JPA多對(duì)多
維護(hù)端注解
@ManyToMany (cascade?=?CascadeType.REFRESH)
@JoinTable (//關(guān)聯(lián)表
?????????????????? name?=? "student_teacher" , //關(guān)聯(lián)表名
?????????????????? inverseJoinColumns?=? @JoinColumn (name?=? "teacher_id" ),//被維護(hù)端外鍵
?????????????????? joinColumns?=? @JoinColumn (name?=? "student_id" ))//維護(hù)端外鍵
被維護(hù)端注解
@ManyToMany(
?????????????????? cascade = CascadeType.REFRESH,
?????????????????? mappedBy = "teachers",//通過維護(hù)端的屬性關(guān)聯(lián)
?????????????????? fetch = FetchType.LAZY)
關(guān)系維護(hù)端刪除時(shí),如果中間表存在些紀(jì)錄的關(guān)聯(lián)信息,則會(huì)刪除該關(guān)聯(lián)信息;
關(guān)系被維護(hù)端刪除時(shí),如果中間表存在些紀(jì)錄的關(guān)聯(lián)信息,則會(huì)刪除失敗 .
?
?
以學(xué)生和老師的對(duì)應(yīng)關(guān)系為例。一個(gè)學(xué)生可以擁有多個(gè)老師,一個(gè)老師也可以擁有多個(gè)學(xué)生。
學(xué)生實(shí)體類
- package?com.taoistwar.jpa.entity.manytomany; ??
- ??
- import?java.util.HashSet; ??
- import?java.util.Set; ??
- ??
- import?javax.persistence.CascadeType; ??
- import?javax.persistence.Column; ??
- import?javax.persistence.Entity; ??
- import?javax.persistence.GeneratedValue; ??
- import?javax.persistence.GenerationType; ??
- import?javax.persistence.Id; ??
- import?javax.persistence.JoinColumn; ??
- import?javax.persistence.JoinTable; ??
- import?javax.persistence.ManyToMany; ??
- ??
- @Entity ??
- public?class?Student?{ ??
- ????private?Integer?id; ??
- ????private?String?name; ??
- ????private?Set<Teacher>?teachers?=?new?HashSet<Teacher>(); ??
- ??
- ????@Id ??
- ????@GeneratedValue(strategy?=?GenerationType.AUTO) ??
- ????public?Integer?getId()?{ ??
- ????????return?id; ??
- ????} ??
- ??
- ????public?void?setId(Integer?id)?{ ??
- ????????this.id?=?id; ??
- ????} ??
- ??
- ????@Column(nullable?=?false,?length?=? 16 ) ??
- ????public?String?getName()?{ ??
- ????????return?name; ??
- ????} ??
- ??
- ????public?void?setName(String?name)?{ ??
- ????????this.name?=?name; ??
- ????} ??
- ??
- ????@ManyToMany(cascade?=?CascadeType.REFRESH) ??
- ????@JoinTable(name?=? "student_teacher" ,?inverseJoinColumns?=?@JoinColumn(name?=? "teacher_id" ),?joinColumns?=?@JoinColumn(name?=? "student_id" )) ??
- ????public?Set<Teacher>?getTeachers()?{ ??
- ????????return?teachers; ??
- ????} ??
- ??
- ????public?void?setTeachers(Set<Teacher>?teachers)?{ ??
- ????????this.teachers?=?teachers; ??
- ????} ??
- ??
- ????public?void?addTeacher(Teacher?teacher)?{ ??
- ????????this.teachers.add(teacher); ??
- ????} ??
- ??
- ????public?void?removeTeachers(Teacher?teacher)?{ ??
- ????????this.teachers.remove(teacher); ??
- ????} ??
- ??
