前一章介紹了python中的集中基本數(shù)據(jù)類型,本章 著重 記錄python中str字符串類型數(shù)據(jù)的應(yīng)用。str字符串主要由兩種方法,一種是方法,一種是魔術(shù)方法。由于內(nèi)容實(shí)在過(guò)于多,本章只介紹其中的方法。我會(huì)按照pycharm給的內(nèi)置方法順序(即字母排列順序) 全部 依次介紹各種方法的使用。
print(dir(str))
"""
'__add__', '__class__', '__contains__', '__delattr__',
'__dir__', '__doc__', '__eq__', '__format__', '__ge__',
'__getattribute__', '__getitem__', '__getnewargs__',
'__gt__', '__hash__', '__init__', '__init_subclass__',
'__iter__', '__le__', '__len__', '__lt__', '__mod__',
'__mul__', '__ne__', '__new__', '__reduce__', '__reduce_ex__',
'__repr__', '__rmod__', '__rmul__', '__setattr__', '__sizeof__',
'__str__', '__subclasshook__', 'capitalize', 'casefold', 'center',
'count', 'encode', 'endswith', 'expandtabs', 'find',
'format', 'format_map', 'index', 'isalnum', 'isalpha',
'isdecimal', 'isdigit', 'isidentifier', 'islower', 'isnumeric',
'isprintable', 'isspace', 'istitle', 'isupper', 'join', 'ljust',
'lower', 'lstrip', 'maketrans', 'partition', 'replace', 'rfind',
'rindex', 'rjust', 'rpartition', 'rsplit', 'rstrip', 'split',
'splitlines', 'startswith', 'strip', 'swapcase', 'title',
'translate', 'upper', 'zfill'
"""
# 前后有兩個(gè)下劃線的為魔術(shù)方法,剩下的則是我們這一節(jié)討論的方法。
首先丟出這節(jié)的目錄:
目錄
首先丟出這節(jié)的目錄:
1、capitalize()
2、casefold()
3、center()
4、count()
5、encode()
6、endswith()
7、expandtabs()
8、find()
9、format
10、format_map(maping)
11、index
12、isalnum
13、isalpha
14、isdecimal
15、isdigit
16、isidentifier
17、islower
18、isnumeric
19、isprintable
?20、isspace
21、istitle
22、isupper
23、join
24、ljust
25、lower
26、lstrip
27、maketrans
28、partition
29、replace
30、rfind
31、rindex
32、rjust
33、rpartition
34、rsplit
35、rstrip
36、split
37、splitlines
38、startswith
39、strip
40、swapcase
41、title
42、translate
43、upper
44、zfill
1、capitalize()
還是老規(guī)矩,看看pycharm對(duì)于capitalize方法給出的解釋。大致意思第一個(gè)字符就是返回一個(gè)大寫(xiě)的版本,其他的還是小寫(xiě)
def capitalize(self): # real signature unknown; restored from __doc__
"""
S.capitalize() -> str
Return a capitalized version of S, i.e. make the first character
have upper case and the rest lower case.
"""
return ""
capitalize()具體使用情況如下
a = "life is too short,I use Python"
print(a) # life is too short,I use Python
print(a.capitalize()) # Life is too short,i use python
s = "人生苦短,我用Python"
print(s) # 人生苦短,我用Python
print(s.capitalize()) # 人生苦短,我用python
s1 = "人生苦短,我用python"
print(s1) # 人生苦短,我用python
print(s1.capitalize()) # 人生苦短,我用python
由上面兩個(gè)變量可以看出。capitalize()是返回一個(gè)字符串副本,并將第一個(gè)字符大寫(xiě)。假如第一個(gè)字符不是英文,會(huì)將后面第一個(gè)英文字符進(jìn)行小寫(xiě)化,若是小寫(xiě)的存在則保持小寫(xiě)狀態(tài),不進(jìn)行改變。
2、casefold()
對(duì)于casefold()方法,pycharm給出的解釋是:
def casefold(self): # real signature unknown; restored from __doc__
"""
S.casefold() -> str
Return a version of S suitable for caseless comparisons.
