一、Convention的Annotation
1) 與Action相關的兩個Annotation是@Action 和@Actions
2) @Action中可指定一個value屬性。類似于指定<action name=””/>屬性值
3) @Action中還可以指定一個params屬性,該屬性是一個字符串數組,用于該Acion指定的參數名和參數值。params屬性應遵守如下格式:{“name1”,”value1”,”name2”,”value2”}
4) @Actions 也用于修飾Action類里的方法,用于將該方法映射到多個URL.@Actions用于組織多個@Action.因此它可將一個方法映射成多個邏輯Action。
通過@Action注釋
對如下例子:
- package com.example.web;
- import com.opensymphony.xwork2.Action;
- import com.opensymphony.xwork2.ActionSupport;
- public class HelloAction extends ActionSupport{
- @Action ( "action1" )
- public Stringmethod1(){
- return SUCCESS;
- }
- @Action ( "/user/action2" )
- public Stringmethod2(){
- return SUCCESS;
- }
- }
方法名 | 默認調用路徑 | 默認映射路徑 |
method1 | /hello!method1.action . | /WEB-INF/content/hello.jsp |
method2 | /hello!method2.action. | /WEB-INF/content/hello.jsp |
通過@Action注釋后
方法名 | @Action注釋后調用路徑 | @Action注釋后映射路徑 |
method1 | /action1!method1.action. 或簡寫 /action1 | /WEB-INF/content/action1.jsp |
method1 | /user/action2!method2.action或 /user/action2 | /WEB-INF/content/user/action2.jsp |
注:
@Action(
"/user"
)則是
/user,對應的映射路徑是
/WEB-INF/content/action3.jsp,
該注釋覆蓋了默認的namespace(“/”)
通過@Actions注釋
- package com.example.web;
- import com.opensymphony.xwork2.ActionSupport;
- import org.apache.struts2.convention.annotation.Action;
- import org.apache.struts2.convention.annotation.Actions;
- public class HelloAction extends ActionSupport{
- @Actions ({
- @Action ( "/different/url" ),
- @Action ( "/another/url" )
- })
- public Stringmethod1(){
- return “error”;
- }
我們可以通過:
/different/url!method1.action(
/different/url
)
或
/another/url!method1.action
來調用
method1
方法。
對應的映射路徑分別是
/WEB-INF/content/different/url-error.jsp; /WEB-INF/content/another/url-error.jsp
可能誤導了大家,一個方法被@Action注釋后,只是多了一種調用方式,而不是說覆蓋了原來的調用方式。比如對于如下例子:
- package com.example.web;
- import com.opensymphony.xwork2.ActionSupport;
- import org.apache.struts2.convention.annotation.Action;
- import org.apache.struts2.convention.annotation.Actions;
- public class HelloAction extends ActionSupport{
- @Action ( "/another/url" )
- public Stringmethod1(){
- return “error”;
- }
我們調用method1方法可以通過兩種方式:
1
/hello!method1.action
映射
url:
/WEB-INF/content/hello-error.jsp
2
/another/url!method1.action
映射
url:
/WEB-INF/content/another/url-error.jsp
可見,兩種方式均可對method1方法進行調用,唯一的區別就是,兩種調用的映射是不一樣的,所以,想跳轉到不同的界面,這是一個非常好的選擇。
通過@Namespace 注釋
- package com.example.web;
- import com.opensymphony.xwork2.ActionSupport;
- import org.apache.struts2.convention.annotation.Action;
- import org.apache.struts2.convention.annotation.Actions;
- @Namespace ( "/other" )
- public class HelloWorld extends ActionSupport{
- public Stringmethod1(){
- return “error”;
- }
- @Action ( "url" )
- public Stringmethod2(){
- return “error”;
- }
- @Action ( "/different/url" )
- public Stringmethod3(){
- return “error”;
-
}
- }
通過
/other/hello-world!method1.action
訪問
method1
方法。
通過
/other/url!method2.action
訪問
method2
方法
映射
url:
/WEB-INF/content/
/other/
url/
hello-error.jsp
通過
/different /url!method3.action
訪問
method3
方法
與@Action 注釋不同的是,該注釋覆蓋了默認的namespace(這里是’/’),此時再用
hello!method1.action
已經不能訪問
method1
了.
@Results和@Result
1 全局的(global)。
全局results可以被action類中所有的action分享,這種results在action類上使用注解進行聲明。
- package com.example.actions;
- import com.opensymphony.xwork2.ActionSupport;
- import org.apache.struts2.convention.annotation.Action;
- import org.apache.struts2.convention.annotation.Actions;
- import org.apache.struts2.convention.annotation.Result;
- import org.apache.struts2.convention.annotation.Results;
- @Results ({
- @Result (name= "failure" ,location= "/WEB-INF/fail.jsp" )
- })
- public class HelloWorld extends ActionSupport{
- public Stringmethod1(){
- return “failure”;
- }
- @Action ( "/different/url" )
- public Stringmethod2(){
- return “failure”;
- }
- }
當我們訪問
/hello
-world
!method1.action
時,返回
/WEB-INF/fail.jsp
當我們訪問
/hello
-world
!method2.action
時,返回
/WEB-INF/fail.jsp
當我們訪問
/different/url!method2.action
時,返回
/WEB-INF/fail.jsp
2 本地的(local)。
本地results只能在action方法上進行聲明。
- package com.example.actions;
- import com.opensymphony.xwork2.ActionSupport;
- import org.apache.struts2.convention.annotation.Action;
- import org.apache.struts2.convention.annotation.Actions;
- import org.apache.struts2.convention.annotation.Result;
- import org.apache.struts2.convention.annotation.Results;
- public class HelloWorld extends ActionSupport{
- @Action (value= "/other/bar" ,results={ @Result (name= "error" ,location= "www.baidu.com" ,type= "redirect" )})
- public Stringmethod1(){
- return “error”;
- }
- }
當我們調用
/hello
-world
!method1.action
時,返回
/WEB-INF/content/hello-error.jsp
當我們調用
/other/bar!method1.action
時,返回
www.baidu.com
更多文章、技術交流、商務合作、聯系博主
微信掃碼或搜索:z360901061
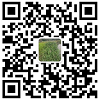
微信掃一掃加我為好友
QQ號聯系: 360901061
您的支持是博主寫作最大的動力,如果您喜歡我的文章,感覺我的文章對您有幫助,請用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點擊下面給點支持吧,站長非常感激您!手機微信長按不能支付解決辦法:請將微信支付二維碼保存到相冊,切換到微信,然后點擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對您有幫助就好】元
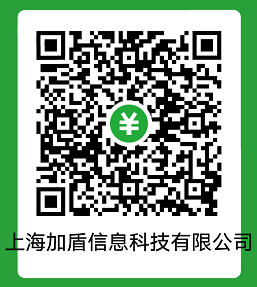