使用ArrayAdapter定制 To-Do List
?
這個(gè)例子將擴(kuò)展 To-Do List 工程,以一個(gè) ToDoItem 對(duì)象來(lái)儲(chǔ)存每一個(gè)項(xiàng)目,包含每個(gè)項(xiàng)目的創(chuàng)建日期。
?
你將擴(kuò)展 ArrayAdapter 類來(lái)綁定一組 ToDoItem 對(duì)象到 ListView 上,并定制用于顯示每一個(gè) ListView 項(xiàng)目的 layout 。
?
1. 返回到 To-Do List 工程。創(chuàng)建一個(gè)新的 ToDoItem 類來(lái)保存任務(wù)和任務(wù)的創(chuàng)建日期。重寫 toString 方法來(lái)返回一個(gè)項(xiàng)目數(shù)據(jù)的概要。
?
- package ?com.paad.todolist;??
- import ?java.text.SimpleDateFormat;??
- import ?java.util.Date;??
- ???
- public ? class ?ToDoItem?{??
- String?task;??
- Date?created;??
- ???
- public ?String?getTask()?{??
- return ?task;??
- }??
- ???
- public ?Date?getCreated()?{??
- return ?created;??
- }??
- ???
- public ?ToDoItem(String?_task)?{??
- this (_task,? new ?Date(java.lang.System.currentTimeMillis()));??
- }??
- ???
- public ?ToDoItem(String?_task,?Date?_created)?{??
- task?=?_task;??
- created?=?_created;??
- }??
- ???
- @Override ??
- public ?String?toString()?{??
- SimpleDateFormat?sdf?=? new ?SimpleDateFormat(“dd/MM/yy”);??
- String?dateString?=?sdf.format(created);??
- return ?“(“?+?dateString?+?“)?“?+?task;??
- }??
- }??
?
2. 打開 ToDoList Activity ,修改 ArrayList 和 ArrayAdapter 變量的類型,儲(chǔ)存 ToDoItem 對(duì)象而不是字符串。然后,你將修改 onCreate 方法來(lái)更新相應(yīng)的變量初始化。你還需要更新 onKeyListener 處理函數(shù)來(lái)支持 ToDoItem 對(duì)象。
?
- private ?ArrayList<ToDoItem>?todoItems;??
- private ?ListView?myListView;??
- private ?EditText?myEditText;??
- private ?ArrayAdapter<ToDoItem>?aa;??
- ???
- @Override ??
- public ? void ?onCreate(Bundle?icicle)?{??
- super .onCreate(icicle);??
- ???
- //?Inflate?your?view ??
- setContentView(R.layout.main);??
- ???
- //?Get?references?to?UI?widgets ??
- myListView?=?(ListView)findViewById(R.id.myListView);??
- myEditText?=?(EditText)findViewById(R.id.myEditText);??
- todoItems?=? new ?ArrayList<ToDoItem>();??
- int ?resID?=?R.layout.todolist_item;??
- aa?=? new ?ArrayAdapter<ToDoItem>( this ,?resID,?todoItems);??
- myListView.setAdapter(aa);??
- myEditText.setOnKeyListener( new ?OnKeyListener()?{??
- public ? boolean ?onKey(View?v,? int ?keyCode,?KeyEvent?event)??
- {??
- if ?(event.getAction()?==?KeyEvent.ACTION_DOWN)??
- if ?(keyCode?==?KeyEvent.KEYCODE_DPAD_CENTER)??
- {??
- ToDoItem?newItem;??
- newItem?=? new ?ToDoItem(myEditText.getText().toString());??
- todoItems.add( 0 ,?newItem);??
- myEditText.setText(“”);??
- aa.notifyDataSetChanged();??
- cancelAdd();??
- return ? true ;??
- }??
- return ? false ;??
- }??
- });??
- registerForContextMenu(myListView);??
- }??
?
