最近自己在做一個小系統玩的時候涉及到了文件的上傳,于是在網上找到Java上傳文件的方案,最后確定使用common-fileupload實現上傳操作。
?
- 需求說明
用戶添加頁面有一個“上傳”按鈕,點擊按鈕彈出上傳界面,上傳完成后關閉上傳界面。
?
- 所需Jar包
commons.fileupload-1.2.0.jar、commons.logging-1.1.1.jar、commons.beanutils-1.8.0.jar、commons.collections-3.2.0.jar、commons.io-1.4.0.jar、commons.lang-2.1.0.jar
?
- 實現效果
?
?
- ?代碼實現
?
/** * 跳轉到上傳頁 * functionId:功能ID * fileType:文件類型 * maxSize:文件容量上限 * callback:回調函數,返回三個參數:文件真名、文件存放名和文件大小 */ function openUpload(functionId,fileType,maxSize,callback){ var url = root+"/CommonController.jhtml?method=goFileUpload&"; if(functionId!=null){ url = url + "functionId="+functionId+"&"; } if(fileType!=null){ url = url + "fileType="+fileType+"&"; } if(maxSize!=null){ url = url + "maxSize="+maxSize; } var win = window.showModalDialog(url,"","dialogWidth:300px;dialogHeight:150px;scroll:no;status:no"); if(win != null){ var arrWin = win.split(","); callback(arrWin[0],arrWin[1],arrWin[2]); } }
? 用戶添加頁面相關代碼,點擊“上傳”按鈕時調用上面的核心js代碼,并且獲取返回值
?
<script> ....... function openUpload_(){ openUpload(null,'JPG,GIF,JPEG,PNG','5',callback); } /** * 回調函數,獲取上傳文件信息 * realName真實文件名 * saveName文件保存名 * maxSize文件實際大小 */ function callback(realName,saveName,maxSize){ $("#photo_").val(saveName); //回調后其它操作 } </script> <tr> <td>頭像:</td> <td> <input type="hidden" name="photo" id="photo_"></input> <input type="button" onclick="openUpload_()" value="上傳"/> </td> </tr>
? 文件上傳 的JSP代碼,需要注意的是在head標簽內添加<base target="_self">以防止頁面跳轉時彈出新窗口,用戶選擇指定文件,點擊上傳時就提交表單訪問指定后臺代碼
?
<%@ include file="/WEB-INF/jsp/header.jsp" %> <%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1"> <meta http-equiv="pragma" content="no-cache" /> <base target="_self"> <title> 文件上傳 </title> </head> <body> <h5> 文件上傳 </h5><hr/> <form id="file_upload_id" name="file_upload_name" action="<%=root%>/CommonController.jhtml?method=doFileUpload" method="post" enctype="multipart/form-data"> <input type="hidden" name="functionId" value="${functionId}"/> <input type="hidden" name="fileType" value="${fileType}"/> <input type="hidden" name="maxSize" value="${maxSize}"/> <div><input type="file" name="file_upload"/></div> <c:if test="${maxSize!=null}"> <div style="font: 12">文件最大不能超過${maxSize}MB</div> </c:if> <c:if test="${fileType!=null}"> <div style="font: 12">文件格式必須是:${fileType}</div> </c:if> <div><input type="submit" value="上傳"/></div> </form> </body> </html>
? CommonController目前有兩個方法,一個是跳轉到上傳頁面的方法,一個是執行上傳操作的方法 doFileUpload,上傳方法運行的大概邏輯是:首先獲取頁面的請求參數,fileType用于限制上傳文件格式,
maxSize用于限制上傳文件最大值,隨后創建上傳目錄上傳即可。
?
