?? java不易開發(fā)大型游戲,但任一語言都有是相通,本程序運行有些小問題,僅供參考:

package cn; import java.util.*; import java.lang.*; //定義一個連線類 class LinkLine { //保存連接點 private int[] points; //接收地圖 private int[][] blocks; public LinkLine(int[][] blocks) { this.blocks = blocks; } //判斷兩個點是否能連接成線段 public boolean linkSegment(int x1 , int y1 , int x2 , int y2) { //如果在同一條垂直線上 if(x1 == x2) { if(y1 > y2) { int temp = y1; y1 = y2; y2 = temp; } for(int y = y1 ; y <= y2 ; y++) { //如果有障礙物 if(blocks[y][x1] > 0) { return false; } } return true; } //如果在同一個水平線上 else if(y1 == y2) { if(x1 > x2) { int temp = x1; x1 = x2; x2 = temp; } for(int x = x1 ; x <= x2 ; x++) { //如果有障礙物 if(blocks[y1][x] > 0) { return false; } } return true; } return false; } //是否可以連接成符合游戲規(guī)則的折線 public boolean foldLineAble(int x1 , int y1 , int x2 , int y2) { //每次都清空折點 points = null; int minDistance = 0; for(int x = x1 - 1; x >= 0; x--) //向左歷遍 { //如果第一條線段可以連接 if(linkSegment(x1 , y1 , x , y1)) { //如果剩下兩條也可以連接 if(linkSegment(x , y1 , x , y2) && linkSegment(x , y2 , x2 , y2)) { //計算最小路程 minDistance = Math.abs(x1 - x) + Math.abs(y2 - y1) + Math.abs(x2 - x); //保存折點 points = new int[]{x1 , y1 , x , y1 , x , y2 , x2 , y2}; //找到折線,不再往這個方向搜索 break; } } else { //遇到障礙,不再往這個方向搜索 break; } } for(int x = x1 + 1; x < blocks[0].length; x++) //向右歷遍 { //如果第一條線段可以連接 if(linkSegment(x1 , y1 , x , y1)) { //如果剩下兩條也可以連接 if(linkSegment(x , y1 , x , y2) && linkSegment(x , y2 , x2 , y2)) { //計算最小路程 int temp = Math.abs(x1 - x) + Math.abs(y2 - y1) + Math.abs(x2 - x); //如果小于上次一歷遍的路程或上一次歷遍沒有連接 if(temp < minDistance || minDistance == 0) { minDistance = temp; //保存折點 points = new int[]{x1 , y1 , x , y1 , x , y2 , x2 , y2}; } //找到折線,不再在這個方向搜索 break; } } else { break; } } for(int y = y1 + 1; y < blocks.length; y++) //向下歷遍 { //如果第一條線段可以連接 if(linkSegment(x1 , y1 , x1 , y)) { //如果剩下兩條也可以連接 if (linkSegment(x1 , y , x2 , y) && linkSegment(x2 , y , x2 , y2)) { //計算最小路程 int temp = Math.abs(y - y1) + Math.abs(x2 - x1) + Math.abs(y- y2); if(temp < minDistance || minDistance == 0) { minDistance = temp; //保存折點 points = new int[]{x1 , y1 , x1 , y , x2 , y , x2 , y2}; } break; } } else { break; } } for(int y = y1 - 1; y >= 0; y--) //向上歷遍 { //如果第一條線段可以連接 if(linkSegment(x1 , y1 , x1 , y)) { //如果剩下兩條也可以連接 if (linkSegment(x1 , y , x2 , y) && linkSegment(x2 , y , x2 , y2)) { //計算最小路程 int temp = Math.abs(y - y1) + Math.abs(x2 - x1) + Math.abs(y- y2); if(temp < minDistance || minDistance ==0) { minDistance = temp; //保存折點 points = new int[]{x1 , y1 , x1 , y , x2 , y , x2 , y2}; } break; } } else { break; } } if(points != null) { return true; } return false; } public boolean linkAble(int x1 , int y1 , int x2 , int y2) { boolean result = false; //如果是同一個點 if(x1 == x2 && y1 == y2) { return false; } if(blocks[y1][x1] == blocks[y2][x2]) { /* 先把要判斷連接的兩個點在地圖的值置0,避免下面的 檢測把它們當成障礙物 */ int temp1 = blocks[y1][x1]; int temp2 = blocks[y2][x2]; blocks[y1][x1] = 0; blocks[y2][x2] = 0; //先判斷是否可以連接成線段 result = linkSegment(x1 , y1 , x2 ,y2); if(result) { //保存連接點 points = new int[]{x1 , y1 , x2 ,y2}; } else { //是否可以連成折線 result = foldLineAble(x1 , y1 , x2 ,y2); } blocks[y1][x1] = temp1; blocks[y2][x2] = temp2; } return result; } //獲取連接點 public int[] getPoints() { return points; } }
