開個(gè)貼,用于記錄平時(shí)經(jīng)常碰到的Python的錯(cuò)誤同時(shí)對(duì)導(dǎo)致錯(cuò)誤的原因進(jìn)行分析,并持續(xù)更新,方便以后查詢,學(xué)習(xí)。
知識(shí)在于積累嘛!微笑
+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
錯(cuò)誤:
>>> def f(x, y):?
??? print x, y?
>>> t = ('a', 'b')?
>>> f(t)?
?
Traceback (most recent call last):?
? File "
??? f(t)?
TypeError: f() takes exactly 2 arguments (1 given)?
【錯(cuò)誤分析】不要誤以為元祖里有兩個(gè)參數(shù),將元祖?zhèn)鬟M(jìn)去就可以了,實(shí)際上元祖作為一個(gè)整體只是一個(gè)參數(shù),
實(shí)際需要兩個(gè)參數(shù),所以報(bào)錯(cuò)。必需再傳一個(gè)參數(shù)方可.
>>> f(t, 'var2')?
('a', 'b') var2?
更常用的用法: 在前面加*,代表引用元祖
>>> f(*t)?
'a', 'b'?
+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
錯(cuò)誤:
>>> def func(y=2, x):?
??? return x + y?
SyntaxError: non-default argument follows default argument?
【錯(cuò)誤分析】在C++,Python中默認(rèn)參數(shù)從左往右防止,而不是相反。這可能跟參數(shù)進(jìn)棧順序有關(guān)。
>>> def func(x, y=2):?
??? return x + y?
>>> func(1)?
3?
+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
錯(cuò)誤:
>>> D1 = {'x':1, 'y':2}?
>>> D1['x']?
1?
>>> D1['z']?
?
Traceback (most recent call last):?
? File "
??? D1['z']?
KeyError: 'z'?
【錯(cuò)誤分析】這是Python中字典鍵錯(cuò)誤的提示,如果想讓程序繼續(xù)運(yùn)行,可以用字典中的get方法,如果鍵存在,則獲取該鍵對(duì)應(yīng)的值,不存在的,返回None,也可打印提示信息.
>>> D1.get('z', 'Key Not Exist!')?
'Key Not Exist!'?
+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
錯(cuò)誤:
>>> from math import sqrt?
>>> exec "sqrt = 1"?
>>> sqrt(4)?
?
Traceback (most recent call last):?
? File "
??? sqrt(4)?
TypeError: 'int' object is not callable?
【錯(cuò)誤分析】exec語句最有用的地方在于動(dòng)態(tài)地創(chuàng)建代碼字符串,但里面存在的潛在的風(fēng)險(xiǎn),它會(huì)執(zhí)行其他地方的字符串,在CGI中更是如此!比如例子中的sqrt = 1,從而改變了當(dāng)前的命名空間,從math模塊中導(dǎo)入的sqrt不再和函數(shù)名綁定而是成為了一個(gè)整數(shù)。要避免這種情況,可以通過增加in
>>> from math import sqrt?
>>> scope = {}?
>>> exec "sqrt = 1" in scope?
>>> sqrt(4)?
2.0?
+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
錯(cuò)誤:
>>> seq = [1, 2, 3, 4]?
>>> sep = '+'?
>>> sep.join(seq)?
?
Traceback (most recent call last):?
? File "
??? sep.join(seq)?
TypeError: sequence item 0: expected string, int found?
【錯(cuò)誤分析】join是split的逆方法,是非常重要的字符串方法,但不能用來連接整數(shù)型列表,所以需要改成:
>>> seq = ['1', '2', '3', '4']?
>>> sep = '+'?
>>> sep.join(seq)?
'1+2+3+4'?
+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
錯(cuò)誤:
>>> print r'C:\Program Files\foo\bar\'?
SyntaxError: EOL while scanning string literal?
