如果你現(xiàn)在正使用iphone、android以及Web等多種平臺工作,請看一下這篇文章,它會(huì)告訴你如何使用PHP創(chuàng)建RESTful API。Representational state transfer (REST) 是一個(gè)用于向不同應(yīng)用分發(fā)數(shù)據(jù)的軟件系統(tǒng)。Web服務(wù)系統(tǒng)會(huì)以JSON或者XML方式響應(yīng)狀態(tài)碼。
REST API處理流程
數(shù)據(jù)庫
數(shù)據(jù)庫表users包含了user_id, user_fullname, user_email, user_password 和 user_status字段,十分簡單。
1
|
CREATE
TABLE
IF
NOT
EXISTS `users`
|
2
|
(
|
3
|
`user_id`
int
(11)
NOT
NULL
AUTO_INCREMENT,
|
4
|
`user_fullname`
varchar
(25)
NOT
NULL
,
|
5
|
`user_email`
varchar
(50)
NOT
NULL
,
|
6
|
`user_password`
varchar
(50)
NOT
NULL
,
|
7
|
`user_status` tinyint(1)
NOT
NULL
DEFAULT
'0'
,
|
8
|
PRIMARY
KEY
(`user_id`)
|
9
|
) ENGINE=InnoDB
DEFAULT
CHARSET=latin1 AUTO_INCREMENT=1 ;
|
Rest API類:api.php
代碼十分簡單,你需要修改數(shù)據(jù)庫配置信息,如數(shù)據(jù)庫名、數(shù)據(jù)庫賬戶以及密碼。
1
|
require_once
(
"Rest.inc.php"
);
|
2
|
3
|
class
API
extends
REST
|
4
|
{
|
5
|
public
$data
=
""
;
|
6
|
const
DB_SERVER =
"localhost"
;
|
7
|
const
DB_USER =
"Database_Username"
;
|
8
|
const
DB_PASSWORD =
"Database_Password"
;
|
9
|
const
DB =
"Database_Name"
;
|
10
|
11
|
private
$db
= NULL;
|
12
|
13
|
public
function
__construct()
|
14
|
{
|
15
|
parent::__construct();
// Init parent contructor
|
16
|
$this
->dbConnect();
// Initiate Database connection
|
17
|
}
|
18
|
19
|
//Database connection
|
20
|
private
function
dbConnect()
|
21
|
{
|
22
|
$this
->db = mysql_connect(self::DB_SERVER,self::DB_USER,self::DB_PASSWORD);
|
23
|
if
(
$this
->db)
|
24
|
mysql_select_db(self::DB,
$this
->db);
|
25
|
}
|
26
|
27
|
//Public method for access api.
|
28
|
//This method dynmically call the method based on the query string
|
29
|
public
function
processApi()
|
30
|
{
|
31
|
$func
=
strtolower
(trim(
str_replace
(
"/"
,
""
,
$_REQUEST
[
'rquest'
])));
|
32
|
if
((int)method_exists(
$this
,
$func
) > 0)
|
33
|
$this
->
$func
();
|
34
|
else
|
35
|
$this
->response(
''
,404);
|
36
|
// If the method not exist with in this class, response would be "Page not found".
|
37
|
}
|
38
|
39
|
private
function
login()
|
40
|
{
|
41
|
..............
|
42
|
}
|
43
|
44
|
private
function
users()
|
45
|
{
|
46
|
..............
|
47
|
}
|
48
|
49
|
private
function
deleteUser()
|
50
|
{
|
51
|
.............
|
52
|
}
|
53
|
54
|
//Encode array into JSON
|
55
|
private
function
json(
$data
)
|
56
|
{
|
57
|
if
(
is_array
(
$data
)){
|
58
|
return
json_encode(
$data
);
|
59
|
}
|
60
|
}
|
61
|
}
|
62
|
63
|
// Initiiate Library
|
64
|
$api
=
new
API;
|
65
|
$api
->processApi();
|
提交登陸
通過訪問REST API地址http://localhost/rest/login/ 顯示從users表中查詢出的用戶數(shù)據(jù)。Restful API 的登錄狀態(tài)是根據(jù)狀態(tài)碼工作的。如果狀態(tài)碼為200,則登陸成功;否則狀態(tài)碼為204,會(huì)顯示失敗信息。更多的狀態(tài)碼信息請查看示例文件中的Rest.inc.php。
1
|
private
function
login()
|
2
|
{
|
3
|
// Cross validation if the request method is POST else it will return "Not Acceptable" status
|
4
|
if
(
$this
->get_request_method() !=
"POST"
)
|
5
|
{
|
6
|
$this
->response(
''
,406);
|
7
|
}
|
8
|
9
|
$email
=
$this
->_request[
'email'
];
|
10
|
$password
=
$this
->_request[
'pwd'
];
|
11
|
12
|
// Input validations
|
13
|
if
(!
empty
(
$email
)
and
!
empty
(
$password
))
|
14
|
{
|
15
|
if
(filter_var(
$email
, FILTER_VALIDATE_EMAIL)){
|
16
|
$sql
= mysql_query(
"SELECT user_id, user_fullname, user_email FROM users WHERE user_email = '$email' AND user_password = '"
.md5(
$password
).