package cn; import javax.swing.*; import java.io.*; import java.awt.event.*; import java.awt.*; import javax.imageio.*; import java.util.*; public class LinkGame { //定義桌面大小 private final int TABLE_WIDTH = 500; private final int TABLE_HEIGHT = 500; //定義一個10行10列的地圖數(shù)組 private final int ROW = 10; private final int COL = 10; private int[][] blocks = new int[ROW][COL]; //保存地圖塊數(shù),初始為36 private int blockCounts = 100; //每塊的障礙物的大小 private final int BLOCK_SIZE = 50; //障礙物圖形有9種 private final int BLOCK_NUM = 8; //保存障礙無圖片 private Image[] blockImage = new Image[BLOCK_NUM+1]; private Image backGroup; private Image selected ; //保存第一次選中的障礙物的數(shù)組坐標 private int fristRow = -1; private int fristCol = -1; private int secondRow = -1; private int secondCol = -1; //選中的障礙物的個數(shù) private int selectCount = 0; private Random rand = new Random(); private JFrame f = new JFrame("瘋狂的猴子I——連連看"); private Menu game = new Menu("游戲選項"); private MenuItem start = new MenuItem("開始"); private MenuBar mb = new MenuBar(); private MyTable myTable = new MyTable(); //定義一個連線類 private LinkLine lkLine = new LinkLine(blocks); //是否可以連線 private boolean isLinked = false; //用于接收折點 private int[] points; //初始畫地圖 public void initMap()throws Exception { backGroup = ImageIO.read(new File("image/back3.jpg")); selected = ImageIO.read(new File("image/selected.gif")); //獲取障礙物圖片 for(int i = 1; i < BLOCK_NUM+1; i++) { try { blockImage[i] = ImageIO.read(new File("image/"+i+".jpg")); } catch (IOException e) { e.printStackTrace(); } } /* 初始化障礙物數(shù)組,將圖片引索值依次賦給數(shù)組元素, 保證所有類型的圖片數(shù)都是偶數(shù);最外層元素全都為0, 因此不用賦值,數(shù)組模型如下: 0 0 0 0 0 0 0 0 0 1 7 3 4 5 6 0 0 7 8 6 1 2 3 0 0 4 5 6 7 8 4 0 0 1 2 3 4 5 2 0 0 7 8 6 5 7 3 0 0 8 5 2 7 8 8 0 0 0 0 0 0 0 0 0 */ int index = 1; for(int i = 1; i < ROW-1; i++) { for(int j = 1; j < COL-1; j++) { blocks[i][j] = index; index++; //如果引索值超過圖片的種類則重置引索 if(index > BLOCK_NUM) { index = 1; } } } //隨機打亂數(shù)組的排列20次 for(int k = 0; k < 20; k++) { for(int i = 1; i < ROW-1; i++) { for(int j = 1; j < COL-1; j++) { //隨機生成行號 int tempRow = rand.nextInt(ROW-2) + 1; //隨機生成列號 int tempCol = rand.nextInt(COL-2) + 1; //如果不是同一個元素,則交換兩個元素 if(tempRow != i || tempCol != j) { int temp = blocks[tempRow][tempCol]; blocks[tempRow][tempCol] = blocks[i][j]; blocks[i][j] = temp; } } } } myTable.repaint(); } //初始化組件 public void initComponent() { //“開始”監(jiān)聽事件 start.