- import ??java.beans.PropertyDescriptor; ??
- import ??java.util.Collection; ??
- ??
- import ??org.apache.commons.beanutils.PropertyUtils; ??
- ??
- ??
- /**? ?
- *?CopyUtil ?
- ?*/ ? ??
- ? public ??? class ??CopyUtil???{ ??
- ??? ??
- ???? /**? ?
- ????*?Copy?properties?of?orig?to?dest ?
- ????*?Exception?the?Entity?and?Collection?Type ?
- ????*??@param??dest ?
- ????*??@param??orig ?
- ????*??@return??the?dest?bean ?
- ?????*/ ? ??
- ????? public ??? static ??Object?copyProperties(Object?dest,?Object?orig)???{ ??
- ???????? if ??(dest??==??? null ???||??orig??==??? null ?)???{ ??
- ???????????? return ??dest; ??
- ???????}? ??
- ??????? ??
- ???????PropertyDescriptor[]?destDesc??=??PropertyUtils.getPropertyDescriptors(dest); ??
- ???????? try ????{ ??
- ???????????? for ??(? int ??i??=??? 0 ?;?i??<??destDesc.length;?i?++?)???{ ??
- ???????????????Class?destType??=??destDesc[i].getPropertyType(); ??
- ???????????????Class?origType??=??PropertyUtils.getPropertyType(orig,?destDesc[i].getName()); ??
- ???????????????? if ?(destType??!=??? null ???&&??destType.equals(origType) ??
- ????????????????????????&&???!?destType.equals(Class.? class ?))???{ ??
- ???????????????????? if ?(?!?Collection.? class ?.isAssignableFrom(origType))??{???????????????????? ??
- ???????????????????????? try ??{ ??
- ???????????????????????????Object?value??=??PropertyUtils.getProperty(orig,?destDesc[i].getName()); ??
- ???????????????????????????PropertyUtils.setProperty(dest,?destDesc[i].getName(),?value); ??
- ???????????????????????}? catch ?(Exception?ex)??{???????????????????????????? ??
- ???????????????????????}? ??
- ???????????????????}? ??
- ???????????????}? ??
- ???????????}? ??
- ??????????? ??
- ???????????? return ??dest; ??
- ???????}? catch ?(Exception?ex)???{ ??
- ???????????? throw ??? new ??CopyException(ex); ??
- //?????????????return?dest;? ??
- ????????}? ??
- ???}????? ??
- ??? ??
- ???? /**? ?
- ????*?Copy?properties?of?orig?to?dest ?
- ????*?Exception?the?Entity?and?Collection?Type ?
- ????*??@param??dest ?
- ????*??@param??orig ?
- ????*??@param??ignores?例如:vo.setUserName?copy?po.setUserName,應(yīng)該寫UserName ?
- ????*??@return??the?dest?bean ?
- ?????*/ ? ??
- ????? public ??? static ??Object?copyProperties(Object?dest,?Object?orig,?String[]?ignores)???{ ??
- ???????? if ??(dest??==??? null ???||??orig??==??? null ?)???{ ??
- ???????????? return ??dest; ??
- ???????}? ??
- ??????? ??
- ???????PropertyDescriptor[]?destDesc??=??PropertyUtils.getPropertyDescriptors(dest); ??
- ???????? try ????{ ??
- ???????????? for ??(? int ??i??=??? 0 ?;?i??<??destDesc.length;?i?++?)???{ ??
- ???????????????? if ??(contains(ignores,?destDesc[i].getName()))???{ ??
- ???????????????????? continue ?; ??
- ???????????????}? ??
- ??????????????? ??
- ???????????????Class?destType??=??destDesc[i].getPropertyType(); ??
- ???????????????Class?origType??=??PropertyUtils.getPropertyType(orig,?destDesc[i].getName()); ??
- ???????????????? if ?(destType??!=??? null ???&&??destType.equals(origType) ??
- ????????????????????????&&???!?destType.equals(Class.? class ?))???{ ??
- ???????????????????? if ?(?!?Collection.? class ?.isAssignableFrom(origType))??{ ??
- ???????????????????????Object?value??=??PropertyUtils.getProperty(orig,?destDesc[i].getName()); ??
- ???????????????????????PropertyUtils.setProperty(dest,?destDesc[i].getName(),?value); ??
- ???????????????????}? ??
- ???????????????}? ??
- ???????????}? ??
- ??????????? ??
- ???????????? return ??dest; ??
- ???????}? catch ?(Exception?ex)???{ ??
- ???????????? throw ??? new ??CopyException(ex); ??
- ???????}? ??
- ???}? ??
- ??? ??
- ???? static ??? boolean ??contains(String[]?ignores,?String?name)???{ ??
- ???????? boolean ??ignored??=??? false ?; ??
