gecos; //注釋字段 char *pw_dir; //初始化工作目錄 char *pw_shell; //初始shell(用戶程序) char *pw_class; //用戶訪問類 time_t pw_change //下次更改口令時間 time_t pw_expire //賬戶有效期時間 }; //陰影口令結構體 /etc/shadow struct spwd { char *sp_namp; //用戶登錄名 char *sp_pwdp; //加密口令 int sp_lstchg; //上次更改口令以來經過的時間 int sp_min; //經多少天后允許更改 int sp_max; //要求更改尚余天數 int sp_warn; //超期警告天數 int sp_inact; //賬戶不活動之前尚余天數 int sp_expire; //賬戶超期天數 unisgned int sp_flag; //保留 }; //組文件結構體 /etc/group struct group { char *gr_name; //組名 char *gr_paswd; //加密口令 int gr_gid; //數值組ID char **gr_mem; //指向各用戶名指針的數組 }; //兩個獲取口令文件項的函數 #include <pwd.h> struct passwd *getpwuid(uid_t uid); struct passwd *getpwnam(const char *name); //查看整個口令文件,調用getpwent時,返回口令文件中的下一個記錄項 #include <pwd.h> struct passwd *getpwent(void); void setpwent(void); //反繞所使用的文件 void endpwent(void); //關閉這些文件 //訪問口令文件的相關函數 #include <shadow.h> struct spwd *getspnam(const char *name); struct spwd *getspent(void); void setspent(void); void endspent(void); //訪問組相關函數 #include <grp.h> struct group *getgrgid(gid_t gid); struct group *getgrnam(const char *name); //類似于獲取所有口令文件和關閉文件 #include <grp.h> struct group *getgrent(void); void setgrent(void); void endgrent(void); //獲取和設置附屬組ID #include <unistd.h> int getgroups(int gidsetsize, gid_t grouplist[]); //若成功返回附屬組ID,出錯返回-1 #include <grp.h> //on linux #include <unistd.h> //on freeBSD int setgroups(int ngroups, const gid_t grouplist[]); #include <grp.h> //on linux #include <unistd.h> //on freeBSD int initgroups(const char *username, gid_t basegid); //大多數UNIX系統都提供了下列兩個數據文件 //utmp文件記錄當前登錄到系統的各個用戶 //wtmp文件跟蹤各個登錄和注銷事件 struct utmp { char ut_line[8]; //tty line, tty0,tty1,tty2 char ut_name[8]; //login name long ut_time; //seconds since Epoch }; //返回主機和操作系統有關的信息 #include <sys/utsname.h> int uname(struct utsname *name); //utsname結構體 struct utsname { char sysname[]; //操作系統名稱 char nodename[]; //節點的名稱 char release[]; //當前release的操作系統版本 char version[]; //當前release的版本 char machine[]; //硬件類型 }; //BSD派生的系統提供了gethostname函數,它只返回主機名 #include <unistd.h> int gethostname(char *name, int namelen); //返回當前時間和日志 #include <time.h> time_t time(time_t *calptr); //可獲取指定時鐘的時間,把時間表示為秒和納秒 #include <sys/time.h> int clock_gettime(clockid_t clock_id, struct timespec *tsp); //此函數把參數tsp指向的timespec結構初始化為與clock_id參數對應的時鐘精度 #include <sys/time.h> int clock_getres(clockid_t clock_id, const struct timespec *tsp); //精確到微秒 #include <sys/time.h> int gettimeofday(struct timeval *restrict tp, void *restrict tzp); //兩個函數的localtime和gmtime將日歷時間轉換成分解的時間,并將這些放在一個tm結構中 struct tm{ int tm_sec; int tm_min; int tm_hour; int tm_mday; int tm_mon; int tm_year; int tm_wday; int tm_yday; int tm_isdst; }; //將日歷時間轉換成本地時間 #include <time.h> struct tm *gettime(const time_t *calptr); //將日歷時間轉換成協調統一時間的年、月、日、時、分、秒 #include <time.h> struct tm *localtime(const time_t *calptr); //以本地時間的年、月、日等作為參數,將其轉換成time_t值 #include <time.h> time_t mktime(struct tm *tmptr); //類似于printf的時間函數,非常復雜, 可以通過可用的多個參數來定制產生的字符串 #include <time.h> size_t strftime(char *restrict buf, size_t maxsize, const char *restrict format, const struct tm *restrict tmptr); size_t strftime_1(char *restrict buf, size_t maxsize, const char *restrict format, const struct tm *restrict tmptr, locale_t locale); //strptime函數是strftime的反過來版本,把字符串時間轉換成分解時間 #include <time.h> char *strptime(const char *restrict buf, const char *restrict format, struct tm *restrict tmptr);
?
?