【錯(cuò)誤分析】Python中原始字符串以r開頭,里面可以放置任意原始字符,包括\,包含在字符中的\不做轉(zhuǎn)義。
但是,不能放在末尾!也就是說,最后一個(gè)字符不能是\,如果真 需要的話,可以這樣寫:
>>> print r'C:\Program Files\foo\bar' "\\"?
C:\Program Files\foo\bar\?
>>> print r'C:\Program Files\foo\bar' + "\\"?
C:\Program Files\foo\bar\?
+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
代碼:
bad = 'bad'?
?
try:?
??? raise bad?
except bad:?
??? print 'Got Bad!'?
錯(cuò)誤:
>>>??
?
Traceback (most recent call last):?
? File "D:\Learn\Python\Learn.py", line 4, in
??? raise bad?
TypeError: exceptions must be old-style classes or derived from BaseException, not str
【錯(cuò)誤分析】因所用的Python版本2.7,比較高的版本,raise觸發(fā)的異常,只能是自定義類異常,而不能是字符串。所以會(huì)報(bào)錯(cuò),字符串改為自定義類,就可以了。
class Bad(Exception):?
??? pass?
?
def raiseException():?
??? raise Bad()?
?
try:?
??? raiseException()?
except Bad:?
??? print 'Got Bad!'?
?
+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
class Super:?
??? def method(self):?
??????? print "Super's method"?
?
class Sub(Super):?
??? def method(self):?
??????? print "Sub's method"?
??????? Super.method()?
??????? print "Over..."?
?
S = Sub()?
S.method()?
執(zhí)行上面一段代碼,錯(cuò)誤如下:
>>>??
Sub's method?
?
Traceback (most recent call last):?
? File "D:\Learn\Python\test.py", line 12, in
??? S.method()?
? File "D:\Learn\Python\test.py", line 8, in method?
??? Super.method()?
TypeError: unbound method method() must be called with Super instance as first argument (got nothing instead)?
【錯(cuò)誤分析】Python中調(diào)用類的方法,必須與實(shí)例綁定,或者調(diào)用自身.
ClassName.method(x, 'Parm')
ClassName.method(self)
所以上面代碼,要調(diào)用Super類的話,只需要加個(gè)self參數(shù)即可。
class Super:?
??? def method(self):?
??????? print "Super's method"?
?
class Sub(Super):?
??? def method(self):?
??????? print "Sub's method"?
??????? Super.method(self)?
??????? print "Over..."?
?
S = Sub()?
S.method()?
?
?
#輸出結(jié)果?
>>>??
Sub's method?
Super's method?
Over...?
+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
>>> reload(sys)?
Traceback (most recent call last):?
? File "
NameError: name 'sys' is not defined?
【錯(cuò)誤分析】reload期望得到的是對(duì)象,所以該模塊必須成功導(dǎo)入。在沒導(dǎo)入模塊前,不能重載.
>>> import sys?
>>> reload(sys)?
+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
>>> def f(x, y, z):?
??? return x + y + z?
?
>>> args = (1,2,3)?
>>> print f(args)?
?
Traceback (most recent call last):?
? File "
??? print f(args)?
TypeError: f() takes exactly 3 arguments (1 given)
【錯(cuò)誤分析】args是一個(gè)元祖,如果是f(args),那么元祖是作為一個(gè)整體作為一個(gè)參數(shù)
*args,才是將元祖中的每個(gè)元素作為參數(shù)
>>> f(*args)?
6?
+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
>>> def f(a,b,c,d):?
...?? print a,b,c,d?
...?
>>> args = (1,2,3,4)?
>>> f(**args)?
Traceback (most recent call last):?
? File "
TypeError: f() argument after ** must be a mapping, not tuple?
【錯(cuò)誤分析】錯(cuò)誤原因**匹配并收集在字典中所有包含位置的參數(shù),但傳遞進(jìn)去的卻是個(gè)元祖。
所以修改傳遞參數(shù)如下:
>>> args = {'a':1,'b':2,'c':3}?
>>> args['d'] = 4?
>>> f(**args)?
1 2 3 4?