"""
意思是返回經(jīng)過(guò)標(biāo)準(zhǔn)化(normalize)后的字符串,標(biāo)準(zhǔn)化類似于轉(zhuǎn)換為小寫(xiě),但更適合用于對(duì)Unicode字符串進(jìn)行不區(qū)分大小寫(xiě)的比較,和我們眾所周知的lower()方法不同,casefold可以將對(duì)于其他語(yǔ)言(非漢語(yǔ)或英文)中把大寫(xiě)轉(zhuǎn)換為小寫(xiě)。如下面的代碼塊中的 西塔 Theta 屬于希臘字符就發(fā)生了改變。
a = "PYTHON"
print(a) # PYTHON
print(a.casefold()) # python
c = "Θ"
print(c) # Θ
print(c.casefold()) #θ
3、center()
順序介紹第三個(gè)就是center,中心中央這個(gè)單詞,那么來(lái)看看pycharm給我們的對(duì)于它的解釋是什么呢?
def center(self, width, fillchar=None): # real signature unknown; restored from __doc__
"""
S.center(width[, fillchar]) -> str
Return S centered in a string of length width. Padding is
done using the specified fill character (default is a space)
"""
return ""
返回一個(gè)長(zhǎng)度為(len(string),width)的字符串。這個(gè)字符串的中間包括當(dāng)前字符串,但兩端用fillchar指定的字符(默認(rèn)是空格)填充。注意:“雙引號(hào)”可以不存在,那么就是默認(rèn)空字符串填寫(xiě)在原str前后兩端,若寫(xiě)了“雙引號(hào)”并沒(méi)有添加空格,則會(huì)出現(xiàn)報(bào)錯(cuò)提醒,如代碼塊:
# 語(yǔ)法:str.center(width , "fillchar") -> str 返回字符串
a = "hello world"
print(a) # hello world
print(a.center(20,"*")) # ****hello world*****
b = "hello world"
print(b.center(20,"")) #TypeError: The fill character must be exactly one character long
4、count()
第四個(gè)是count,這個(gè)方法還是比較常見(jiàn)的一種方法在各個(gè)編程語(yǔ)言中,統(tǒng)計(jì)某個(gè)字符在這個(gè)字符串中出現(xiàn)的次數(shù),可搜索范圍限定為string[start :end] , 其中pycharm給的解釋是:
def count(self, sub, start=None, end=None): # real signature unknown; restored from __doc__
"""
S.count(sub[, start[, end]]) -> int
Return the number of non-overlapping occurrences of substring sub in
string S[start:end]. Optional arguments start and end are
interpreted as in slice notation.
"""
return 0
那么在實(shí)際的環(huán)境中,應(yīng)用的效果:
# S.count(sub[, start[, end]]) -> int
s = "color red yellow green blue black white purple"
print(s.count("o", 0, )) #3
print(s.count("o")) # 3
print(s.count("o", ,10)) # SyntaxError: invalid syntax
將指定字符串找到后,用上count() 方法,括號(hào)里面第一個(gè)字符用“雙引號(hào)”包含在其中,中間的start為指定開(kāi)始的位置,從你指定位置開(kāi)始向后尋找,指定查找的字符,end為終止節(jié)點(diǎn),此位置后的字符串將不考慮在查找范圍內(nèi),當(dāng)然我們可以填了start后空出end的位置,那么就自動(dòng)匹配到字符串結(jié)束。假如start & end 都不存在,則是對(duì)整個(gè)字符串進(jìn)行搜索。 假如start的位置空出,只填寫(xiě)sub & end 的話,會(huì)報(bào)錯(cuò)的。
5、encode()
對(duì)于這個(gè)encode() 方法,看單詞就知道這個(gè)方法跟編碼有關(guān)。pycharm給的解釋:返回使用指定編碼和errors指定的錯(cuò)誤處理方式對(duì)字符串進(jìn)行編碼的結(jié)果。
def encode(self, encoding='utf-8', errors='strict'): # real signature unknown; restored from __doc__
"""
S.encode(encoding='utf-8', errors='strict') -> bytes
Encode S using the codec registered for encoding. Default encoding
is 'utf-8'. errors may be given to set a different error
handling scheme. Default is 'strict' meaning that encoding errors raise
a UnicodeEncodeError. Other possible values are 'ignore', 'replace' and
'xmlcharrefreplace' as well as any other name registered with
codecs.register_error that can handle UnicodeEncodeErrors.
