將jdbc結果集轉換成對象列表
估計hibernate就是用得這種方式進行轉換的。
實體對象
- package ?test; ??
- //實體對象,該對象的屬性與數據庫中的字段相同,當然可以改變具體看需求 ??
- public ? class ?Person?{ ??
- ???? private ? int ?id; ??
- ???? private ? int ?age; ??
- ???? private ?String?name; ??
- ???? public ? int ?getId()?{ ??
- ???????? return ?id; ??
- ????} ??
- ???? public ? void ?setId( int ?id)?{ ??
- ???????? this .id?=?id; ??
- ????} ??
- ???? public ? int ?getAge()?{ ??
- ???????? return ?age; ??
- ????} ??
- ???? public ? void ?setAge( int ?age)?{ ??
- ???????? this .age?=?age; ??
- ????} ??
- ???? public ?String?getName()?{ ??
- ???????? return ?name; ??
- ????} ??
- ???? public ? void ?setName(String?name)?{ ??
- ???????? this .name?=?name; ??
- ????} ??
- ???? ??
- }??
?
- package ?test; ??
- ??
- import ?java.lang.reflect.Field; ??
- import ?java.sql.Connection; ??
- import ?java.sql.PreparedStatement; ??
- import ?java.sql.ResultSet; ??
- import ?java.sql.SQLException; ??
- import ?java.util.List; ??
- ??
- public ? class ?Main?{ ??
- ???? //用于測試的方法 ??
- ???? public ? static ? void ?main(String[]?args)? throws ?InstantiationException,?IllegalAccessException,?IllegalArgumentException,?ClassNotFoundException?{ ??
- ????????Connection?conn?=?DbUtils.getConn(); ??
- ????????ResultSet?rs?=? null ; ??
- ????????PreparedStatement?psmt?=? null ; ??
- ????????System.out.println(conn); ??
- ???????? try ?{ ??
- ????????????psmt?=?conn.prepareStatement( "select?*?from?person" ); ??
- ????????????rs?=?psmt.executeQuery(); ??
- ????????????List?list?=?DbUtils.populate(rs,?Person. class ); ??
- ???????????? for ( int ?i?=? 0 ?;?i<list.size()?;?i++){ ??
- ????????????????Person?per?=?(Person)?list.get(i); ??
- ????????????????System.out.println( "person?:?id?=?" +per.getId()+ "?name?=?" +per.getName()+ "?age?=?" +per.getAge()); ??
- ????????????} ??
- ????????}? catch ?(SQLException?e)?{ ??
- ????????????e.printStackTrace(); ??
- ????????} finally { ??
- ???????????? if (rs!= null ){ ??
- ???????????????? try ?{ ??
- ????????????????????rs.close(); ??
- ????????????????}? catch ?(SQLException?e)?{ ??
- ????????????????????e.printStackTrace(); ??
- ????????????????} ??
- ????????????????rs= null ; ??
- ????????????} ??
- ???????????? if (psmt!= null ){ ??
- ???????????????? try ?{ ??
- ????????????????????psmt.close(); ??
- ????????????????}? catch ?(SQLException?e)?{ ??
- ????????????????????e.printStackTrace(); ??
- ????????????????} ??
- ????????????????psmt= null ; ??
- ????????????} ??
- ???????????? if (conn!= null ){ ??
- ???????????????? try ?{ ??
- ????????????????????conn.close(); ??
- ????????????????}? catch ?(SQLException?e)?{ ??
- ????????????????????e.printStackTrace(); ??
- ????????????????} ??
- ????????????????conn= null ; ??
- ????????????} ??
- ????????} ??
- ???????? ??
- ????} ??
- ??
- }??
- package ?test; ??
- ??
- import ?java.lang.reflect.Field; ??
- import ?java.sql.Connection; ??
- import ?java.sql.DriverManager; ??
- import ?java.sql.ResultSet; ??
- import ?java.sql.ResultSetMetaData; ??
- import ?java.sql.SQLException; ??
- import ?java.util.ArrayList; ??
- import ?java.util.List; ??
- ??
- public ? class ?DbUtils?{ ??
- ???? private ? static ?String?url?=? "jdbc:mysql://localhost:3306/test" ; ??
