?
Buffer Basics
Attributes
Capacity
The maximum number of data elements the buffer can hold.
The capacity is set when the buffer is created and can never be changed.?
指定緩沖區總容量,在分配緩沖區時指定
Limit
The first element of the buffer that should not be read or written.
In other words, the count of live elements in the buffer.?
指定有多少元素可讀,或者多少空間可寫
Postion
The index of the next element to beread or written.
The position is updated automatically by relative get()and put()methods.?
指定下一個可讀的元素,或者下一個可寫的位置
Mark
A remembered position. Calling mark()sets mark= position.
Calling reset() sets position= mark. The mark is undefined until set.?
標記緩沖區某個位置
?
4個屬性的大小關系比較:
0 <= mark <= position <= limit <= capacity
?
?
API
Super Class
package java.nio; public abstract class Buffer { public final int capacity() public final int position() public final Buffer position (int newPosition) public final int limit() public final Buffer limit (int newLimit) public final Buffer mark() public final Buffer reset() public final Buffer clear() public final Buffer flip() public final Buffer rewind() public final int remaining() public final boolean hasRemaining() public abstract boolean isReadOnly(); }
?
Invocation chaining
buffer.mark().position(5).reset();
?
Filling
buffer.put((byte)'H').put((byte)'e').put((byte)'l').put((byte)'l').put((byte)'o');
?
buffer.put(0, (byte)'M').put((byte)'w');
?
Filpping
buffer.limit(buffer.position()).position(0);
?equal to
buffer.flip();
?
Draining
thread-safe
for (int i = 0; buffer.hasRemaining(), i++) { myByteArray [i] = buffer.get(); }
?
?not thread-safe:
int count = buffer.remaining(); for (int i = 0; i < count, i++) { myByteArray [i] = buffer.get(); }
Buffers are not thread-safe.
If you want to access a given buffer concurrently from multiple threads, you'll need to do your own synchronization.
(e.g., acquiring a lock on the buffer object) prior to accessing the buffer. )
?
Clear
Once a buffer has been filled and drained, it can be reused.
The clear()method resets a buffer to an empty state.
It doesn't change any of the data elements of the buffer but simply sets the limit to the capacity and the position back to 0.
This leaves the buffer ready to be filled again.
?
package com.java.nio; import java.nio.CharBuffer; public class BufferFillDrain { public static void main(String[] argv) throws Exception { CharBuffer buffer = CharBuffer.allocate(100); while (fillBuffer(buffer)) { buffer.flip(); drainBuffer(buffer); buffer.clear(); } } private static void drainBuffer(CharBuffer buffer) { while (buffer.hasRemaining()) { System.out.print(buffer.get()); } System.out.println(""); } private static boolean fillBuffer(CharBuffer buffer) { if (index >= strings.length) { return (false); } String string = strings[index++]; for (int i = 0; i < string.length(); i++) { buffer.put(string.charAt(i));//Not effecient! } return (true); } private static int index = 0; private static String[] strings = { "A random string value", "The product of an infinite number of monkeys", "Hey hey we're the Monkees", "Opening act for the Monkees: Jimi Hendrix", "'Scuse me while I kiss this fly", // Sorry Jimi ;-) "Help Me! Help Me!", }; }?
?
Compating
Occasionally, you may wish to drain some, but not all, of the data from a buffer, then
resume filling it. To do this, the unread data elements need to be shifted down so that the
first element is at index zero. While this could be inefficient if done repeatedly, it's
occasionally necessary, and the API provides a method, compact(), to do it for you. The
buffer implementation can potentially copy the data much more efficiently than you
could by using the get()and put()methods. So if you have a need to do this, use
compact().
?
If you want to drain the buffer contents after compaction, the buffer will need to be
flipped as discussed earlier.
?
?
buffer.compact()?
?
Mark
The clear()method makes a buffer empty,
while reset()returns the position to a previously set mark.
?
?
Bulk Moves
The design goal of buffers is to enable efficient data transfer.
Moving data elements one at a time,? is not very efficient.
As you can see in the following listing, the BufferAPI provides methods to do bulk moves of data elements in or out of a buffer.?