"""
return b""
encoding參數(shù)中有utf-8、utf-16、utf-32、gbk、ASCII 碼,參數(shù)errors的值可能取值包含 ‘strict’ 、‘ignore’ 、 ‘replace’等。
# encode()語(yǔ)法:str.encode(encoding='UTF-8',errors='strict')
str = "hello world!"
print(str.encode("gbk","strict")) # b'hello world!'
print(str.encode("utf-8", "strict")) # b'hello world!'
print(str.encode("utf-16","strict"))
# b'\xff\xfeh\x00e\x00l\x00l\x00o\x00 \x00w\x00o\x00r\x00l\x00d\x00!\x00'
print(str.encode("utf-32","strict"))
# b'\xff\xfe\x00\x00h\x00\x00\x00e\x00\x00\x00l\x00\x00\x00l\x00\x00\x00o\x00\x00\x00 \x00\x00\x00w\x00\x00\x00o\x00\x00\x00r\x00\x00\x00l\x00\x00\x00d\x00\x00\x00!\x00\x00\x00'
6、endswith()
endswitch()方法是用于檢查字符串是否以suffix結(jié)尾,還可使用索引start和end來(lái)指定匹配范圍,如果以指定后綴結(jié)尾返回True,否則返回False。pycharm的使用方法 & 解釋為:
def endswith(self, suffix, start=None, end=None): # real signature unknown; restored from __doc__
"""
S.endswith(suffix[, start[, end]]) -> bool
Return True if S ends with the specified suffix, False otherwise.
With optional start, test S beginning at that position.
With optional end, stop comparing S at that position.
suffix can also be a tuple of strings to try.
"""
return False
endswith()方法語(yǔ)法:
S.endswith(suffix[, start[, end]]) -> bool
具體用法如下:
a = "hello world!"
print(a.endswith("d")) # False
print(a.endswith("!", 0)) # True
print(a.endswith("ll", 2, 4)) # True
print(a[2:4]) # ll
這個(gè)方法若用于檢查最后字符串最后一位的話,無(wú)須填寫(xiě)start & end,默認(rèn)就好了。若檢驗(yàn)中間的指定字符串用法就類似于list中的切片,用start & end 截取出指定范圍并進(jìn)行校對(duì)。
7、expandtabs()
返回將字符串中的制表符展開(kāi)為空格后的結(jié)果,可指定可選參數(shù)tabsize(默認(rèn)為8),說(shuō)通俗一點(diǎn)就是把字符串中的 tab 符號(hào)('\t')轉(zhuǎn)為空格,tab 符號(hào)('\t')默認(rèn)的空格數(shù)是 8。pycharm的使用方法 & 解釋為:
def expandtabs(self, tabsize=8): # real signature unknown; restored from __doc__
"""
S.expandtabs(tabsize=8) -> str
Return a copy of S where all tab characters are expanded using spaces.
If tabsize is not given, a tab size of 8 characters is assumed.
"""
return ""
expandtabs()方法語(yǔ)法為:
S.expandtabs(tabsize=8) -> str
具體方法如下:
str = "this\tis python ! "
print(str.expandtabs(8)) # this is python !
print(str.expandtabs(12)) # this is python !
print(str.expandtabs(16)) # this is python !
該方法返回字符串中的 tab 符號(hào)('\t')轉(zhuǎn)為空格后生成的新字符串。轉(zhuǎn)換的長(zhǎng)度隨expandtabs中參數(shù)變化而變化。
8、find()
返回找到的第一個(gè)子串sub的索引,如果沒(méi)有找到這樣的子串,就返回-1;還可將搜索范圍限制為string[start : end]
def find(self, sub, start=None, end=None): # real signature unknown; restored from __doc__
"""
S.find(sub[, start[, end]]) -> int
Return the lowest index in S where substring sub is found,
such that sub is contained within S[start:end]. Optional
arguments start and end are interpreted as in slice notation.
Return -1 on failure.