- ???????? for ??(? int ??j??=??? 0 ?;?ignores??!=??? null ???&&??j??<??ignores.length;?j?++?)???{ ??
- ???????????? if ??(ignores[j].equals(name))???{ ??
- ???????????????ignored??=??? true ?; ??
- ???????????????? break ?; ??
- ???????????}? ??
- ???????}? ??
- ??????? ??
- ???????? return ??ignored; ??
- ???}? ??
- ???
import java.beans.PropertyDescriptor; import java.util.Collection; import org.apache.commons.beanutils.PropertyUtils; /** * CopyUtil */ public class CopyUtil { /** * Copy properties of orig to dest * Exception the Entity and Collection Type * @param dest * @param orig * @return the dest bean */ public static Object copyProperties(Object dest, Object orig) { if (dest == null || orig == null ) { return dest; } PropertyDescriptor[] destDesc = PropertyUtils.getPropertyDescriptors(dest); try { for ( int i = 0 ; i < destDesc.length; i ++ ) { Class destType = destDesc[i].getPropertyType(); Class origType = PropertyUtils.getPropertyType(orig, destDesc[i].getName()); if (destType != null && destType.equals(origType) && ! destType.equals(Class. class )) { if ( ! Collection. class .isAssignableFrom(origType)) { try { Object value = PropertyUtils.getProperty(orig, destDesc[i].getName()); PropertyUtils.setProperty(dest, destDesc[i].getName(), value); } catch (Exception ex) { } } } } return dest; } catch (Exception ex) { throw new CopyException(ex); // return dest; } } /** * Copy properties of orig to dest * Exception the Entity and Collection Type * @param dest * @param orig * @param ignores 例如:vo.setUserName copy po.setUserName,應(yīng)該寫UserName * @return the dest bean */ public static Object copyProperties(Object dest, Object orig, String[] ignores) { if (dest == null || orig == null ) { return dest; } PropertyDescriptor[] destDesc = PropertyUtils.getPropertyDescriptors(dest); try { for ( int i = 0 ; i < destDesc.length; i ++ ) { if (contains(ignores, destDesc[i].getName())) { continue ; } Class destType = destDesc[i].getPropertyType(); Class origType = PropertyUtils.getPropertyType(orig, destDesc[i].getName()); if (destType != null && destType.equals(origType) && ! destType.equals(Class. class )) { if ( ! Collection. class .isAssignableFrom(origType)) { Object value = PropertyUtils.getProperty(orig, destDesc[i].getName()); PropertyUtils.setProperty(dest, destDesc[i].getName(), value); } } } return dest; } catch (Exception ex) { throw new CopyException(ex); } } static boolean contains(String[] ignores, String name) { boolean ignored = false ; for ( int j = 0 ; ignores != null && j < ignores.length; j ++ ) { if (ignores[j].equals(name)) { ignored = true ; break ; } } return ignored; } }
- public ? class ?PO2VO? extends ?TestCase?{ ??
- ??
- ???? /*?(non-Javadoc) ?
- ?????*?@see?junit.framework.TestCase#setUp() ?
- ?????*/ ??
- ???? protected ? void ?setUp()? throws ?Exception?{ ??
- ???????? super .setUp(); ??
- ????} ??
- ???? ??
- ???? public ? void ?testPO2VO(){ ??
- ????????TUserBasicVO?vo?=? new ?TUserBasicVO(); ??
- ????????TUserBasic?po?=? new ?TUserBasic(); ??
- ????????po.setPwd( "111" ); ??
- ????????po.setUserName( "222" ); ??
- ????????String[]?a={ "Pwd" , "UserName" }; ??
- ???????? try ?{ ??
- ????????????CopyUtil.copyProperties(vo,?po,a); ??
- ????????}? catch ?(Exception?e)?{ ??
- ???????????? //?TODO?Auto-generated?catch?block ??
- ????????????e.printStackTrace(); ??
- ????????} ??
- ????????System.out.println(vo.getPwd()); ??
- ????} ??
- }??
更多文章、技術(shù)交流、商務(wù)合作、聯(lián)系博主
微信掃碼或搜索:z360901061
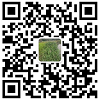
微信掃一掃加我為好友
QQ號聯(lián)系: 360901061
您的支持是博主寫作最大的動力,如果您喜歡我的文章,感覺我的文章對您有幫助,請用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點擊下面給點支持吧,站長非常感激您!手機微信長按不能支付解決辦法:請將微信支付二維碼保存到相冊,切換到微信,然后點擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對您有幫助就好】元
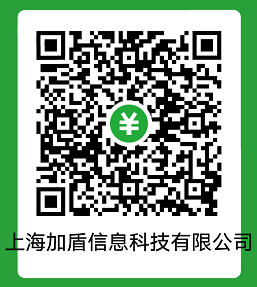