訪問系統數據文件的一些歷程
說明 | 數據文件 | 頭文件 | 結構 | 附加的鍵搜索函數 |
口令 | /etc/passwd | <pwd.h> | passwd | getpwnam,getpwuid |
組 | /etc/group | <grp.h> | group | getgrnam,getgrgid |
陰影 | /etc/shadow | <shadow.h> | spwd | getspnam |
主機 | /etc/hosts | <netdb.h> | hostent | getnameinfo,getaddrinfo |
網絡 | /etc/networks | <netdb.h> | netent | getnetbyname,getnetbyaddr |
協議 | /etc/protocols | <netdb.h> | protoent | getprotobyname,getprotobynumber |
服務 | /etc/services | <netdb.h> | servent | getservbyname,getservbyport |
?
?
標識符 | 選項 | 說明 |
CLOCCK_REALTIME | ? | 實時系統時間 |
CLOCK_MONOTONIC | _POSIX_MONOTONIC_CLOCK | 不帶負跳的實時系統時間 |
CLOCK_PROCESS_CPUTIME_ID | _POSIX_CPUTIME | 調用進程的CPU時間 |
CLOCK_THREAD_CPUTIME_ID | _POSIX_THREAD_CPUTIME | 調用線程的CPU時間 |
?
各個時間函數之間的關系如下
?
?
讀取/etc/passwd,/etc/shadow,/etc/group文件中的某個指定用戶信息
#include <fcntl.h> #include <stdio.h> #include <unistd.h> #include <sys/stat.h> #include <stdio.h> #include <errno.h> #include <string.h> #include <pwd.h> #include <shadow.h> #include <grp.h> int main(int argc, char *argv[]) { char *name = "mysql"; if(argc > 1) { name = argv[1]; } struct passwd *pwd; if((pwd=getpwnam(name)) == NULL) { printf("getpwnam error\r\n"); } printf("name=%s\n",pwd->pw_name); printf("passwd=%s\n",pwd->pw_passwd); printf("uid=%d\n",pwd->pw_uid); printf("gid=%d\n",pwd->pw_gid); printf("gencos=%s\n",pwd->pw_gecos); printf("init dir=%s\n",pwd->pw_dir); printf("init shell=%s\n",pwd->pw_shell); struct spwd *sp; if((sp=getspnam(name)) == NULL) { printf("getspnam error\r\n"); } printf("\nspwd passwod = %s\n",sp->sp_pwdp); struct group *gp; //gp = getgrnam(name); if((gp=getgrgid(pwd->pw_gid)) == NULL) { printf("getgrgid error\r\n"); } printf("\ngroup gr_name = %s\n",gp->gr_name); printf("group passwrd = %s\n",gp->gr_passwd); printf("group gid = %d\n",gp->gr_gid); return 0; }
?
迭代/etc/passwd中的內容(/etc/shadow和/etc/group的迭代方式跟下面類似)
讀取uname相關信息
#include <stdio.h> #include <pwd.h> #include <sys/utsname.h> int main(int argc, char *argv[]) { struct passwd *ptr; setpwent(); while((ptr=getpwent()) != NULL) { printf("%s\n",ptr->pw_name); } endpwent(); struct utsname name; if(uname(&name) < 0) { printf("uname error\r\n"); } printf("sysname = %s\n",name.sysname); printf("nodename = %s\n",name.nodename); printf("release = %s\n",name.release); printf("version = %s\n",name.version); printf("machine = %s\n",name.machine); return 0; }
?
?
時間格式轉換
#include <stdio.h> #include <pwd.h> #include <stdlib.h> #include <time.h> int main(int argc, char *argv[]) { time_t t; struct tm *tmp; char buf1[16]; char buf2[16]; time(&t); tmp = localtime(&t); if(strftime(buf1, 16, "time and date: %r, %a %b %d, %Y",tmp) == 0) { printf("buffer length 16 is too small\n"); } else { printf("%s\n",buf1); } if(strftime(buf2, 64, "time and date: %r, %a %b %d, %Y",tmp) ==0) { printf("buffer length 64 is too small\n"); } else { printf("%s\n",buf2); } return 0; }
?
?
?
?
?
?
?
更多文章、技術交流、商務合作、聯系博主
微信掃碼或搜索:z360901061
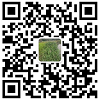
微信掃一掃加我為好友
QQ號聯系: 360901061
您的支持是博主寫作最大的動力,如果您喜歡我的文章,感覺我的文章對您有幫助,請用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點擊下面給點支持吧,站長非常感激您!手機微信長按不能支付解決辦法:請將微信支付二維碼保存到相冊,切換到微信,然后點擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對您有幫助就好】元
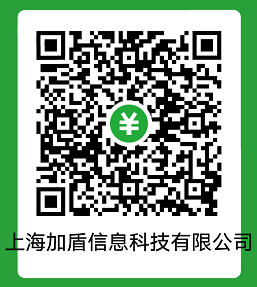