Java IO 編程?
1、基本概念?
?? Java中對(duì)文件的操作是以流的方式進(jìn)行的,流是Java內(nèi)存中一組有序數(shù)據(jù)序列。Java將數(shù)據(jù)從源(文件、內(nèi)存、鍵盤、網(wǎng)絡(luò))讀入到內(nèi)存中,形成了流,然后還可以將這些流寫到另外的目的地(文件、內(nèi)存、控制臺(tái)、網(wǎng)絡(luò))之所以叫做流,是因?yàn)檫@個(gè)數(shù)據(jù)序列在不同時(shí)刻所操作的是源的不同部分。?
2、流的分類?
流的分類方式一般有以下三種:?
(1) 輸入的方向分:輸入流和輸出流,輸入和輸出的參照對(duì)象是Java程序。?
(2) 處理數(shù)據(jù)的單位分:字節(jié)流和字符流,字節(jié)流讀取的最小單位是一個(gè)字節(jié)。?
(3) 功能的不同分:節(jié)點(diǎn)流和處理流,一個(gè)是直接一個(gè)是包裝的。?
3、流分類的關(guān)系?
流分類的根源來(lái)自四個(gè)基本的類,這四個(gè)類的關(guān)系如下:?
?? 字節(jié)流 ?????????? 字符流?
輸入流 InputStream Reader?
輸出流 OutputStream Writer?
4、其他知識(shí)補(bǔ)充?
(1)什么是IO?
?? IO(Input/Output)是計(jì)算機(jī)輸入/輸出的接口,Java的核心庫(kù)java.io提供了全面的IO接口,包括:文件讀寫、標(biāo)準(zhǔn)設(shè)備輸出等等。Java中的IO是以流為基礎(chǔ)進(jìn)行輸入輸出的,所有數(shù)據(jù)被串行化寫入輸出流,或者從輸入流讀入。?
(2)流IO和塊IO?
?? 此外,Java也對(duì)塊傳輸提供支持,在核心庫(kù)java.nio中采用的便是塊IO,流IO和塊IO對(duì)比而言,流IO的好處是簡(jiǎn)單易用,缺點(diǎn)是效率不如塊IO;相反塊IO是效率比較高但是編程比較復(fù)雜。Java的IO模型設(shè)計(jì)非常優(yōu)秀,它使用了Decorator模式,按照功能進(jìn)行劃分stream,編程過(guò)程中可以動(dòng)態(tài)地裝配這些stream,以便獲取所需要的功能。?
?? 備注:以上資料提取自百度文庫(kù),鏈接地址如下:?
???
http://wenku.baidu.com/view/9aa0ec35eefdc8d376ee3280.html
?
5、代碼模擬實(shí)戰(zhàn)?
- import ?java.io.File;??
- import ?java.io.IOException;??
- ??
- public ? class ?FileDemoTest?{??
- ???? /** ?
- ?????*?@description?基礎(chǔ)File類操作 ?
- ?????*?@param?args ?
- ?????*?@throws?IOException?IO異常處理 ?
- ?????*/ ??
- ???? public ? static ? void ?main(String[]?args)? throws ?IOException?{??
- ???????? //?Windows系統(tǒng)下的文件目錄格式,這個(gè)和Unix系統(tǒng)等不同 ??
- ???????? //?String?pathSeparator?=?File.pathSeparator;?//?; ??
- ???????? //?String?separator?=?File.separator;?//?\ ??
- ??
- ???????? //?1、創(chuàng)建和刪除文件 ??
- ????????File?file?=? new ?File( "d:\\helloworld.txt" );??
- ???????? //?File?file?=?new?File("d:"+File.separator+"helloworld.txt"); ??
- ????????file.createNewFile();? //?創(chuàng)建文件 ??
- ???????? if ?(file.exists())?{? //?如果文件存在,則刪除文件 ??
- ????????????file.delete();??
- ????????}??
- ??
- ???????? //?2、創(chuàng)建文件夾操作 ??
- ????????File?file2?=? new ?File( "d:\\helloworld" );??
- ???????? //?File?file2?=?new?File("d:"+File.separator+"helloworld"); ??
- ????????file2.mkdir();? //?建立文件夾 ??