"""
return 0
具體的格式:?S.find(sub[, start[, end]]) -> int;str -- 是檢索的字符串;start -- 開(kāi)始索引,默認(rèn)為0;end -- 結(jié)束索引,默認(rèn)為字符串的長(zhǎng)度 。 那么運(yùn)行起來(lái)是一個(gè)這樣的一個(gè)情況:
# S.find(sub[, start[, end]]) -> int
a = "life is short , i use python."
print("字母i在{}位置上".format(a.find("i"))) # 字母i在1位置上
print('use在{}位置上'.format(a.find('s',14,15))) # use在-1位置上
print('use在{}位置上'.format(a.find('s',14,20))) # use在19位置上
首先我們先找的字母i,find()函數(shù)自動(dòng)會(huì)檢查到第一個(gè)符合我們指定的字符并返回位置,第二個(gè)print輸出為指定的字符串,并且指定了開(kāi)始索引和結(jié)束索引,但是在這個(gè)范圍內(nèi),并沒(méi)有找到符合的字符串,所以返回值為-1,第三個(gè)print輸出則返回的是一個(gè)匹配正確的字符串的首字母u的位置。即use中的u字母在19的位置上。
9、format
實(shí)現(xiàn)了標(biāo)準(zhǔn)的Python字符串格式設(shè)置。將字符串中用大括號(hào)分割的字段替換為相應(yīng)的參數(shù),再返回結(jié)果。
具體用法就像上面的使用的代碼塊。pycharm給的用法解釋為:
def format(self, *args, **kwargs): # known special case of str.format
"""
S.format(*args, **kwargs) -> str
Return a formatted version of S, using substitutions from args and kwargs.
The substitutions are identified by braces ('{' and '}').
"""
pass
10、format_map(maping)
類似于使用關(guān)鍵字參數(shù)調(diào)用format,只是參數(shù)是映射的方式提供的。即mapping是一個(gè)字典對(duì)象。
def format_map(self, mapping): # real signature unknown; restored from __doc__
"""
S.format_map(mapping) -> str
Return a formatted version of S, using substitutions from mapping.
The substitutions are identified by braces ('{' and '}').
"""
return ""
# S.format_map(mapping) -> str
Student = {"id":"001","name":"Bob","age":"18"}
print("My name is {name},I'm {age} old ,my id is {id}".format_map(Student))
# My name is Bob,I'm 18 old ,my id is 001
print("My name is {name},I'm {age} old ".format_map(Student))
# My name is Bob,I'm 18 old
于format不同,format是將后面的參數(shù)插入前面輸出的大括號(hào)中,參數(shù)類型可以是str、list、數(shù)字等。但是format_map()中的參數(shù)只能是鍵值對(duì)其中的value值,你可以設(shè)置了多個(gè)鍵值對(duì),不必全部輸出。選擇想輸出的value在大括號(hào)中填入其對(duì)應(yīng)的key值即可。
11、index
index(sub[, start[, end]])返回找到的第一個(gè)子串sub的索引,如果沒(méi)有找到這樣的子串,將引發(fā)valueError異常;還可將搜索范圍限制為string[start:end]。
def index(self, sub, start=None, end=None): # real signature unknown; restored from __doc__
"""
S.index(sub[, start[, end]]) -> int
Return the lowest index in S where substring sub is found,
such that sub is contained within S[start:end]. Optional
arguments start and end are interpreted as in slice notation.
Raises ValueError when the substring is not found.
"""
return 0
s = "If we just open the door a crack,the light comes pouring in."
print("open中o字母在{}位置上".format(s.index("open")))
print(s.index("open", 0, 1)) # ValueError: substring not found
index()方法和第8個(gè)提到的find()方法很相似,都是在一定長(zhǎng)度的字符串中查找規(guī)定子串的首次出現(xiàn)位置。不同點(diǎn)在于,index直接返回ValueError異常,而find返回值為-1。
12、isalnum
isalnum()檢查字符串中的字符是否 都為字母或數(shù) ,滿足則返回Ture,不滿足返回False。
def isalnum(self): # real signature unknown; restored from __doc__
"""
S.isalnum() -> bool
Return True if all characters in S are alphanumeric
and there is at least one character in S, False otherwise.