3. 如果你運(yùn)行 Activity ,它將顯示每個(gè) to-do 項(xiàng)目,如圖 5-3 所示。
?
圖 5-3
?
4. 現(xiàn)在,你可以創(chuàng)建一個(gè)自定義的 layout 來(lái)顯示每一個(gè) to-do 項(xiàng)目。修改在第 4 章中創(chuàng)建的自定義 layout ,包含另外一個(gè) TextView ,它將用于顯示每個(gè) to-do 項(xiàng)目的創(chuàng)建日期。
?
- <? xml ? version =”1.0”? encoding =”utf-8” ?> ??
- < RelativeLayout ? xmlns:android =”http://schemas.android.com/apk/res/android”??
- android:layout_width =”fill_parent”??
- android:layout_height =”fill_parent”??
- android:background =”@color/notepad_paper” > ??
- < TextView ??
- android:id =”@+id/rowDate”??
- android:layout_width =”wrap_content”??
- android:layout_height =”fill_parent”??
- android:padding =”10dp”??
- android:scrollbars =”vertical”??
- android:fadingEdge =”vertical”??
- android:textColor =”@color/notepad_text”??
- android:layout_alignParentRight =”true”??
- /> ??
- < TextView ??
- android:id =”@+id/row”??
- android:layout_width =”fill_parent”??
- android:layout_height =”fill_parent”??
- android:padding =”10dp”??
- android:scrollbars =”vertical”??
- android:fadingEdge =”vertical”??
- android:textColor =”@color/notepad_text”??
- android:layout_alignParentLeft =”@+id/rowDate”??
- /> ??
- </ RelativeLayout > ??
?
5. 創(chuàng)建一個(gè)新的類( ToDoItemAdapter ),使用指定的 ToDoItem 變量來(lái)擴(kuò)展一個(gè) ArrayAdapter 。重寫 getView 方法來(lái)將 ToDoItem 對(duì)象中的 task 和 date 屬性指定給第 4 步創(chuàng)建的 layout 中的 View 。
- ???
- import ?java.text.SimpleDateFormat;??
- import ?android.content.Context;??
- import ?java.util.*;??
- import ?android.view.*;??
- import ?android.widget.*;??
- ???
- public ? class ?ToDoItemAdapter? extends ?ArrayAdapter<ToDoItem>?{??
- int ?resource;??
- ???
- public ?ToDoItemAdapter(Context?_context, int ?_resource,?List<ToDoItem>?_items)?{??
- super (_context,?_resource,?_items);??
- resource?=?_resource;??
- }??
- ???
- @Override ??
- public ?View?getView( int ?position,?View?convertView,?ViewGroup?parent)??
- {??
- LinearLayout?todoView;??
- ToDoItem?item?=?getItem(position);??
- String?taskString?=?item.getTask();??
- Date?createdDate?=?item.getCreated();??
- SimpleDateFormat?sdf?=? new ?SimpleDateFormat(“dd/MM/yy”);??
- String?dateString?=?sdf.format(createdDate);??
- if ?(convertView?==? null )??
- {??
- todoView?=? new ?LinearLayout(getContext());??
- String?inflater?=?Context.LAYOUT_INFLATER_SERVICE;??
- LayoutInflater?vi;??
- vi?=?(LayoutInflater)getContext().getSystemService(inflater);??
- vi.inflate(resource,?todoView,? true );??
- }??
- else ??
- {??
- todoView?=?(LinearLayout)?convertView;??
- }??
- TextView?dateView?=?(TextView)todoView.findViewById(R.id.rowDate);??
- TextView?taskView?=?(TextView)todoView.findViewById(R.id.row);??
- dateView.setText(dateString);??
- taskView.setText(taskString);??
- return ?todoView;??
- }??
- }??
?
6. 最后,用 ToDoItemAdapter 替換 ArrayAdapter 的定義。
?
private ToDoItemAdapter aa;
?