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent event) { try { initMap(); } catch (Exception e) { System.out.println(e.getMessage()); } } }); game.add(start); mb.add(game); f.setMenuBar(mb); f.add(myTable); myTable.setPreferredSize( new Dimension(TABLE_WIDTH,TABLE_HEIGHT)); f.pack(); f.setVisible(true); } public void init() { //游戲桌面鼠標按下事件 myTable.addMouseListener(new MouseAdapter() { public void mousePressed(MouseEvent e) { //將桌面坐標轉(zhuǎn)換為數(shù)組坐標 int xPos = e.getX() / BLOCK_SIZE; int yPos = e.getY() / BLOCK_SIZE; //如果超出地圖范圍,直接退出方法 if(xPos < 1 || xPos > COL-2 || yPos < 1 || yPos > ROW-2) { return; } selectCount ++; switch(selectCount) { case 1: //第一次選中 fristRow = yPos; fristCol = xPos; break; case 2: //第二次選中 secondRow = yPos; secondCol = xPos; break; default : break; } if(selectCount == 2) { //判斷選中的障礙物是否可以連線 isLinked = lkLine.linkAble(fristCol , fristRow , secondCol , secondRow); //System.out.println(isLinked); //如果連線成功 if(isLinked) { //清楚障礙物 blocks[fristRow][fristCol] = 0; blocks[secondRow][secondCol] = 0; //獲取折點 points = lkLine.getPoints(); } } myTable.repaint(); } //游戲桌面鼠標松開事件 public void mouseReleased(MouseEvent e) { //重置 if(selectCount == 2) { selectCount=0; } myTable.repaint(); } }); } public static void main(String[] args)throws Exception { LinkGame lg = new LinkGame(); //初始化地圖 lg.initMap(); //初始化組件 lg.initComponent(); //初始游戲 lg.init(); } public void drawSelected(Graphics g) { int fristX ; int fristY ; int secondX ; int secondY ; //第一次選中或第2次選種 if(selectCount == 1 || selectCount == 2) { g.drawImage(selected , fristCol*BLOCK_SIZE , fristRow*BLOCK_SIZE , BLOCK_SIZE , BLOCK_SIZE , null); } //第2次選種 if(selectCount == 2) { g.drawImage(selected , secondCol * BLOCK_SIZE , secondRow * BLOCK_SIZE , BLOCK_SIZE , BLOCK_SIZE ,null); //如果連接成功 if(isLinked) { //如果連接點為空直接返回 if(points == null){return ;}; //繪制連接線 int index = 0; fristX = points[index++] ; fristY = points[index++] ; for(int i = index; i < points.length;) { secondX = fristX ; secondY = fristY ; fristX = points[i++] ; fristY = points[i++] ; g.drawLine( fristX*BLOCK_SIZE + 25 , fristY*BLOCK_SIZE + 25 , secondX*BLOCK_SIZE + 25 , secondY*BLOCK_SIZE + 25 ); } } } } class MyTable extends JPanel { public void paint(Graphics g) { //背景 g.drawImage(backGroup , 0 , 0 ,TABLE_WIDTH , TABLE_HEIGHT ,null); //繪制障礙物 for(int i = 0; i < ROW; i++) { for(int j = 0; j < COL; j++) { //是否有障礙物 if(blocks[i][j] != 0) { int index = blocks[i][j]; g.drawImage( blockImage[index] , j*BLOCK_SIZE , i*BLOCK_SIZE , BLOCK_SIZE , BLOCK_SIZE , null ); //繪制每一塊障礙物的邊框 g.drawRect( j*BLOCK_SIZE , i*BLOCK_SIZE , BLOCK_SIZE , BLOCK_SIZE ); } } } //繪制選中點的紅框 drawSelected(g); } } }
更多文章、技術(shù)交流、商務合作、聯(lián)系博主
微信掃碼或搜索:z360901061
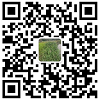
微信掃一掃加我為好友
QQ號聯(lián)系: 360901061
您的支持是博主寫作最大的動力,如果您喜歡我的文章,感覺我的文章對您有幫助,請用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點擊下面給點支持吧,站長非常感激您!手機微信長按不能支付解決辦法:請將微信支付二維碼保存到相冊,切換到微信,然后點擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對您有幫助就好】元
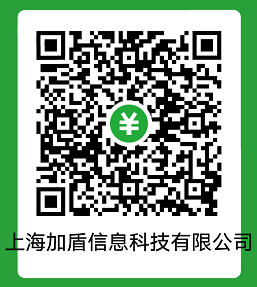