+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
【錯(cuò)誤分析】在函數(shù)hider()內(nèi)使用了內(nèi)置變量open,但根據(jù)Python作用域規(guī)則LEGB的優(yōu)先級(jí):
先是查找本地變量==》模塊內(nèi)的其他函數(shù)==》全局變量==》內(nèi)置變量,查到了即停止查找。
所以open在這里只是個(gè)字符串,不能作為打開文件來使用,所以報(bào)錯(cuò),更改變量名即可。
可以導(dǎo)入__builtin__模塊看到所有內(nèi)置變量:異常錯(cuò)誤、和內(nèi)置方法
>>> import __builtin__
>>> dir(__builtin__)
['ArithmeticError', 'AssertionError', 'AttributeError',..
? .........................................zip,filter,map]
?
++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
In [105]: T1 = (1)?
In [106]: T2 = (2,3)?
In [107]: T1 + T2?
---------------------------------------------------------------------------?
TypeError???????????????????????????????? Traceback (most recent call last)?
----> 1 T1 + T2;?
?
TypeError: unsupported operand type(s) for +: 'int' and 'tuple'?
【錯(cuò)誤分析】(1)的類型是整數(shù),所以不能與另一個(gè)元祖做合并操作,如果只有一個(gè)元素的元祖,應(yīng)該用(1,)來表示
In [108]: type(T1)?
Out[108]: int?
?
In [109]: T1 = (1,)?
In [110]: T2 = (2,3)?
In [111]: T1 + T2?
Out[111]: (1, 2, 3)?
+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
>>> hash(1,(2,[3,4]))?
?
Traceback (most recent call last):?
? File "
??? hash((1,2,(2,[3,4])))?
TypeError: unhashable type: 'list'?
【錯(cuò)誤分析】字典中的鍵必須是不可變對(duì)象,如(整數(shù),浮點(diǎn)數(shù),字符串,元祖).
可用hash()判斷某個(gè)對(duì)象是否可哈希
>>> hash('string')?
-1542666171?
但列表中元素是可變對(duì)象,所以是不可哈希的,所以會(huì)報(bào)上面的錯(cuò)誤.
如果要用列表作為字典中的鍵,最簡單的辦法是:
>>> D = {}?
>>> D[tuple([3,4])] = 5?
>>> D?
{(3, 4): 5}?
+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
>>> L = [2,1,4,3]?
>>> L.reverse().sort()?
Traceback (most recent call last):?
? File "
AttributeError: 'NoneType' object has no attribute 'sort'?
>>> L?
[3, 4, 1, 2]?
【錯(cuò)誤分析】列表屬于可變對(duì)象,其append(),sort(),reverse()會(huì)在原處修改對(duì)象,不會(huì)有返回值,
或者說返回值為空,所以要實(shí)現(xiàn)反轉(zhuǎn)并排序,不能并行操作,要分開來寫
>>> L = [2,1,4,3]?
>>> L.reverse()?
>>> L.sort()?
>>> L?
[1, 2, 3, 4]?
或者用下面的方法實(shí)現(xiàn):
In [103]: sorted(reversed([2,1,4,3]))?
Out[103]: [1, 2, 3, 4]?
+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
>>> class = 78?
SyntaxError: invalid syntax?
【錯(cuò)誤分析】class是Python保留字,Python保留字不能做變量名,可以用Class,或klass
同樣,保留字不能作為模塊名來導(dǎo)入,比如說,有個(gè)and.py,但不能將其作為模塊導(dǎo)入
>>> import and?
SyntaxError: invalid syntax?
+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
>>> f = open('D:\new\text.data','r')?
Traceback (most recent call last):?
? File "
IOError: [Errno 22] invalid mode ('r') or filename: 'D:\new\text.data'?
>>> f = open(r'D:\new\text.data','r')?
>>> f.read()?
'Very\ngood\naaaaa'?
【錯(cuò)誤分析】\n默認(rèn)為換行,\t默認(rèn)為TAB鍵.