public class CommonController extends BaseController { Log log = LogFactory.getLog(CommonController.class); Properties fileUploadPro = null; public CommonController(){ fileUploadPro = PropertiesUtil.getPropertiesByClass("fileupload.properties"); } @Override public ModeAndView init(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { return null; } /** * 跳轉到 文件上傳 頁 * @param request * @param response * @return * @throws ServletException * @throws IOException */ public ModeAndView goFileUpload(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { String functionId = request.getParameter("functionId"); String fileType = request.getParameter("fileType"); String maxSize = request.getParameter("maxSize"); ModeAndView mav = new ModeAndView("/WEB-INF/jsp/common/fileUpload.jsp"); if(functionId!=null && !"".equals(functionId.trim())){ mav.addObject("functionId", functionId); } if(fileType!=null && !"".equals(fileType.trim())){ mav.addObject("fileType", fileType); } if(maxSize!=null && !"".equals(maxSize.trim())){ mav.addObject("maxSize", maxSize); } return mav; } /** * 上傳文件 * @param request * @param response * @return * @throws ServletException * @throws IOException */ @SuppressWarnings("unchecked") public ModeAndView doFileUpload(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { //獲取并解析文件類型和支持最大值 String functionId = request.getParameter("functionId"); String fileType = request.getParameter("fileType"); String maxSize = request.getParameter("maxSize"); //臨時目錄名 String tempPath = fileUploadPro.getProperty("tempPath"); //真實目錄名 String filePath = fileUploadPro.getProperty("filePath"); FileUtil.createFolder(tempPath); FileUtil.createFolder(filePath); DiskFileItemFactory factory = new DiskFileItemFactory(); //最大緩存 factory.setSizeThreshold(5*1024); //設置臨時文件目錄 factory.setRepository(new File(tempPath)); ServletFileUpload upload = new ServletFileUpload(factory); if(maxSize!=null && !"".equals(maxSize.trim())){ //文件最大上限 upload.setSizeMax(Integer.valueOf(maxSize)*1024*1024); } try { //獲取所有文件列表 List<FileItem> items = upload.parseRequest(request); for (FileItem item : items) { if(!item.isFormField()){ //文件名 String fileName = item.getName(); //檢查文件后綴格式 String fileEnd = fileName.substring(fileName.lastIndexOf(".")+1).toLowerCase(); if(fileType!=null && !"".equals(fileType.trim())){ boolean isRealType = false; String[] arrType = fileType.split(","); for (String str : arrType) { if(fileEnd.equals(str.toLowerCase())){ isRealType = true; break; } } if(!isRealType){ //提示錯誤信息:文件格式不正確 super.printJsMsgBack(response, "文件格式不正確!"); return null; } } //創建文件唯一名稱 String uuid = UUID.randomUUID().toString(); //真實上傳路徑 StringBuffer sbRealPath = new StringBuffer(); sbRealPath.append(filePath).append(uuid).append(".").append(fileEnd); //寫入文件 File file = new File(sbRealPath.toString()); item.write(file); //上傳成功,向父窗體返回數據:真實文件名,虛擬文件名,文件大小 StringBuffer sb = new StringBuffer(); sb.append("window.returnValue='").append(fileName).append(",").append(uuid).append(".").append(fileEnd).append(",").append(file.length()).append("';"); sb.append("window.close();"); super.printJsMsg(response, sb.toString()); log.info("上傳文件成功,JS信息:"+sb.toString()); }//end of if }//end of for }catch (Exception e) { //提示錯誤:比如文件大小 super.printJsMsgBack(response, "上傳失敗,文件大小不能超過"+maxSize+"M!"); log.error("上傳文件異常!",e); return null; } return null; } }
? 至此一個 文件上傳 即已實現,而且能夠基本滿足不同模塊的上傳通用性,我還留著個functionId參數用于以后針對不同模塊上傳文件到不同目錄。
更多文章、技術交流、商務合作、聯系博主
微信掃碼或搜索:z360901061
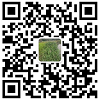
微信掃一掃加我為好友
QQ號聯系: 360901061
您的支持是博主寫作最大的動力,如果您喜歡我的文章,感覺我的文章對您有幫助,請用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點擊下面給點支持吧,站長非常感激您!手機微信長按不能支付解決辦法:請將微信支付二維碼保存到相冊,切換到微信,然后點擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對您有幫助就好】元
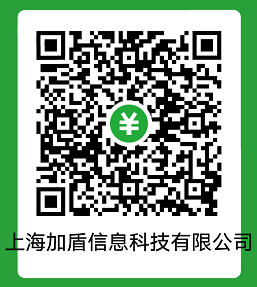