- ???????? if ?(file2.isDirectory())?{??
- ????????????System.out.println( "hello-directory" );? //?判斷是否是目錄 ??
- ????????}??
- ??
- ???????? //?3、遍歷文件或者文件夾操作 ??
- ????????File?file3?=? new ?File( "d:\\" );??
- ???????? //?File?file3?=?new?File("d:"+File.separator); ??
- ????????File?files[]?=?file3.listFiles();? //?列出全部?jī)?nèi)容 ??
- ???????? for ?( int ?i?=? 0 ;?i?<?files.length;?i++)?{??
- ????????????System.out.println(files[i]);??
- ????????}??
- ????}??
- }??
- import ?java.io.File;??
- import ?java.io.IOException;??
- import ?java.io.InputStream;??
- import ?java.io.FileInputStream;??
- ??
- public ? class ?InputStreamDemo?{??
- ???? /** ?
- ?????*?@description?字節(jié)流:輸入流InputStream ?
- ?????*?@param?args ?
- ?????*?@throws?IOException ?
- ?????*/ ??
- ???? public ? static ? void ?main(String[]?args)? throws ?IOException?{??
- ???????? //?字節(jié)流的輸入流和輸出流過(guò)程步驟比較固定 ??
- ???????? //?第1步、使用File類找到一個(gè)文件 ??
- ????????File?f?=? new ?File( "d:" ?+?File.separator?+? "helloworld.txt" );??
- ???????? //?第2步、通過(guò)子類實(shí)例化父類對(duì)象(InputStream為抽象類,本身不能直接實(shí)例化) ??
- ????????InputStream?input?=? new ?FileInputStream(f);??
- ???????? //?第3步、進(jìn)行讀操作 ??
- ???????? byte ?b[]?=? new ? byte [( int )f.length()];? //?數(shù)組大小由文件大小來(lái)確定 ??
- ???????? for ?( int ?i?=? 0 ;?i?<?b.length;?i++)?{??
- ????????????b[i]?=?( byte )?input.read();? //?讀取內(nèi)容 ??
- ????????}??
- ???????? //?第4步、關(guān)閉輸出流 ??
- ????????input.close();???
- ????}??
- }??
- import ?java.io.File;??
- import ?java.io.IOException;??
- import ?java.io.OutputStream;??
- import ?java.io.FileOutputStream;??
- ??
- public ? class ?OutputStreamDemo?{??
- ???? /** ?
- ?????*@description?字節(jié)流:輸出流OutputStream類 ?
- ?????*@param?args ?
- ?????*@throws?IOException? ?
- ?????*/ ??
- ???? public ? static ? void ?main(String[]?args)? throws ?IOException?{??
- ???????? //?輸入和輸出流參考的是Java程序,輸出流操作步驟也比較固定 ??
- ???????? //?第1步、使用File類找到一個(gè)文件 ??
- ????????File?f?=? new ?File( "d:" ?+?File.separator?+? "helloworld.txt" );??
- ???????? //?第2步、通過(guò)子類實(shí)例化父類對(duì)象 ??
- ???????? //OutputStream?out?=?new?FileOutputStream(f); ??
- ????????OutputStream?out?=? new ?FileOutputStream(f, true );??
- ???????? //?第3步、進(jìn)行寫操作 ??
- ????????String?str?=? "say?hello?world!!!" ;??
- ???????? byte ?b[]?=?str.getBytes();??
- ???????? for ( int ?i= 0 ;i<b.length;i++){???
- ????????????out.write(b[i]);? //?單個(gè)寫入 ??
- ????????}??
- ???????? //?out.write(b); ??
- ???????? //?重載方法write ??
- ???????? //?public?abstract?void?write(int?b)?throws?IOException; ??
- ???????? //?public?void?write(byte?b[])?throws?IOException?{} ??
- ???????? //?第4步、關(guān)閉輸出流 ??
- ????????out.close();? //?關(guān)閉輸出流 ??
- ????}??
- }??
- import ?java.io.File;??
- import ?java.io.IOException;??
- import ?java.io.Reader;??
- import ?java.io.FileReader;??
- ??
- public ? class ?ReaderDemo?{??
- ???? /** ?