所以在D:\目錄下找不到ew目錄下的ext.data文件,將其改為raw方式輸入即可。
+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
try:?
??? print 1 / 0?
?????
except ZeroDivisionError:?
??? print 'integer division or modulo by zero'?
?????
finally:?
??? print 'Done'?
?
else:???
??? print 'Continue Handle other part'?
報(bào)錯(cuò)如下:?
D:\>python Learn.py?
? File "Learn.py", line 11?
??? else:?
?????? ^?
SyntaxError: invalid syntax?
【錯(cuò)誤分析】錯(cuò)誤原因,else, finally執(zhí)行位置;正確的程序應(yīng)該如下:
try:?
??? print 1 / 0?
?????
except ZeroDivisionError:?
??? print 'integer division or modulo by zero'?
?
?
else:???
??? print 'Continue Handle other part'?
?????
finally:?
??? print 'Done'?
+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
>>> [x,y for x in range(2) for y in range(3)]?
? File "
??? [x,y for x in range(2) for y in range(3)]?
?????????? ^?
SyntaxError: invalid syntax?
【錯(cuò)誤分析】錯(cuò)誤原因,列表解析中,x,y必須以數(shù)組的方式列出(x,y)
>>> [(x,y) for x in range(2) for y in range(3)]?
[(0, 0), (0, 1), (0, 2), (1, 0), (1, 1), (1, 2)]?
+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
class JustCounter:?
??? __secretCount = 0?
?
??? def count(self):?
??????? self.__secretCount += 1?
??????? print 'secretCount is:', self.__secretCount?
?
count1 = JustCounter()?
?
count1.count()?
count1.count()?
?
count1.__secretCount?
報(bào)錯(cuò)如下:
>>>??
secretCount is: 1?
secretCount is: 2?
?
?
Traceback (most recent call last):?
? File "D:\Learn\Python\Learn.py", line 13, in
??? count1.__secretCount?
AttributeError: JustCounter instance has no attribute '__secretCount'?
【錯(cuò)誤分析】雙下劃線的類屬性__secretCount不可訪問,所以會(huì)報(bào)無此屬性的錯(cuò)誤.
解決辦法如下:
# 1. 可以通過其內(nèi)部成員方法訪問?
# 2. 也可以通過訪問?
ClassName._ClassName__Attr?
#或??
ClassInstance._ClassName__Attr?
#來訪問,比如:?
print count1._JustCounter__secretCount?
print JustCounter._JustCounter__secretCount??
+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
>>> print x?
Traceback (most recent call last):?
? File "
NameError: name 'x' is not defined?
>>> x = 1?
>>> print x?
1?
【錯(cuò)誤分析】Python不允許使用未賦值變量
+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
>>> t = (1,2)?
>>> t.append(3)?
Traceback (most recent call last):?
? File "
AttributeError: 'tuple' object has no attribute 'append'?
>>> t.remove(2)?
Traceback (most recent call last):?
? File "
AttributeError: 'tuple' object has no attribute 'remove'?
>>> t.pop()?
Traceback (most recent call last):?
? File "
AttributeError: 'tuple' object has no attribute 'pop'?
【錯(cuò)誤分析】屬性錯(cuò)誤,歸根到底在于元祖是不可變類型,所以沒有這幾種方法.
+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
>>> t = ()?
>>> t[0]?
Traceback (most recent call last):?
? File "
IndexError: tuple index out of range?
>>> l = []?
>>> l[0]?
Traceback (most recent call last):?
? File "
IndexError: list index out of range?
【錯(cuò)誤分析】空元祖和空列表,沒有索引為0的項(xiàng)
+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
>>> if X>Y:?
...? X,Y = 3,4?
...?? print X,Y?
? File "
??? print X,Y?
??? ^?
IndentationError: unexpected indent?
?
?
>>>?? t = (1,2,3,4)?
? File "
??? t = (1,2,3,4)?
??? ^?
IndentationError: unexpected indent?