在 onCreate 中,使用 new ToDoItemAdapter 來(lái)替換 ArrayAdapter<String> 的實(shí)例化。
?
aa = new ToDoItemAdapter(this, resID, todoItems);
?
7. 如果你運(yùn)行 Activity ,它看起來(lái)如圖 5-4 的截圖。
?
圖 5-4
?
使用SimpleCursorAdapter
?
SimpleCursorAdapter 允許你綁定一個(gè)游標(biāo)的列到 ListView 上,并使用自定義的 layout 顯示每個(gè)項(xiàng)目。
?
SimpleCursorAdapter 的創(chuàng)建,需要傳入當(dāng)前的上下文、一個(gè) layout 資源,一個(gè)游標(biāo)和兩個(gè)數(shù)組:一個(gè)包含使用的列的名字,另一個(gè)(相同大?。?shù)組包含 View 中的資源 ID ,用于顯示相應(yīng)列的數(shù)據(jù)值。
?
下面的框架代碼顯示了如何構(gòu)造一個(gè) SimpleCursorAdapter 來(lái)顯示聯(lián)系人信息:
?
- String?uriString?=?“content: //contacts/people/”; ??
- Cursor?myCursor?=?managedQuery(Uri.parse(uriString),? null ,? null ,? null ,? null );??
- String[]?fromColumns?=? new ?String[]?{People.NUMBER,?People.NAME};??
- int []?toLayoutIDs?=? new ? int []?{?R.id.nameTextView,?R.id.numberTextView};??
- SimpleCursorAdapter?myAdapter;??
- myAdapter?=? new ?SimpleCursorAdapter(
- 2011-01-07 18:47
- 瀏覽 517
- 評(píng)論(0)
- 分類: 移動(dòng)開發(fā)
- 相關(guān)推薦
發(fā)表評(píng)論
- 瀏覽: 647346 次
-
性別:
- 來(lái)自: 北京
-
最新評(píng)論
-
龍寶寶吱吱
: 我現(xiàn)在知道是在部署的項(xiàng)目里找,可是找不到啊,一般這個(gè)文件會(huì)在什 ...
webservice瀏覽器遠(yuǎn)程調(diào)用測(cè)試 -
龍寶寶吱吱
: 這個(gè)webconfig文件在哪里啊,大神
webservice瀏覽器遠(yuǎn)程調(diào)用測(cè)試 -
yuer1218
: 正在學(xué)習(xí)這塊,能把源碼發(fā)我一份嗎,12045464@qq.co ...
Android時(shí)鐘的widget【安卓進(jìn)化三十七】 -
hongshanguo
: 為嘛沒有任務(wù)管理器的,求案例啊??
近百android程序源碼貢獻(xiàn) -
難得糊涂CN2010
: 最近一個(gè)項(xiàng)目要用到widget,可以送下源碼不?dz.bita ...
Android時(shí)鐘的widget【安卓進(jìn)化三十七】
更多文章、技術(shù)交流、商務(wù)合作、聯(lián)系博主
微信掃碼或搜索:z360901061
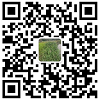
微信掃一掃加我為好友
QQ號(hào)聯(lián)系: 360901061
您的支持是博主寫作最大的動(dòng)力,如果您喜歡我的文章,感覺我的文章對(duì)您有幫助,請(qǐng)用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點(diǎn)擊下面給點(diǎn)支持吧,站長(zhǎng)非常感激您!手機(jī)微信長(zhǎng)按不能支付解決辦法:請(qǐng)將微信支付二維碼保存到相冊(cè),切換到微信,然后點(diǎn)擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對(duì)您有幫助就好】元
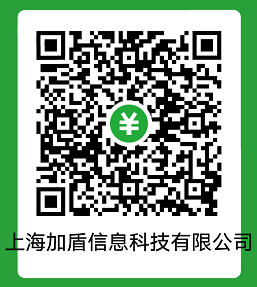
評(píng)論