iBatis學習筆記:(versions 2.2.0 and higher)
配置文件SqlMapConfig.xml:
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE sqlMapConfig
PUBLIC "-//ibatis.apache.org//DTD SQL Map Config 2.0//EN"
"http://ibatis.apache.org/dtd/sql-map-config-2.dtd">
<!-- Always ensure to use the correct XML header as above! -->
<sqlMapConfig>
<!-- The properties (name=value) in the file specified here can be used placeholders in this config
file (e.g. “${driver}”. The file is relative to the classpath and is completely optional. -->
<properties resource=" examples/sqlmap/maps/SqlMapConfigExample.properties " />
<!-- These settings control SqlMapClient configuration details, primarily to do with transaction
management. They are all optional (more detail later in this document). -->
<settings
cacheModelsEnabled="true"
enhancementEnabled="true"
lazyLoadingEnabled="true"
maxRequests="128"
maxSessions="10"
maxTransactions="5"
useStatementNamespaces="false"
defaultStatementTimeout="5"
statementCachingEnabled="true"
classInfoCacheEnabled="true"
/>
<!-- This element declares a factory class that iBATIS will use for creating result objects.
This element is optional (more detail later in this document). -->
<resultObjectFactory type="com.mydomain.MyResultObjectFactory" >
<property name="someProperty" value="someValue"/>
</resultObjectFactory>
<!-- Type aliases allow you to use a shorter name for long fully qualified class names. -->
<typeAlias alias="order" type="testdomain.Order"/>
<!-- Configure a datasource to use with this SQL Map using SimpleDataSource.
Notice the use of the properties from the above resource -->
<transactionManager type="JDBC" >
<dataSource type="SIMPLE">
<property name="JDBC.Driver" value="${driver}"/>
<property name="JDBC.ConnectionURL" value="${url}"/>
<property name="JDBC.Username" value="${username}"/>
<property name="JDBC.Password" value="${password}"/>
<property name="JDBC.DefaultAutoCommit" value="true" />
<property name="Pool.MaximumActiveConnections" value="10"/>
<property name="Pool.MaximumIdleConnections" value="5"/>
<property name="Pool.MaximumCheckoutTime" value="120000"/>
<property name="Pool.TimeToWait" value="500"/>
<property name="Pool.PingQuery" value="select 1 from ACCOUNT"/>
<property name="Pool.PingEnabled" value="false"/>
<property name="Pool.PingConnectionsOlderThan" value="1"/>
<property name="Pool.PingConnectionsNotUsedFor" value="1"/>
</dataSource>
</transactionManager>
<!-- Identify all SQL Map XML files to be loaded by this SQL map. Notice the paths
are relative to the classpath. For now, we only have one… -->
<sqlMap resource="examples/sqlmap/maps/Person.xml" />
</sqlMapConfig>
其中:
<properties>元素指定了一個標準java properties文件的位置,用classpath相對位置或URL形式指定,之后整個配置文件以及所有被包含進來的映射文件都可以用${key}形式的占位符來獲取properties文件中的value值。
<settings>元素:
設置一些全局屬性,如延遲加載、緩存模式等。
所有可設置屬性及其說明如下:
maxRequests This is the maximum number of threads that can execute an SQL
statement at a time. Threads beyond the set value will be blocked until
another thread completes execution. Different DBMS have different
limits, but no database is without these limits. This should usually be at
least 10 times maxTransactions (see below) and should always be greater
than both maxSessions and maxTransactions. Often reducing the
maximum number of concurrent requests can increase performance.
Example: maxRequests= ”256”
Default: 512
maxSessions This is the number of sessions (or clients) that can be active at a given
time. A session is either an explicit session, requested
programmatically, or it is automatic whenever a thread makes use of an
SqlMapClient instance (e.g. executes a statement etc.). This should
always be greater than or equal to maxTransactions and less than
maxRequests. Reducing the maximum number of concurrent sessions
can reduce the overall memory footprint.
Example: maxSessions= ”64”
Default: 128
maxTransactions This is the maximum number of threads that can enter
SqlMapClient.startTransaction() at a time. Threads beyond the set value
will be blocked until another thread exits. Different DBMS have
different limits, but no database is without these limits. This value
should always be less than or equal to maxSessions and always much
less than maxRequests. Often reducing the maximum number of
concurrent transactions can increase performance.
Example: maxTransactions= ”16”
Default: 32
cacheModelsEnabled This setting globally enables or disables all cache models for an
SqlMapClient. This can come in handy for debugging.
Example: cacheModelsEnabled= ”true”
Default: true (enabled)
lazyLoadingEnabled This setting globally enables or disables all lazy loading for an
SqlMapClient. This can come in handy for debugging.
Example: lazyLoadingEnabled= ”true”
Default: true (enabled)
enhancementEnabled This setting enables runtime bytecode enhancement to facilitate
optimized JavaBean property access as well as enhanced lazy loading.
Example: enhancementEnabled = ”true”
Default: false (disabled)
useStatementNamespaces With this setting enabled, you must always refer to mapped statements
by their fully qualified name, which is the combination of the sqlMap
name and the statement name. For example:
queryForObject(“sqlMapName.statementName”);
Example: useStatementNamespaces= ”false”
Default: false (disabled)
defaultStatementTimeout (iBATIS versions 2.2.0 and later)
This setting is an integer value that will be applied as the JDBC query
timeout for all statements. This value can be overridden with the
“statement” attribute of any mapped statement. If not specified, no
query timeout will be set unless specified on the “statement” attribute of
a mapped statement. The specified value is the number of seconds the
driver will wait for a statement to finish. Note that not all drivers
support this setting.
classInfoCacheEnabled With this setting enabled, iBATIS will maintain a cache of introspected
classes. This will lead to a significant reduction in startup time if many
classes are reused.