【錯(cuò)誤分析】一般出在代碼縮進(jìn)的問題
+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
>>> f = file('1.txt')?
>>> f.readline()?
'AAAAA\n'?
>>> f.readline()?
'BBBBB\n'?
>>> f.next()?
'CCCCC\n'?
【錯(cuò)誤分析】如果文件里面沒有行了會(huì)報(bào)這種異常
>>> f.next() #?
Traceback (most recent call last):?
? File "
StopIteration
有可迭代的對(duì)象的next方法,會(huì)前進(jìn)到下一個(gè)結(jié)果,而在一系列結(jié)果的末尾時(shí),會(huì)引發(fā)StopIteration的異常.
next()方法屬于Python的魔法方法,這種方法的效果就是:逐行讀取文本文件的最佳方式就是根本不要去讀取。
取而代之的用for循環(huán)去遍歷文件,自動(dòng)調(diào)用next()去調(diào)用每一行,且不會(huì)報(bào)錯(cuò)
for line in open('test.txt','r'):?
??? print line?
+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
>>> string = 'SPAM'?
>>> a,b,c = string?
Traceback (most recent call last):?
? File "
ValueError: too many values to unpack?
【錯(cuò)誤分析】接受的變量少了,應(yīng)該是
>>> a,b,c,d = string?
>>> a,d?
('S', 'M')?
#除非用切片的方式?
>>> a,b,c = string[0],string[1],string[2:]?
>>> a,b,c?
('S', 'P', 'AM')?
或者?
>>> a,b,c = list(string[:2]) + [string[2:]]?
>>> a,b,c?
('S', 'P', 'AM')?
或者?
>>> (a,b),c = string[:2],string[2:]?
>>> a,b,c?
('S', 'P', 'AM')?
或者?
>>> ((a,b),c) = ('SP','AM')?
>>> a,b,c?
('S', 'P', 'AM')?
?
簡單點(diǎn)就是:?
>>> a,b = string[:2]?
>>> c?? = string[2:]?
>>> a,b,c?
('S', 'P', 'AM')?
+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
>>> mydic={'a':1,'b':2}?
>>> mydic['a']?
1?
>>> mydic['c']?
Traceback (most recent call last):?
? File "
KeyError: 'c'?
【錯(cuò)誤分析】當(dāng)映射到字典中的鍵不存在時(shí)候,就會(huì)觸發(fā)此類異常, 或者可以,這樣測試
>>> 'a' in mydic.keys()?
True?
>>> 'c' in mydic.keys()????????????? #用in做成員歸屬測試?
False?
>>> D.get('c','"c" is not exist!')?? #用get或獲取鍵,如不存在,會(huì)打印后面給出的錯(cuò)誤信息?
'"c" is not exist!'?
+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
File "study.py", line 3?
? return None?
? ^?
dentationError: unexpected indent?
【錯(cuò)誤分析】一般是代碼縮進(jìn)問題,TAB鍵或空格鍵不一致導(dǎo)致
+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
>>>def A():?
return A()?
>>>A() #無限循環(huán),等消耗掉所有內(nèi)存資源后,報(bào)最大遞歸深度的錯(cuò)誤???
File "
class Bird:?
??? def __init__(self):?
??????? self.hungry = True?
??? def eat(self):?
??????? if self.hungry:?
??????????? print "Ahaha..."?
??????????? self.hungry = False?
??????? else:?
??????????? print "No, Thanks!"?
該類定義鳥的基本功能吃,吃飽了就不再吃?
輸出結(jié)果:?
>>> b = Bird()?
>>> b.eat()?
Ahaha...?
>>> b.eat()?
No, Thanks!?
下面一個(gè)子類SingBird,?
class SingBird(Bird):?
??? def __init__(self):?
??????? self.sound = 'squawk'?
??? def sing(self):?
??????? print self.sound
輸出結(jié)果:?
>>> s = SingBird()?
>>> s.sing()?
squawk?