- ???? private ? static ?String?username?=? "root" ; ??
- ???? private ? static ?String?password?=? "" ; ??
- ???? private ? static ?String?driverClass?=? "com.mysql.jdbc.Driver" ; ??
- ???? //沒什么好說的,獲取數據庫連接 ??
- ???? public ? static ?Connection?getConn(){ ??
- ????????Connection?conn?=? null ; ??
- ???????? try ?{ ??
- ????????????Class.forName(driverClass); ??
- ????????????conn?=?DriverManager.getConnection(url,username,password); ??
- ????????}? catch ?(ClassNotFoundException?e)?{ ??
- ????????????e.printStackTrace(); ??
- ????????}? catch ?(SQLException?e)?{ ??
- ????????????e.printStackTrace(); ??
- ????????} ??
- ???????? ??
- ???????? return ?conn; ??
- ????} ??
- ???? /* ?
- ?????*?將rs結果轉換成對象列表 ?
- ?????*?@param?rs?jdbc結果集 ?
- ?????*?@param?clazz?對象的映射類 ?
- ?????*?return?封裝了對象的結果列表 ?
- ?????*/ ??
- ???? public ? static ?List?populate(ResultSet?rs?,?Class?clazz)? throws ?SQLException,?InstantiationException,?IllegalAccessException{ ??
- ???????? //結果集的元素對象? ??
- ????????ResultSetMetaData?rsmd?=?rs.getMetaData(); ??
- ???????? //獲取結果集的元素個數 ??
- ????????? int ?colCount?=?rsmd.getColumnCount(); ??
- //???????System.out.println("#"); ??
- //???????for(int?i?=?1;i<=colCount;i++){ ??
- //???????????System.out.println(rsmd.getColumnName(i)); ??
- //???????????System.out.println(rsmd.getColumnClassName(i)); ??
- //???????????System.out.println(rsmd.getColumnClassLabel(i)); ??
- //???????} ??
- ????????? //返回結果的列表集合 ??
- ?????????List?list?=? new ?ArrayList(); ??
- ????????? //業務對象的屬性數組 ??
- ?????????Field[]?fields?=?clazz.getDeclaredFields(); ??
- ????????? while (rs.next()){ //對每一條記錄進行操作 ??
- ?????????????Object?obj?=?clazz.newInstance(); //構造業務對象實體 ??
- ????????????? //將每一個字段取出進行賦值 ??
- ????????????? for ( int ?i?=? 1 ;i<=colCount;i++){ ??
- ?????????????????Object?value?=?rs.getObject(i); ??
- ????????????????? //尋找該列對應的對象屬性 ??
- ????????????????? for ( int ?j= 0 ;j<fields.length;j++){ ??
- ?????????????????????Field?f?=?fields[j]; ??
- ????????????????????? //如果匹配進行賦值 ??
- ????????????????????? if (f.getName().equalsIgnoreCase(rsmd.getColumnName(i))){ ??
- ????????????????????????? boolean ?flag?=?f.isAccessible(); ??
- ?????????????????????????f.setAccessible( true ); ??
- ?????????????????????????f.set(obj,?value); ??
- ?????????????????????????f.setAccessible(flag); ??
- ?????????????????????} ??
- ?????????????????} ??
- ?????????????} ??
- ?????????????list.add(obj); ??
- ?????????} ??
- ???????? return ?list; ??
- ????} ??
- }??
更多文章、技術交流、商務合作、聯系博主
微信掃碼或搜索:z360901061
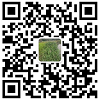
微信掃一掃加我為好友
QQ號聯系: 360901061
您的支持是博主寫作最大的動力,如果您喜歡我的文章,感覺我的文章對您有幫助,請用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點擊下面給點支持吧,站長非常感激您!手機微信長按不能支付解決辦法:請將微信支付二維碼保存到相冊,切換到微信,然后點擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對您有幫助就好】元
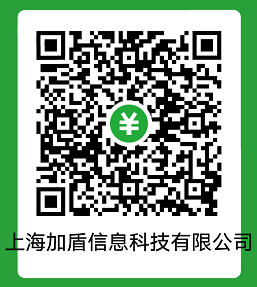