- }??
package com.taoistwar.jpa.entity.manytomany; import java.util.HashSet; import java.util.Set; import javax.persistence.CascadeType; import javax.persistence.Column; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.GenerationType; import javax.persistence.Id; import javax.persistence.JoinColumn; import javax.persistence.JoinTable; import javax.persistence.ManyToMany; @Entity public class Student { private Integer id; private String name; private Set<Teacher> teachers = new HashSet<Teacher>(); @Id @GeneratedValue(strategy = GenerationType.AUTO) public Integer getId() { return id; } public void setId(Integer id) { this.id = id; } @Column(nullable = false, length = 16) public String getName() { return name; } public void setName(String name) { this.name = name; } @ManyToMany(cascade = CascadeType.REFRESH) @JoinTable(name = "student_teacher", inverseJoinColumns = @JoinColumn(name = "teacher_id"), joinColumns = @JoinColumn(name = "student_id")) public Set<Teacher> getTeachers() { return teachers; } public void setTeachers(Set<Teacher> teachers) { this.teachers = teachers; } public void addTeacher(Teacher teacher) { this.teachers.add(teacher); } public void removeTeachers(Teacher teacher) { this.teachers.remove(teacher); } }
?重點(diǎn)在于:
- @ManyToMany (cascade?=?CascadeType.REFRESH) ??
- ???? @JoinTable (name?=? "student_teacher" ,?inverseJoinColumns?=? @JoinColumn (name?=? "teacher_id" ),?joinColumns?=? @JoinColumn (name?=? "student_id" )) ??
- ???? public ?Set<Teacher>?getTeachers()?{ ??
- ???????? return ?teachers; ??
- ????}??
@ManyToMany(cascade = CascadeType.REFRESH) @JoinTable(name = "student_teacher", inverseJoinColumns = @JoinColumn(name = "teacher_id"), joinColumns = @JoinColumn(name = "student_id")) public Set<Teacher> getTeachers() { return teachers; }
?老師實(shí)體類
- package?com.taoistwar.jpa.entity.manytomany; ??
- ??
- import?java.util.HashSet; ??
- import?java.util.Set; ??
- ??
- import?javax.persistence.CascadeType; ??
- import?javax.persistence.Column; ??
- import?javax.persistence.Entity; ??
- import?javax.persistence.FetchType; ??
- import?javax.persistence.GeneratedValue; ??
- import?javax.persistence.GenerationType; ??
- import?javax.persistence.Id; ??
- import?javax.persistence.ManyToMany; ??
- ??
- @Entity ??
- public?class?Teacher?{ ??
- ????private?Integer?id; ??
- ????private?String?name; ??
- ????private?Set<Student>?students?=?new?HashSet<Student>(); ??
- ??
- ????@Id ??
- ????@GeneratedValue(strategy?=?GenerationType.AUTO) ??
- ????public?Integer?getId()?{ ??
- ????????return?id; ??
- ????} ??
- ??
- ????public?void?setId(Integer?id)?{ ??
- ????????this.id?=?id; ??
- ????} ??
- ??
- ????@Column(nullable?=?false,?length?=? 16 ) ??
- ????public?String?getName()?{ ??
- ????????return?name; ??
- ????} ??
- ??
- ????public?void?setName(String?name)?{ ??
- ????????this.name?=?name; ??
- ????} ??
- ??
- ????@ManyToMany(cascade?=?CascadeType.REFRESH,?mappedBy?=? "teachers" ,?fetch?=?FetchType.LAZY) ??
- ????public?Set<Student>?getStudents()?{ ??
- ????????return?students; ??
- ????} ??
- ??
- ????public?void?setStudents(Set<Student>?students)?{ ??
- ????????this.students?=?students; ??
- ????} ??
- ??
- ??????@Override ??
- ????public?int?hashCode()?{ ??
- ????????final?int?prime?=? 31 ; ??
- ????????int?result?=? 1 ; ??
- ????????result?=?prime?*?result?+?((id?==?null)??? 0 ?:?id.hashCode()); ??
- ????????return?result; ??
- ????} ??
- ??
- ????@Override ??
- ????public?boolean?equals(Object?obj)?{ ??