?
public abstract class CharBuffer extends Buffer implements CharSequence, Comparable { // This is a partial API listing public CharBuffer get (char [] dst) public CharBuffer get (char [] dst, int offset, int length) public final CharBuffer put (char[] src) public CharBuffer put (char [] src, int offset, int length) public CharBuffer put (CharBuffer src) public final CharBuffer put (String src) public CharBuffer put (String src, int start, int end) }?
?
?
buffer.get (myArray);?equivalent to
?
?
buffer.get (myArray, 0, myArray.length);Bulk transfers are always of a fixed size. Omitting the length means that the entirearray will be filled.
?
?
?
char [] bigArray = new char [1000]; // Get count of chars remaining in the buffer int length = buffer.remaining(); // Buffer is known to contain < 1,000 chars buffer.get (bigArrray, 0, length); // Do something useful with the data processData (bigArray, length); buffer.put (myArray); //equivalent to buffer.put (myArray, 0, myArray.length); dstBuffer.put (srcBuffer); //equivalent to while (srcBuffer.hasRemaining()) { dstBuffer.put (srcBuffer.get()); } buffer.put (myString); //equivalent to buffer.put (myString, 0, myString.length()); buffer.put (myString, 5, 9); //equivalent to for (int i = start; i < end; i++) } buffer.put (myString.charAt (i)); }?
?
Creating Buffers
New buffers are created by either allocation or wrapping.
Allocation creates a buffer object and allocates private space to hold capacitydata elements. Wrapping creates a buffer object but does not allocate any space to hold the data elements.
It uses the array you provide as backing storage to hold the data elements of the buffer.?
?
CharBuffer charBuffer = CharBuffer.allocate (100); //or //This constructs a new buffer object, but the data elements will live in the array. //Any change to array will reflect to buffer, reverse the same. char [] myArray = new char [100]; CharBuffer charbuffer = CharBuffer.wrap (myArray);
?
Buffers created by either allocate()or wrap()are always nondirect.
Nondirect buffers have backing arrays, and you can gain access to those arrays with the remaining API methods listed above.
The boolean method hasArray() tells you if the buffer has an accessible backing array or
not.
If it returns true, the array() method returns a reference to the array storage used by the buffer object.?
If hasArray()returns false, do not call array()or arrayOffset().
?
Direct Buffers
The most significant way in which byte buffers are distinguished from other buffer types
is that they can be the sources and/or targets of I/O performed by Channels.
?
?
ByteBuffer.allocateDirect()
?
?
ByteBuffer與其它Buffer的最大區別在于,它可以結合Channel進行IO操作
Channel只接收ByteBuffer類型的參數值
?
Direct byte buffers are usually the best choice for I/O operations.
If you will be using the buffer repeatedly in a high-performance scenario, you're better off allocating direct buffers and reusing them.
推薦使用直接緩沖區,并復用它!
?
Direct buffers are optimal for I/O, but they may be more expensive to create than
nondirect byte buffers.
創建直接緩沖區的代價比較高,比在JVM的堆空間創建Buffer更慢。
?
到底使用直接緩沖區還是使用普通緩沖區呢?
First make it work, then make it fast.
?
注意:對于普通緩沖區,Java底層會進行優化處理,效率也是很高的。
?
?
?
?
?
?
更多文章、技術交流、商務合作、聯系博主
微信掃碼或搜索:z360901061
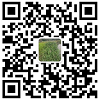
微信掃一掃加我為好友
QQ號聯系: 360901061
您的支持是博主寫作最大的動力,如果您喜歡我的文章,感覺我的文章對您有幫助,請用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點擊下面給點支持吧,站長非常感激您!手機微信長按不能支付解決辦法:請將微信支付二維碼保存到相冊,切換到微信,然后點擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對您有幫助就好】元
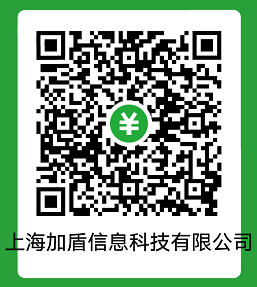