我們先實現單個按鈕,為了復用,不管單選還是復選按鈕都是使用同一個類來實現,為了區別單選還是復選,我們用一個自定義枚舉類型 CheckButtonStyle屬性style來區別,當其值設置為CheckButtonStyleDefault或CheckButtonStyleBox時,為復選按鈕:
當其值設為 CheckButtonStyleRadio時,為單選按鈕:
當按鈕在選中/反選狀態間切換時 ,文字左邊的圖片自動轉換。
整個控件是由一個 ImageView、一個Label、一個BOOL變量及其他變量組成,.h文件如下:
typedef enum {
CheckButtonStyleDefault = 0 ,
CheckButtonStyleBox = 1 ,
CheckButtonStyleRadio = 2
} CheckButtonStyle;
#import <Foundation/Foundation.h>
@interface CheckButton : UIControl {
//UIControl* control;
UILabel * label ;
UIImageView * icon ;
BOOL checked ;
id value , delegate ;
CheckButtonStyle style ;
NSString * checkname ,* uncheckname ; // 勾選/反選時的圖片文件名
}
@property ( retain , nonatomic ) id value,delegate;
@property ( retain , nonatomic )UILabel* label;
@property ( retain , nonatomic )UIImageView* icon;
@property ( assign )CheckButtonStyle style;
-( CheckButtonStyle )style;
-( void )setStyle:( CheckButtonStyle )st;
-( BOOL )isChecked;
-( void )setChecked:( BOOL )b;
@end
具體實現如下:
#import "CheckButton.h"
@implementation CheckButton
@synthesize label,icon,value,delegate;
-( id )initWithFrame:( CGRect ) frame
{
if ( self =[ super initWithFrame : frame ]) {
icon =[[ UIImageView alloc ] initWithFrame :
CGRectMake ( 10 , 0 , frame . size . height , frame . size . height )];
[ self setStyle : CheckButtonStyleDefault ]; // 默認風格為方框(多選)樣式
//self.backgroundColor=[UIColor grayColor];
[ self addSubview : icon ];
label =[[ UILabel alloc ] initWithFrame : CGRectMake ( icon . frame . size . width + 24 , 0 ,
frame . size . width - icon . frame . size . width - 24 ,
frame . size . height )];
label . backgroundColor =[ UIColor clearColor ];
label . font =[ UIFont fontWithName : @"Arial" size : 20 ];
label . textColor =[ UIColor
colorWithRed : 0xf9 / 255.0
green : 0xd8 / 255.0
blue : 0x67 / 255.0
alpha : 1 ];
label . textAlignment = UITextAlignmentLeft ;
[ self addSubview : label ];
[ self addTarget : self action : @selector ( clicked ) forControlEvents : UIControlEventTouchUpInside ];
}
return self ;
}
-( CheckButtonStyle )style{
return style ;
}
-( void )setStyle:( CheckButtonStyle )st{
style =st;
switch ( style ) {
case CheckButtonStyleDefault :
case CheckButtonStyleBox :
checkname = @"checked.png" ;
uncheckname = @"unchecked.png" ;
break ;
case CheckButtonStyleRadio :
checkname = @"radio.png" ;
uncheckname = @"unradio.png" ;
break ;
default :
break ;
}
[ self setChecked : checked ];
}
-( BOOL )isChecked{
return checked ;
}
-( void )setChecked:( BOOL )b{
if (b!= checked ){
checked =b;
}
if ( checked ) {
[ icon setImage :[ UIImage imageNamed : checkname ]];
} else {
[ icon setImage :[ UIImage imageNamed : uncheckname ]];
}
}
-( void )clicked{
[ self setChecked :! checked ];
if ( delegate != nil ) {
SEL sel= NSSelectorFromString ( @"checkButtonClicked" );
if ([ delegate respondsToSelector :sel]){
[ delegate performSelector :sel];
}
}
}
-( void )dealloc{
value = nil ; delegate = nil ;
[ label release ];
[ icon release ];
[ super dealloc ];
}
@end
使用 CheckButton類很簡單,構造、設置標簽文本等屬性,然后addSubview:
CheckButton * cb=[[ CheckButton a lloc ] initWithFrame : CGRectMake ( 20 , 60 , 260 , 32 )];
cb. label . text = @"checkbutton1" ;
cb. value =[[ NSNumber alloc ] initWithInt : 18 ];
cb. style = CheckButtonStyleDefault ;
[ self . view addSubview :cb];
二、單選按鈕組的實現