Example: classInfoCacheEnabled= “true”
Default: true (enabled)
statementCachingEnabled (iBATIS versions 2.3.0 and later)
With this setting enabled, iBATIS will maintain a local cache of
prepared statements. This can lead to significant performance
improvements.
Example: statementCachingEnabled= “true”
Default: true (enabled)
<resultObjectFactory>元素:
指定對象生成工廠類,用于將查詢結果封裝成對象返回。這是可選設置,若不指定,ibatis將使用Class.newInstance()的方式生成查詢結果類。對象工廠類必須實現com.ibatis.sqlmap.engine.mapping.result.ResultObjectFactory接口。
<typeAlias>元素:
用來為一些亢長的類名起“別名”,例如:
<typeAlias alias="shortname" type="com.long.class.path.Class"/>
以后就能用“shortname”來取代“com.long.class.path.Class”了。
除了自己定義別名外,iBatis框架預先定義了一些別名,以方便使用,他們是:
Transaction Manager Aliases:
JDBC??? com.ibatis.sqlmap.engine.transaction.jdbc.JdbcTransactionConfig
JTA???? com.ibatis.sqlmap.engine.transaction.jta.JtaTransactionConfig
EXTERNAL com.ibatis.sqlmap.engine.transaction.external.ExternalTransactionConfig
Data Source Factory Aliases:
SIMPLE?? com.ibatis.sqlmap.engine.datasource.SimpleDataSourceFactory
DBCP???? com.ibatis.sqlmap.engine.datasource.DbcpDataSourceFactory
JNDI????? com.ibatis.sqlmap.engine.datasource.JndiDataSourceFactory
<transactionManager>元素:
設置事務類型和<dataSource>元素,如上文所說,預定義的事務類型有JDBC, JTA, EXTERNAL;數據源類型有SIMPLE, DBCP, JNDI;若指定EXTERNAL或JTA,那就還有額外的屬性需要設置:
?
<dataSource>元素:
在transactionManager元素中,定義數據源。預定義三種數據源工廠:SIMPLE, DBCP, JNDI,不過也可以自己寫一個。
SIMPLE:在沒有容器數據源支持的情況下使用的最簡單的數據源實現,具體設置見剛才的例子。
DBCP:使用apache的DBCP數據源,ibatis框架對其直接提供支持,設置方法如下:
<transactionManager type="JDBC">
<dataSource type="DBCP">
<property name="driverClassName" value="${driver}"/>
<property name="url" value="${url}"/>
<property name="username" value="${username}"/>
<property name="password" value="${password}"/>
<!-- OPTIONAL PROPERTIES BELOW -->
<property name="maxActive" value="10"/>
<property name="maxIdle" value="5"/>
<property name="maxWait" value="60000"/>
<!-- Use of the validation query can be problematic.
If you have difficulty, try without it. -->
<property name="validationQuery" value="select * from ACCOUNT"/>
<property name="logAbandoned" value="false"/>
<property name="removeAbandoned" value="false"/>
<property name="removeAbandonedTimeout" value="50000"/>
<property name="Driver.DriverSpecificProperty" value="SomeValue"/>
</datasource>
</transactionManager>
所有的設置屬性請參考: http://jakarta.apache.org/commons/dbcp/configuration.html
注:以‘Driver.’開頭的屬性會被加入到JDBC的屬性中(有些JDBC需要)。
JNDI:指定配置的JNDI數據源。設置格式:
<transactionManager type="JDBC" >
<dataSource type="JNDI">
<property name="DataSource" value="java:comp/env/jdbc/jpetstore"/>
</dataSource>
</transactionManager>
上面只是使用普通的JDBC事務,但通常設置JNDI數據源更愿意用JTA全局事務:
<transactionManager type="JTA" >
<property name="UserTransaction" value="java:/comp/UserTransaction"/>
<dataSource type="JNDI">
<property name="DataSource" value="java:comp/env/jdbc/jpetstore"/>
</dataSource>
</transactionManager>
<sqlMap>元素:
用來包含SQL映射文件或另一個配置文件,以classpath或URL的形式:
<!-- CLASSPATH RESOURCES -->
<sqlMap resource="com/ibatis/examples/sql/Customer.xml" />
<sqlMap resource="com/ibatis/examples/sql/Account.xml" />
<sqlMap resource="com/ibatis/examples/sql/Product.xml" />
<!-- URL RESOURCES -->
<sqlMap url="file:///c:/config/Customer.xml " />
<sqlMap url="file:///c:/config/Account.xml " />
<sqlMap url="file:///c:/config/Product.xml" />
更多文章、技術交流、商務合作、聯系博主
微信掃碼或搜索:z360901061
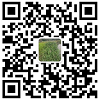
微信掃一掃加我為好友
QQ號聯系: 360901061
您的支持是博主寫作最大的動力,如果您喜歡我的文章,感覺我的文章對您有幫助,請用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點擊下面給點支持吧,站長非常感激您!手機微信長按不能支付解決辦法:請將微信支付二維碼保存到相冊,切換到微信,然后點擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對您有幫助就好】元
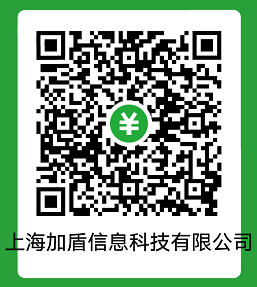