"' LIMIT 1"
,
$this
->db);
|
17
|
if
(mysql_num_rows(
$sql
) > 0){
|
18
|
$result
= mysql_fetch_array(
$sql
,MYSQL_ASSOC);
|
19
|
20
|
// If success everythig is good send header as "OK" and user details
|
21
|
$this
->response(
$this
->json(
$result
), 200);
|
22
|
}
|
23
|
$this
->response(
''
, 204);
// If no records "No Content" status
|
24
|
}
|
25
|
}
|
26
|
27
|
// If invalid inputs "Bad Request" status message and reason
|
28
|
$error
=
array
(
'status'
=>
"Failed"
,
"msg"
=>
"Invalid Email address or Password"
);
|
29
|
$this
->response(
$this
->json(
$error
), 400);
|
30
|
}
|
獲取用戶信息
通過訪問REST API 地址http://localhost/rest/users/ 獲取用戶的信息。
1
|
private
function
users()
|
2
|
{
|
3
|
// Cross validation if the request method is GET else it will return "Not Acceptable" status
|
4
|
if
(
$this
->get_request_method() !=
"GET"
)
|
5
|
{
|
6
|
$this
->response(
''
,406);
|
7
|
}
|
8
|
$sql
= mysql_query(
"SELECT user_id, user_fullname, user_email FROM users WHERE user_status = 1"
,
$this
->db);
|
9
|
if
(mysql_num_rows(
$sql
) > 0)
|
10
|
{
|
11
|
$result
=
array
();
|
12
|
while
(
$rlt
= mysql_fetch_array(
$sql
,MYSQL_ASSOC))
|
13
|
{
|
14
|
$result
[] =
$rlt
;
|
15
|
}
|
16
|
// If success everythig is good send header as "OK" and return list of users in JSON format
|
17
|
$this
->response(
$this
->json(
$result
), 200);
|
18
|
}
|
19
|
$this
->response(
''
,204);
// If no records "No Content" status
|
20
|
}
|
刪除用戶信息
根據(jù)user_id刪除特定用戶的信息,只需要訪問REST API地址http://localhost/rest/deleteUser/
1
|
private
function
deleteUser()
|
2
|
{
|
3
|
4
|
if
(
$this
->get_request_method() !=
"DELETE"
){
|
5
|
$this
->response(
''
,406);
|
6
|
}
|
7
|
$id
= (int)
$this
->_request[
'id'
];
|
8
|
if
(
$id
> 0)
|
9
|
{
|
10
|
mysql_query(
"DELETE FROM users WHERE user_id = $id"
);
|
11
|
$success
=
array
(
'status'
=>
"Success"
,
"msg"
=>
"Successfully one record deleted."
);
|
12
|
$this
->response(
$this
->json(
$success
),200);
|
13
|
}
|
14
|
else
|
15
|
{
|
16
|
$this
->response(
''
,204);
// If no records "No Content" status
|
17
|
}
|
18
|
}
|
Chrome拓展
測試PHP restful API 響應(yīng)的一個(gè)chrome的插件為 Advanced REST client Application
.htaccess code
使用.htaccess使URL更加友好。在demo示例中修改htaccess.txt to .htaccess。
1
|
<
IfModule
mod_rewrite.c>
|
2
|
RewriteEngine On
|
3
|
RewriteCond %{REQUEST_FILENAME} !-d
|
4
|
RewriteCond %{REQUEST_FILENAME} !-s
|
5
|
RewriteRule ^(.*)$ api.php?rquest=$1 [QSA,NC,L]
|
6
|
7
|
RewriteCond %{REQUEST_FILENAME} -d
|
8
|
RewriteRule ^(.*)$ api.php [QSA,NC,L]
|
9
|
10
|
RewriteCond %{REQUEST_FILENAME} -s
|
11
|
RewriteRule ^(.*)$ api.php [QSA,NC,L]
|
12
|
</
IfModule
>
|
Demo示例下載:
PHP示例源碼:REST API示例(104)原文出自: http://www.9lessons.info/2012/05/create-restful-services-api-in-php.html
本文由PHP愛好者原創(chuàng)翻譯!轉(zhuǎn)載請注明鏈接!
更多文章、技術(shù)交流、商務(wù)合作、聯(lián)系博主
微信掃碼或搜索:z360901061
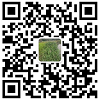
微信掃一掃加我為好友
QQ號聯(lián)系: 360901061
您的支持是博主寫作最大的動(dòng)力,如果您喜歡我的文章,感覺我的文章對您有幫助,請用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點(diǎn)擊下面給點(diǎn)支持吧,站長非常感激您!手機(jī)微信長按不能支付解決辦法:請將微信支付二維碼保存到相冊,切換到微信,然后點(diǎn)擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對您有幫助就好】元
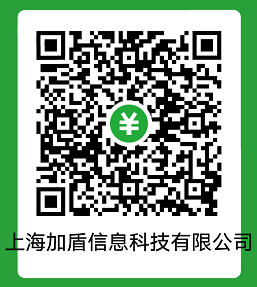