SingBird是Bird的子類,但如果調(diào)用Bird類的eat()方法時(shí),?
>>> s.eat()?
Traceback (most recent call last):?
? File "
??? s.eat()?
? File "D:\Learn\Python\Person.py", line 42, in eat?
??? if self.hungry:?
AttributeError: SingBird instance has no attribute 'hungry'?
【錯(cuò)誤分析】代碼錯(cuò)誤很清晰,SingBird中初始化代碼被重寫,但沒有任何初始化hungry的代碼
class SingBird(Bird):?
??? def __init__(self):?
??????? self.sound = 'squawk'?
??????? self.hungry = Ture #加這么一句?
??? def sing(self):?
??????? print self.sound?
+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
class Bird:?
??? def __init__(self):?
??????? self.hungry = True?
??? def eat(self):?
??????? if self.hungry:?
??????????? print "Ahaha..."?
??????????? self.hungry = False?
??????? else:?
??????????? print "No, Thanks!"?
?
class SingBird(Bird):?
??? def __init__(self):?
??????? super(SingBird,self).__init__()?
??????? self.sound = 'squawk'?
??? def sing(self):?
??????? print self.sound?
>>> sb = SingBird()?
Traceback (most recent call last):?
? File "
??? sb = SingBird()?
? File "D:\Learn\Python\Person.py", line 51, in __init__?
??? super(SingBird,self).__init__()?
TypeError: must be type, not classobj?
【錯(cuò)誤分析】在模塊首行里面加上__metaclass__=type,具體還沒搞清楚為什么要加
__metaclass__=type?
class Bird:?
??? def __init__(self):?
??????? self.hungry = True?
??? def eat(self):?
??????? if self.hungry:?
??????????? print "Ahaha..."?
??????????? self.hungry = False?
??????? else:?
??????????? print "No, Thanks!"?
?
class SingBird(Bird):?
??? def __init__(self):?
??????? super(SingBird,self).__init__()?
??????? self.sound = 'squawk'?
??? def sing(self):?
??????? print self.sound?
>>> S = SingBird()?
>>> S.?
SyntaxError: invalid syntax?
>>> S.?
SyntaxError: invalid syntax?
>>> S.eat()?
Ahaha...?
+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
>>> T?
(1, 2, 3, 4)?
>>> T[0] = 22??
Traceback (most recent call last):?
? File "
??? T[0] = 22?
TypeError: 'tuple' object does not support item assignment
【錯(cuò)誤分析】元祖不可變,所以不可以更改;可以用切片或合并的方式達(dá)到目的.
>>> T = (1,2,3,4)?
>>> (22,) + T[1:]?
(22, 2, 3, 4)?
+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
>>> X = 1;?
>>> Y = 2;?
>>> X + = Y?
? File "
??? X + = Y?
??????? ^?
SyntaxError: invalid syntax?
【錯(cuò)誤分析】增強(qiáng)行賦值不能分開來寫,必須連著寫比如說 +=, *=
>>> X += Y?
>>> X;Y?
3?
2?
更多文章、技術(shù)交流、商務(wù)合作、聯(lián)系博主
微信掃碼或搜索:z360901061
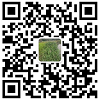
微信掃一掃加我為好友
QQ號(hào)聯(lián)系: 360901061
您的支持是博主寫作最大的動(dòng)力,如果您喜歡我的文章,感覺我的文章對(duì)您有幫助,請(qǐng)用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點(diǎn)擊下面給點(diǎn)支持吧,站長非常感激您!手機(jī)微信長按不能支付解決辦法:請(qǐng)將微信支付二維碼保存到相冊(cè),切換到微信,然后點(diǎn)擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對(duì)您有幫助就好】元
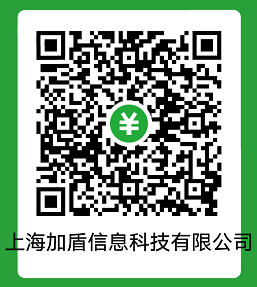