- ????????if?(this?==?obj) ??
- ????????????return?true; ??
- ????????if?(obj?==?null) ??
- ????????????return?false; ??
- ????????if?(getClass()?!=?obj.getClass()) ??
- ????????????return?false; ??
- ????????Teacher?other?=?(Teacher)?obj; ??
- ????????if?(id?==?null)?{ ??
- ????????????if?(other.id?!=?null) ??
- ????????????????return?false; ??
- ????????}?else?if?(!id.equals(other.id)) ??
- ????????????return?false; ??
- ????????return?true; ??
- ????} ??
- }??
package com.taoistwar.jpa.entity.manytomany; import java.util.HashSet; import java.util.Set; import javax.persistence.CascadeType; import javax.persistence.Column; import javax.persistence.Entity; import javax.persistence.FetchType; import javax.persistence.GeneratedValue; import javax.persistence.GenerationType; import javax.persistence.Id; import javax.persistence.ManyToMany; @Entity public class Teacher { private Integer id; private String name; private Set<Student> students = new HashSet<Student>(); @Id @GeneratedValue(strategy = GenerationType.AUTO) public Integer getId() { return id; } public void setId(Integer id) { this.id = id; } @Column(nullable = false, length = 16) public String getName() { return name; } public void setName(String name) { this.name = name; } @ManyToMany(cascade = CascadeType.REFRESH, mappedBy = "teachers", fetch = FetchType.LAZY) public Set<Student> getStudents() { return students; } public void setStudents(Set<Student> students) { this.students = students; } @Override public int hashCode() { final int prime = 31; int result = 1; result = prime * result + ((id == null) ? 0 : id.hashCode()); return result; } @Override public boolean equals(Object obj) { if (this == obj) return true; if (obj == null) return false; if (getClass() != obj.getClass()) return false; Teacher other = (Teacher) obj; if (id == null) { if (other.id != null) return false; } else if (!id.equals(other.id)) return false; return true; } }
?重點(diǎn)在于:
- @ManyToMany (cascade?=?CascadeType.REFRESH,?mappedBy?=? "teachers" ,?fetch?=?FetchType.LAZY) ??
- ???? public ?Set<Student>?getStudents()?{ ??
- ???????? return ?students; ??
- ????}??
@ManyToMany(cascade = CascadeType.REFRESH, mappedBy = "teachers", fetch = FetchType.LAZY) public Set<Student> getStudents() { return students; }
?擁有mappedBy注解的實(shí)體類為關(guān)系被維護(hù)端,另外的實(shí)體類為關(guān)系維護(hù)端的。顧名思意,關(guān)系的維護(hù)端對(duì)關(guān)系(在多對(duì)多為中間關(guān)聯(lián)表)的CRUD做操作。關(guān)系的被維護(hù)端沒有該操作,不能維護(hù)關(guān)系。
測(cè)試類
- package ?com.taoistwar.jpa.entity.manytomany; ??
- ??
- import ?javax.persistence.EntityManager; ??
- import ?javax.persistence.EntityManagerFactory; ??
- import ?javax.persistence.Persistence; ??
- ??
- import ?org.junit.Test; ??
- ??
- public ? class ?ManyToMany?{ ??
- ???? @Test ??
- ???? public ? void ?save()?{ ??
- ????????EntityManagerFactory?emf?=?Persistence ??
- ????????????????.createEntityManagerFactory( "JPAPU" ); ??
- ????????EntityManager?em?=?emf.createEntityManager(); ??
- ????????em.getTransaction().begin(); ??
- ????????Student?student?=? new ?Student(); ??
- ????????student.setName( "小李" ); ??
- ????????Teacher?teacher?=? new ?Teacher(); ??
- ????????teacher.setName( "大李" ); ??
- ????????em.persist(student); ??
- ????????em.persist(teacher); ??
- ????????em.getTransaction().commit(); ??
- ????????emf.close(); ??
- ????} ??
- ???? ??
- ???? @Test ??