- ?????*@description?字節(jié)流和字符流按照處理數(shù)據(jù)的單位劃分 ?
- ?????*@param?args ?
- ?????*@throws?IOException?IO異常處理 ?
- ?????*/ ??
- ???? public ? static ? void ?main(String[]?args)? throws ?IOException?{??
- ???????? //?第1步、使用File類找到一個(gè)文件 ??
- ????????File?f?=? new ?File( "d:" ?+?File.separator?+? "helloworld.txt" );??
- ???????? //?第2步、通過(guò)子類實(shí)例化父類對(duì)象 ??
- ????????Reader?input?=? new ?FileReader(f);??
- ???????? //?第3步、進(jìn)行讀操作 ??
- ???????? char ?c[]?=? new ? char [ 1024 ];??
- ???????? int ?temp?=? 0 ;??
- ???????? int ?len?=? 0 ;??
- ???????? while ?((temp?=?input.read())?!=?- 1 )?{??
- ???????????? //?如果不是-1就表示還有內(nèi)容,可以繼續(xù)讀取 ??
- ????????????c[len]?=?( char )?temp;??
- ????????????len++;??
- ????????}??
- ???????? //?第4步、關(guān)閉輸出流 ??
- ????????input.close();??
- ????}??
- }??
- import ?java.io.File;??
- import ?java.io.IOException;??
- import ?java.io.Writer;??
- import ?java.io.FileWriter;??
- ??
- public ? class ?WriterDemo?{??
- ???? /** ?
- ?????*@description?通過(guò)代碼學(xué)習(xí)發(fā)現(xiàn),基本上步驟一致的 ?
- ?????*@param?args ?
- ?????*@throws?IOException ?
- ?????*/ ??
- ???? public ? static ? void ?main(String[]?args)? throws ?IOException?{??
- ???????? //?第1步、使用File類找到一個(gè)文件 ??
- ????????File?f?=? new ?File( "d:" ?+?File.separator?+? "helloworld.txt" );??
- ???????? //?第2步、通過(guò)子類實(shí)例化父類對(duì)象 ??
- ????????Writer?out?=? new ?FileWriter(f);? //?通過(guò)對(duì)象多態(tài)性,進(jìn)行實(shí)例化 ??
- ???????? //?第3步、進(jìn)行寫操作 ??
- ????????String?str?=? "\nsay?hello\tworld" ;???
- ????????out.write(str);???
- ???????? //?第4步、關(guān)閉輸出流 ??
- ???????? //?out.flush();?//?清空緩沖 ??
- ????????out.close();? //?關(guān)閉輸出流 ??
- ??????????
- ???????? //?另外有緩沖功能的類為:BufferedReader ??
- ????}??
- }??
- import ?java.io.ByteArrayInputStream;??
- import ?java.io.File;??
- import ?java.io.FileInputStream;??
- import ?java.io.FileOutputStream;??
- import ?java.io.IOException;??
- import ?java.io.InputStream;??
- import ?java.io.InputStreamReader;??
- import ?java.io.OutputStreamWriter;??
- import ?java.io.Reader;??
- import ?java.io.Writer;??
- ??
- public ? class ?ByteAndCharStreamTest?{??
- ???? /** ?
- ?????*@description??字節(jié)流:InputStream,OutputStream ?
- ?????*??????????????字符流:Reader,Writer?? ?
- ?????*??????????????//?字節(jié)流和字符流相互轉(zhuǎn)換測(cè)試 ?
- ?????*@param?args ?
- ?????*?@throws?IOException?文件操作異常處理 ?
- ?????*/ ??
- ???? public ? static ? void ?main(String[]?args)? throws ?IOException?{??
- ???????? //?1、輸入流-字節(jié)流轉(zhuǎn)換成字符流 ??
- ???????? //?通過(guò)InputStreamReader類來(lái)進(jìn)行轉(zhuǎn)換 ??
- ????????InputStream?is?=? new ?FileInputStream( "d:\\helloworld.txt" );??
- ????????Reader?reader?=? new ?InputStreamReader(is);? //?將字節(jié)流轉(zhuǎn)換成字符流 ??
- ???????? char ?c[]?=? new ? char [ 1024 ];??