復選按鈕無所謂“組”的概念,單選按鈕則不同。在同一個組中,單選按鈕只允許同時選擇一個按鈕,不能選多個,因此我們要實現一個單選按鈕組的類:
#import <Foundation/Foundation.h>
#import "CheckButton.h"
@interface RadioGroup : NSObject {
NSMutableArray * children ;
NSString * text ;
id value ;
}
@property ( readonly )NSString* text;
@property ( readonly ) id value;
-( void )add:( CheckButton *)cb;
-( void )checkButtonClicked:( id )sender;
@end
#import "RadioGroup.h"
@implementation RadioGroup
@synthesize text,value;
-( id )init{
if ( self =[ super init ]){
children =[[ NSMutableArray alloc ] init ];
}
return self ;
}
-( void )add:( CheckButton *)cb{
cb. delegate = self ;
if (cb. checked ) {
text =cb. label . text ;
value =cb. value ;
}
[ children addObject :cb];
}
-( void )checkButtonClicked:( id )sender{
CheckButton * cb=( CheckButton *)sender;
if (!cb. checked ) {
// 實現單選
for ( CheckButton * each in children ){
if (each. checked ) {
[each setChecked : NO ];
}
}
[cb setChecked : YES ];
// 復制選擇的項
text =cb. label . text ;
value =cb. value ;
}
NSLog ( @"text:%@,value:%d" , text ,[( NSNumber *) value intValue ]);
}
-( void )dealloc{
[ text release ];
value = nil ;
[ children release ];
[ super dealloc ];
}
@end
單選按鈕組在 ViewController中的使用:
-( id )initWithNibName:( NSString *)nibNameOrNil bundle:( NSBundle *)nibBundleOrNil{
if ( self =[ super initWithNibName :nibNameOrNil bundle :nibBundleOrNil]){
// 單選按鈕組
rg =[[ RadioGroup alloc ] init ];
// 第 1 個單選按鈕
CheckButton * cb=[[ CheckButton alloc ] initWithFrame : CGRectMake ( 20 , 60 , 260 , 32 )];
// 把單選按鈕加入按鈕組
[ rg add :cb];
cb. label . text = @"★" ;
cb. value =[[ NSNumber alloc ] initWithInt : 1 ];
// 把按鈕設置為單選按鈕樣式
cb. style = CheckButtonStyleRadio ;
// 加入視圖
[ self . view addSubview :cb];
[cb release ]; //add 后,會自動持有,可以釋放
// 第 2 個單選按鈕
cb=[[ CheckButton alloc ] initWithFrame : CGRectMake ( 20 , 100 , 260 , 32 )];
[ rg add :cb];
cb. label . text = @"★★" ;
cb. value =[[ NSNumber alloc ] initWithInt : 2 ];
cb. style = CheckButtonStyleRadio ;
[ self . view addSubview :cb];
[cb release ];
// 第 3 個單選按鈕
cb=[[ CheckButton alloc ] initWithFrame : CGRectMake ( 20 , 140 , 260 , 32 )];
// 各種屬性必須在 [rg addv] 之前設置,否則 text 和 value 不會被 populate
cb. checked = YES ;
cb. label . text = @"★★★" ;
cb. value =[[ NSNumber alloc ] initWithInt : 3 ];
cb. style = CheckButtonStyleRadio ;
[ self . view addSubview :cb];
[ rg add :cb]; // 屬性設置完之后再 add
[cb release ];
// 第 4 個單選按鈕
cb=[[ CheckButton alloc ] initWithFrame : CGRectMake ( 20 , 180 , 260 , 32 )];
[ rg add :cb];
cb. label . text = @"★★★★" ;
cb. value =[[ NSNumber alloc ] initWithInt : 4 ];
cb. style = CheckButtonStyleRadio ;
[ self . view addSubview :cb];
[cb release ];
// 第 5 個單選按鈕
cb=[[ CheckButton alloc ] initWithFrame : CGRectMake ( 20 , 220 , 260 , 32 )];
[ rg add :cb];
cb. label . text = @"★★★★★" ;
cb. value =[[ NSNumber alloc ] initWithInt : 5 ];
cb. style = CheckButtonStyleRadio ;
[ self . view addSubview :cb];
[cb release ];
}
return self ;
}
運行效果:
更多文章、技術交流、商務合作、聯系博主
微信掃碼或搜索:z360901061
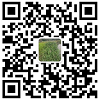
微信掃一掃加我為好友
QQ號聯系: 360901061
您的支持是博主寫作最大的動力,如果您喜歡我的文章,感覺我的文章對您有幫助,請用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點擊下面給點支持吧,站長非常感激您!手機微信長按不能支付解決辦法:請將微信支付二維碼保存到相冊,切換到微信,然后點擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對您有幫助就好】元
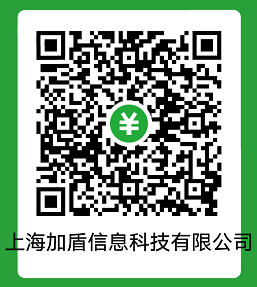