- ???? public ? void ?bind()?{ ??
- ????????EntityManagerFactory?emf?=?Persistence ??
- ????????????????.createEntityManagerFactory( "JPAPU" ); ??
- ????????EntityManager?em?=?emf.createEntityManager(); ??
- ????????em.getTransaction().begin(); ??
- ????????Student?student?=?em.find(Student. class ,? 1 ); ??
- ????????Teacher?teacher?=?em.find(Teacher. class ,? 1 ); ??
- ????????student.addTeacher(teacher); ??
- ????????em.persist(student); ??
- ????????em.getTransaction().commit(); ??
- ????????emf.close(); ??
- ????} ??
- ???? ??
- ???? @Test ??
- ???? public ? void ?unbind()?{ ??
- ????????EntityManagerFactory?emf?=?Persistence ??
- ????????????????.createEntityManagerFactory( "JPAPU" ); ??
- ????????EntityManager?em?=?emf.createEntityManager(); ??
- ????????em.getTransaction().begin(); ??
- ????????Student?student?=?em.find(Student. class ,? 1 ); ??
- ????????Teacher?teacher?=?em.find(Teacher. class ,? 1 ); ??
- ????????student.removeTeachers(teacher); ??
- ????????em.persist(student); ??
- ????????em.getTransaction().commit(); ??
- ????????emf.close(); ??
- ????} ??
- ???? ??
- ???? @Test ??
- ???? public ? void ?removeTeacher()?{ ??
- ????????EntityManagerFactory?emf?=?Persistence ??
- ????????????????.createEntityManagerFactory( "JPAPU" ); ??
- ????????EntityManager?em?=?emf.createEntityManager(); ??
- ????????em.getTransaction().begin(); ??
- ???????? //?關(guān)系被維護(hù)端刪除時(shí),如果中間表存在些紀(jì)錄的關(guān)聯(lián)信息,則會(huì)刪除失敗 ??
- ????????em.remove(em.getReference(Teacher. class ,? 1 )); ??
- ????????em.getTransaction().commit(); ??
- ????????emf.close(); ??
- ????} ??
- ???? ??
- ???? @Test ??
- ???? public ? void ?removeStudent()?{ ??
- ????????EntityManagerFactory?emf?=?Persistence ??
- ????????????????.createEntityManagerFactory( "JPAPU" ); ??
- ????????EntityManager?em?=?emf.createEntityManager(); ??
- ????????em.getTransaction().begin(); ??
- ???????? //?關(guān)系維護(hù)端刪除時(shí),如果中間表存在些紀(jì)錄的關(guān)聯(lián)信息,則會(huì)刪除該關(guān)聯(lián)信息 ??
- ????????em.remove(em.getReference(Student. class ,? 1 )); ??
- ????????em.getTransaction().commit(); ??
- ????????emf.close(); ??
- ????} ??
- ???? ??
- }??
轉(zhuǎn) http://taoistwar.iteye.com
更多文章、技術(shù)交流、商務(wù)合作、聯(lián)系博主
微信掃碼或搜索:z360901061
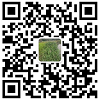
微信掃一掃加我為好友
QQ號(hào)聯(lián)系: 360901061
您的支持是博主寫作最大的動(dòng)力,如果您喜歡我的文章,感覺我的文章對(duì)您有幫助,請(qǐng)用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點(diǎn)擊下面給點(diǎn)支持吧,站長(zhǎng)非常感激您!手機(jī)微信長(zhǎng)按不能支付解決辦法:請(qǐng)將微信支付二維碼保存到相冊(cè),切換到微信,然后點(diǎn)擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對(duì)您有幫助就好】元
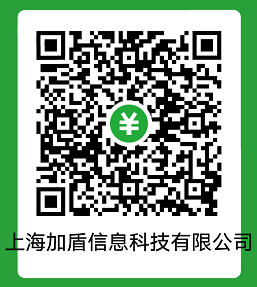