- ???????? int ?len?=?reader.read(c);? //?讀取操作,保存在字符數(shù)組中 ??
- ????????reader.close();? //?關(guān)閉操作 ??
- ????????System.out.println( new ?String(c, 0 ,len));? //?字符數(shù)組轉(zhuǎn)換成String類實(shí)例 ??
- ??????????
- ???????? //?2、輸出流-字節(jié)流轉(zhuǎn)換成字符流 ??
- ???????? //?通過(guò)OutputStreamWriter類來(lái)進(jìn)行轉(zhuǎn)換 ??
- ????????File?f?=? new ?File( "d:" ?+?File.separator?+? "helloworld.txt" );??
- ????????Writer?out?=? new ?OutputStreamWriter( new ?FileOutputStream(f));? //?字節(jié)流變?yōu)樽址? ??
- ????????out.write( "hello?world!!!" );? //?使用字符流輸出 ??
- ????????out.close();??
- ??????????
- ???????? //?3、從字符流到字節(jié)流轉(zhuǎn)換可以采用String類提供的操作 ??
- ???????? //?從字符流中獲取char[],轉(zhuǎn)換成String實(shí)例然后再調(diào)用String?API的getBytes()方法? ??
- ???????? //?接1中的代碼如下,最后通過(guò)ByteArrayInputStream即可完成操作 ??
- ????????String?str?=? new ?String(c, 0 ,len);??
- ???????? byte ?b[]?=?str.getBytes();??
- ????????InputStream?is2?=? new ?ByteArrayInputStream(b);??
- ????????is2.close();??
- ??????????
- ???????? //?4、其他常見(jiàn)的流 ??
- ???????? //?內(nèi)存操作流ByteArrayInputStream,ByteArrayOutputStream ??
- ???????? //?管道流PipedOutputStream,PipedInputStream ??
- ???????? //?打印流PrintStream ??
- ???????? //?緩存流BufferedReader ??
- ???????? //?...等等 ??
- ????}??
- }??
- import ?java.io.FileOutputStream;??
- import ?java.io.IOException;??
- import ?java.io.OutputStream;??
- ??
- public ? class ?CharSetDemo?{??
- ???? /** ?
- ?????*?@description?字符編碼學(xué)習(xí)測(cè)試方法 ?
- ?????*?@param?args ?
- ?????*?@throws?IOException?文件IO異常處理 ?
- ?????*/ ??
- ???? public ? static ? void ?main(String[]?args)? throws ?IOException?{??
- ???????? //?1、字符編碼,通過(guò)system類來(lái)獲取 ??
- ????????String?fe?=?System.getProperty( "file.encoding" );??
- ????????System.out.println(fe);? //?GBK ??
- ??????????
- ???????? //?2、進(jìn)行轉(zhuǎn)碼操作 ??
- ????????OutputStream?out?=? new ?FileOutputStream( "d:\\helloworld.txt" );??
- ???????? byte ?b[]?=? "Java,你好!!!" .getBytes( "ISO8859-1" );? //?轉(zhuǎn)碼操作 ??
- ????????out.write(b);? //?保存 ??
- ????????out.close();? //?關(guān)閉 ??
- ????}??
- }??
- import ?java.io.FileInputStream;??
- import ?java.io.FileOutputStream;??
- import ?java.io.InputStream;??
- import ?java.io.ObjectInputStream;??
- import ?java.io.OutputStream;??
- import ?java.io.ObjectOutputStream;??
- import ?java.io.Serializable;??
- ??
- class ?Demo? implements ?Serializable{? //?實(shí)現(xiàn)序列化接口 ??
- ???? //?序列化方式之一,Java自身提供的序列化支持 ??
- ???? //?其他序列化方式,待以后有需要進(jìn)一步學(xué)習(xí) ??
- ???? private ? static ? final ? long ?serialVersionUID?=?1L;??
- ???? private ?String?info;? //?定義私有屬性 ??
- ??????
- ???? //?如果某個(gè)屬性不想被序列化,采用transient來(lái)標(biāo)識(shí) ??
- ???? //private?transient?String?noser;? ??
- ??????
- ???? public ?Demo(String?info){??
- ???????? this .info?=?info;??
- ????}??
- ???? public ?String?getInfo()?{??
