Python max內(nèi)置函數(shù)
max(iterable, *[, key, default])
max(arg1, arg2, *args[, key])
Return the largest item in an iterable or the largest of two or more arguments.
If one positional argument is provided, it should be an iterable. The largest item in the iterable is returned. If two or more positional arguments are provided, the largest of the positional arguments is returned.
There are two optional keyword-only arguments. The key argument specifies a one-argument ordering function like that used for list.sort(). The default argument specifies an object to return if the provided iterable is empty. If the iterable is empty and default is not provided, a ValueError is raised.
If multiple items are maximal, the function returns the first one encountered. This is consistent with other sort-stability preserving tools such as sorted(iterable, key=keyfunc, reverse=True)[0] and heapq.nlargest(1, iterable, key=keyfunc).
? 說明:
1. 函數(shù)功能為取傳入的多個(gè)參數(shù)中的最大值,或者傳入的可迭代對(duì)象元素中的最大值。默認(rèn)數(shù)值型參數(shù),取值大者;字符型參數(shù),取字母表排序靠后者。還可以傳入命名參數(shù)key,其為一個(gè)函數(shù),用來指定取最大值的方法。default命名參數(shù)用來指定最大值不存在時(shí)返回的默認(rèn)值。
2. 函數(shù)至少傳入兩個(gè)參數(shù),但是有只傳入一個(gè)參數(shù)的例外,此時(shí)參數(shù)必須為可迭代對(duì)象,返回的是可迭代對(duì)象中的最大元素。
>>> max(1) # 傳入1個(gè)參數(shù)報(bào)錯(cuò) Traceback (most recent call last): File "", line 1, in max(1) TypeError: 'int' object is not iterable >>> max(1,2) # 傳入2個(gè)參數(shù) 取2個(gè)中較大者 2 >>> max(1,2,3) # 傳入3個(gè)參數(shù) 取3個(gè)中較大者 3 >>> max('1234') # 傳入1個(gè)可迭代對(duì)象,取其最大元素值 '4'
3. 當(dāng)傳入?yún)?shù)為數(shù)據(jù)類型不一致時(shí),傳入的所有參數(shù)將進(jìn)行隱式數(shù)據(jù)類型轉(zhuǎn)換后再比較,如果不能進(jìn)行隱式數(shù)據(jù)類型轉(zhuǎn)換,則會(huì)報(bào)錯(cuò)。
>>> max(1,1.1,1.3E1) # 整數(shù)與浮點(diǎn)數(shù)可取最大值 13.0 >>> max(1,2,3,'3') # 數(shù)值與字符串不能取最大值 Traceback (most recent call last): File "", line 1, in max(1,2,3,'3') TypeError: unorderable types: str() > int() >>> max([1,2],[1,3]) # 列表與列表可取最大值 [1, 3] >>> max([1,2],(1,3)) # 列表與元組不能取最大值 Traceback (most recent call last): File " ", line 1, in max([1,2],(1,3)) TypeError: unorderable types: tuple() > list()
4. 當(dāng)存在多個(gè)相同的最大值時(shí),返回的是最先出現(xiàn)的那個(gè)最大值。
#定義a、b、c 3個(gè)列表 >>> a = [1,2] >>> b = [1,1] >>> c = [1,2] #查看a、b、c 的id >>> id(a) 68128320 >>> id(b) 68128680 >>> id(c) 68128240 #取最大值 >>> d = max(a,b,c) >>> id(d) 68128320 #驗(yàn)證是否最大值是否是a >>> id(a) == id(d) True
5. 默認(rèn)數(shù)值型參數(shù),取值大者;字符型參數(shù),取字母表排序靠后者;序列型參數(shù),則依次按索引位置的值進(jìn)行比較取最大者。還可以通過傳入命名參數(shù)key,指定取最大值方法。
>>> max(1,2) # 取數(shù)值大者 2 >>> max('a','b') # 取排序靠后者 'b' >>> max('ab','ac','ad') # 依次按索引比較取較大者 'ad' >>> max(-1,0) # 數(shù)值默認(rèn)去數(shù)值較大者 0 >>> max(-1,0,key = abs) # 傳入了求絕對(duì)值函數(shù),則參數(shù)都會(huì)進(jìn)行求絕對(duì)值后再取較大者 -1
6. key參數(shù)的另外一個(gè)作用是,不同類型對(duì)象本來不能比較取最大值的,傳入適當(dāng)?shù)膋ey函數(shù),變得可以比較能取最大值了。
>>> max(1,2,'3') #數(shù)值和字符串不能取最大值 Traceback (most recent call last): File "", line 1, in max(1,2,'3') TypeError: unorderable types: str() > int() >>> max(1,2,'3',key = int) # 指定key為轉(zhuǎn)換函數(shù)后,可以取最大值 '3' >>> max((1,2),[1,1]) #元組和列表不能取最大值 Traceback (most recent call last): File " ", line 1, in max((1,2),[1,1]) TypeError: unorderable types: list() > tuple() >>> max((1,2),[1,1],key = lambda x : x[1]) #指定key為返回序列索引1位置的元素后,可以取最大值 (1, 2) 復(fù)制代碼
7. 當(dāng)只傳入的一個(gè)可迭代對(duì)象時(shí),而且可迭代對(duì)象為空,則必須指定命名參數(shù)default,用來指定最大值不存在時(shí),函數(shù)返回的默認(rèn)值。
>>> max(()) #空可迭代對(duì)象不能取最大值 Traceback (most recent call last): File "", line 1, in max(()) ValueError: max() arg is an empty sequence >>> max((),default=0) #空可迭代對(duì)象,指定default參數(shù)為默認(rèn)值 0 >>> max((),0) #默認(rèn)值必須使用命名參數(shù)進(jìn)行傳參,否則將被認(rèn)為是一個(gè)比較的元素 Traceback (most recent call last): File " ", line 1, in max((),0) TypeError: unorderable types: int() > tuple()
感謝閱讀,希望能幫助到大家,謝謝大家對(duì)本站的支持!
更多文章、技術(shù)交流、商務(wù)合作、聯(lián)系博主
微信掃碼或搜索:z360901061
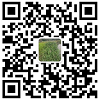
微信掃一掃加我為好友
QQ號(hào)聯(lián)系: 360901061
您的支持是博主寫作最大的動(dòng)力,如果您喜歡我的文章,感覺我的文章對(duì)您有幫助,請(qǐng)用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點(diǎn)擊下面給點(diǎn)支持吧,站長非常感激您!手機(jī)微信長按不能支付解決辦法:請(qǐng)將微信支付二維碼保存到相冊(cè),切換到微信,然后點(diǎn)擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對(duì)您有幫助就好】元
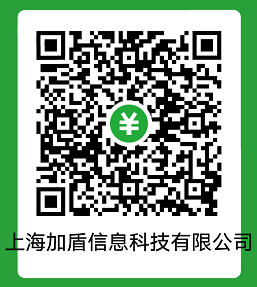