在Android中,兩個Acitivity之間是靠Intent傳遞信息的,因為Intent本來就起到信使的作用,所以用它來傳遞數(shù)據(jù)也顯得順理成章了.
Intent 提供了多個方法來"攜帶"額外的數(shù)據(jù)
putExtras(Bundle data): 向Intent中放入需要"攜帶"的數(shù)據(jù)
putXxx(String key,Xxx date):向Bundle放入Int,Long等各種類型的數(shù)據(jù)(Xxx指代各種數(shù)據(jù)類型的名稱)
putSerializable(String key,Serializable date):向Bundle中放入一個可序列化的對象.
當(dāng)然Intent也提供了相應(yīng)的取出"攜帶"數(shù)據(jù)的方法
getXxx(String key):從Bundle取出Int,Long 等各種數(shù)據(jù)類型的數(shù)據(jù).
getSerializable(String Key,Serializable data): 從Bundle取出一個可序列化的對象.
下面以使用getSerializable為例,定義一個可序列化的Person類,模擬一個用戶注冊的過程,通過注冊那個窗口(Acitivity)傳遞注冊信息到另一個窗口
下面是定義的一個DTO類Person用來記錄注冊的信息,注意!要定義成可序列化的類,繼承Serializable
package WangLi.Activity.Bundle; import java.io.Serializable; public class Person implements Serializable { private String _Name; private String _Passwd; private String _Gender; public String getName() { return _Name; } public String getPass() { return _Passwd; } public String getGender() { return _Gender; } public Person(String Name,String Passwd,String Gender) { this._Name = Name; this._Passwd = Passwd; this._Gender = Gender; } }
第一個Activity界面如圖
填入注冊信息后,點"注冊"后跳到新窗口,顯示剛剛輸入的信息
下面是注冊窗口,界面xml 和代碼
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:orientation="vertical" > <TableLayout android:layout_width="fill_parent" android:layout_height="wrap_content" > <TextView android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="請輸入您的注冊信息" android:textSize="20sp" /> <TableRow> <TextView android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="用戶名:" android:textSize="16sp" /> <EditText android:id="@+id/name" android:layout_width="fill_parent" android:layout_height="wrap_content" android:hint="請?zhí)顚懴胱缘馁~號" android:selectAllOnFocus="true" /> </TableRow> <TableRow> <TextView android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="密碼:" android:textSize="16sp" /> <EditText android:id="@+id/passwd" android:layout_width="fill_parent" android:layout_height="wrap_content" android:password = "true" android:selectAllOnFocus="true" /> </TableRow> <TableRow> <TextView android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="性別" android:textSize="16sp" /> <RadioGroup android:layout_width="fill_parent" android:layout_height="wrap_content" android:orientation="horizontal" > <RadioButton android:id="@+id/male" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="男" android:textSize="16sp" /> <RadioButton android:id="@+id/female" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="女" android:textSize="16sp" /> </RadioGroup> </TableRow> <TableRow> <Button android:id="@+id/bn" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text='注冊' android:textSize="16sp" /> </TableRow> </TableLayout> </LinearLayout>
package WangLi.Activity.Bundle; import android.app.Activity; import android.content.Intent; import android.os.Bundle; import android.view.View; import android.view.View.OnClickListener; import android.widget.Button; import android.widget.EditText; import android.widget.RadioButton; public class BundleTest extends Activity { /** Called when the activity is first created. */ @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); Button bn = (Button)findViewById(R.id.bn); bn.setOnClickListener(new OnClickListener() { public void onClick(View v) { EditText name = (EditText)findViewById(R.id.name); EditText passwd = (EditText)findViewById(R.id.passwd); RadioButton male = (RadioButton)findViewById(R.id.male); String gender = male.isChecked() ? "男" : "女"; Person p = new Person(name.getText().toString(),passwd.getText().toString(),gender); //創(chuàng)建Bundle對象 Bundle data = new Bundle(); data.putSerializable("person", p); //創(chuàng)建一個Intent Intent intent = new Intent(BundleTest.this,ResultActivity.class); intent.putExtras(data); //啟動intent對應(yīng)的Activity startActivity(intent); } }); } }
下面是第接受信息窗口在接受到注冊信息以后的樣子
第二個接受信息窗口界面xml 及代碼
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:orientation="vertical" > <TableLayout android:layout_width="fill_parent" android:layout_height="wrap_content" > <TextView android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="請輸入您的注冊信息" android:textSize="20sp" /> <TableRow> <TextView android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="用戶名:" android:textSize="16sp" /> <EditText android:id="@+id/name" android:layout_width="fill_parent" android:layout_height="wrap_content" android:hint="請?zhí)顚懴胱缘馁~號" android:selectAllOnFocus="true" /> </TableRow> <TableRow> <TextView android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="密碼:" android:textSize="16sp" /> <EditText android:id="@+id/passwd" android:layout_width="fill_parent" android:layout_height="wrap_content" android:password = "true" android:selectAllOnFocus="true" /> </TableRow> <TableRow> <TextView android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="性別" android:textSize="16sp" /> <RadioGroup android:layout_width="fill_parent" android:layout_height="wrap_content" android:orientation="horizontal" > <RadioButton android:id="@+id/male" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="男" android:textSize="16sp" /> <RadioButton android:id="@+id/female" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="女" android:textSize="16sp" /> </RadioGroup> </TableRow> <TableRow> <Button android:id="@+id/bn" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text='注冊' android:textSize="16sp" /> </TableRow> </TableLayout> </LinearLayout>
package WangLi.Activity.Bundle; import android.app.Activity; import android.content.Intent; import android.os.Bundle; import android.widget.TextView; public class ResultActivity extends Activity { @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.result); TextView name = (TextView)findViewById(R.id.name); TextView passwd = (TextView)findViewById(R.id.passwd); TextView gender = (TextView)findViewById(R.id.gender); //獲取啟動該Result的Intent Intent intent = getIntent(); //獲取該intent所攜帶的數(shù)據(jù) Bundle data = intent.getExtras(); //從Bundle包中取出數(shù)據(jù) Person p = (Person)data.getSerializable("person"); name.setText("用戶名:"+p.getName()); passwd.setText("密碼:"+p.getPass()); gender.setText("性別:"+p.getGender()); } }
當(dāng)然,最后也別忘了把所有Activity都加入AndroidManifest.xml中
更多文章、技術(shù)交流、商務(wù)合作、聯(lián)系博主
微信掃碼或搜索:z360901061
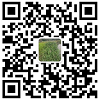
微信掃一掃加我為好友
QQ號聯(lián)系: 360901061
您的支持是博主寫作最大的動力,如果您喜歡我的文章,感覺我的文章對您有幫助,請用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點擊下面給點支持吧,站長非常感激您!手機微信長按不能支付解決辦法:請將微信支付二維碼保存到相冊,切換到微信,然后點擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對您有幫助就好】元
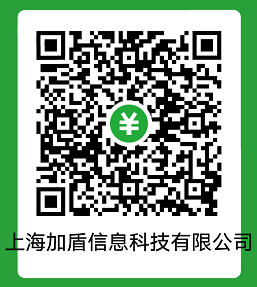