- ???????? return ?info;??
- ????}??
- ???? public ? void ?setInfo(String?info)?{??
- ???????? this .info?=?info;??
- ????}??
- ???? public ?String?toString()?{??
- ???????? return ? "info?=?" ?+? this .info;??
- ????}??
- }??
- public ? class ?SerializedDemo?{??
- ???? /** ?
- ?????*@description?對(duì)象序列化、反序列化操作 ?
- ?????*@param?args ?
- ?????*@throws?Exception ?
- ?????*/ ??
- ???? public ? static ? void ?main(String[]?args)? throws ?Exception?{??
- ???????? //?1、對(duì)象序列化是將內(nèi)存中的對(duì)象轉(zhuǎn)換成二進(jìn)制形式的數(shù)據(jù)流 ??
- ???????? //?2、對(duì)象反序列化剛好和對(duì)象序列化方向相反,將二進(jìn)制數(shù)據(jù)流轉(zhuǎn)換成對(duì)象 ??
- ??????????
- ???????? //?a、對(duì)象序列化采用ObjectOutputStream類 ??
- ????????ObjectOutputStream?oos?=? null ;? //?聲明對(duì)象輸出流 ??
- ????????OutputStream?out?=? new ?FileOutputStream( "d:\\helloworld.txt" );???
- ????????oos?=? new ?ObjectOutputStream(out);??
- ????????oos.writeObject( new ?Demo( "helloworld" ));??
- ????????oos.close();??
- ??????????
- ???????? //?b、對(duì)象反序列化采用ObjectInputStream類 ??
- ????????ObjectInputStream?ois?=? null ;? //?聲明對(duì)象輸入流 ??
- ????????InputStream?input?=? new ?FileInputStream( "d:\\helloworld.txt" );??
- ????????ois?=? new ?ObjectInputStream(input);? //?實(shí)例化對(duì)象輸入流 ??
- ????????Object?obj?=?ois.readObject();? //?讀取對(duì)象 ??
- ????????ois.close();??
- ????????System.out.println(obj);??
- ????}??
- }??
二、Java類集合框架?
在百度搜到的一個(gè)比較詳細(xì)的java類集合筆記,鏈接如下:?
http://wenku.baidu.com/view/52cf133f5727a5e9856a6186.html ?
最后,記錄一個(gè)思考題目:采用Java類集合如何實(shí)現(xiàn)一個(gè)stack,使得增加、刪除、獲取最大值、最小值以及中值的時(shí)間操作效率相當(dāng)。?
- /** ?
- ?*?@author?Administrator ?
- ?*? ?
- ?*?@description?如何從java類集框架中選取適當(dāng)?shù)臄?shù)據(jù)結(jié)構(gòu)呢??? ?
- ?*?@history ?
- ?*/ ??
- public ? interface ?Stack<T>?{??
- ???? public ? void ?add(T?t);? //?添加元素 ??
- ???? public ? void ?delete(T?t);? //?刪除元素 ??
- ???? public ?T?getMax();? //?獲取最大值 ??
- ???? public ?T?getMin();? //?獲取最小值 ??
- ???? public ?T?getMiddle();? //?獲取第中間大值 ??
- } ?
更多文章、技術(shù)交流、商務(wù)合作、聯(lián)系博主
微信掃碼或搜索:z360901061
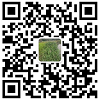
微信掃一掃加我為好友
QQ號(hào)聯(lián)系: 360901061
您的支持是博主寫作最大的動(dòng)力,如果您喜歡我的文章,感覺(jué)我的文章對(duì)您有幫助,請(qǐng)用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點(diǎn)擊下面給點(diǎn)支持吧,站長(zhǎng)非常感激您!手機(jī)微信長(zhǎng)按不能支付解決辦法:請(qǐng)將微信支付二維碼保存到相冊(cè),切換到微信,然后點(diǎn)擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對(duì)您有幫助就好】元
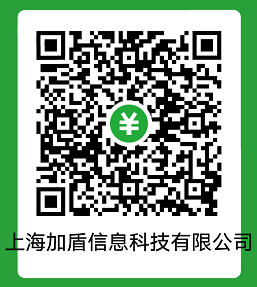