"""
return False
a = 'd1231dsa1'
print(a.isalnum()) # True
b = "$asd%gq12"
print(b.isalnum()) # False
很好理解。
13、isalpha
isalpha()檢查字符串中的字符是否 都是字母 。用法如上12,isalnum方法。
# S.isalpha() -> bool
a = "hello"
print(a.isalpha()) # True
14、isdecimal
isdecimal()檢查字符串中的字符是否都是十進(jìn)制的數(shù),用法如上12,isalnum方法。注:python中二進(jìn)制為0o開(kāi)頭,八進(jìn)制數(shù)位0x開(kāi)頭,16進(jìn)制數(shù)位0b開(kāi)頭。
# S.isdecimal() -> bool
a = oct(64)
print(a, a.isdecimal()) # 0o100 False
b = hex(64)
print(b, b.isdecimal()) # 0x40 False
c = bin(64)
print(c, c.isdecimal()) # 0b1000000 False
d = '64'
print(d, d.isdecimal()) # 64 True
15、isdigit
isdigit()檢查字符串中的字符是否都是數(shù)字。
# S.isdigit() -> bool
a = "\t2t123"
print(a.isdigit()) # False
a = "123"
print(a.isdigit()) # Ture
16、isidentifier
isidentifier()檢查字符串是否可用作Python標(biāo)識(shí)符。在python里,標(biāo)識(shí)符有字母、數(shù)字、下劃線組成。所有標(biāo)識(shí)符可以包括英文、數(shù)字以及下劃線(_),但不能以數(shù)字開(kāi)頭。標(biāo)識(shí)符可以簡(jiǎn)單的理解成為一個(gè)文件命名的規(guī)范。
# S.isidentifier() -> bool
a = "\t"
print(a.isidentifier()) # False
a = "1"
print(a.isidentifier()) # False
?
17、islower
islower()檢查字符串中 所有字母都是小寫(xiě) 的。不管字符串中有沒(méi)有其他東西,比如數(shù)字,漢字,符號(hào)等等。
# S.islower() -> bool
a = 'abc'
print(a.islower()) # True
a = 'Abc'
print(a.islower()) # False
a = "123abc"
print(a.islower()) # True
18、isnumeric
isnumeric()檢查字符串中的所有字符是否 都是數(shù)字字符 。
# S.isnumeric() -> bool
a = '\t\n'
print(a.isnumeric()) # False
a = '123'
print(a.isnumeric()) # Ture
a = '123\t'
print(a.isnumeric()) # False
a = "hello world"
print(a.isnumeric()) # False
19、isprintable
isprintable()檢查字符串中的字符是否都是可打印的。如\t 制表符 , \n換行符是無(wú)法打印的。
#S.isprintable() -> bool
a = '\t\t\t'
print(a.isprintable()) # False
a = '\t\n'
print(a.isprintable()) # False
a = '123\t'
print(a.isprintable()) # False
?20、isspace
isspace()檢查字符串中的字符都是空白字符。
#S.isspace() -> bool
a = ' '
print(a.isspace()) # True
a = '00'
print(a.isspace()) # False
a = '\t\t\t'
print(a.isspace()) # True
a = '\t\n'
print(a.isspace()) # True
21、istitle
istitle()檢查字符串中位于非字母后面的字母都是大寫(xiě)的,且其他所有字母都是小寫(xiě)的。我的理解就是檢查開(kāi)頭第一個(gè)英文字符是不是大寫(xiě)。
# S.istitle() -> bool
a = "56賦值A(chǔ)%&*@13 23A"
print(a.istitle()) # True
a = "asad1AVB"
print(a.istitle()) # False
a = "hello world!"
print(a.istitle()) # False
a = "Hello World!"
print(a.istitle()) # True
22、isupper
isupper()檢查字符串中的字母是否都是大寫(xiě)。
# S.isupper() -> bool
a = "hello world!"
print(a.isupper()) # False
a = "HELLO WORLD!"
print(a.isupper()) # True
"""
對(duì)于上面的一類以is開(kāi)頭的方法,就是檢驗(yàn)是否滿足
其后面剩余的字符的方法,如islower拆成is & lower
其中l(wèi)ower方法會(huì)在后面介紹,是一種將字符串中大寫(xiě)字符
轉(zhuǎn)換成小寫(xiě)的一個(gè)方法。
"""
23、join
join()方法,將string與sequence中所有的字符串元素合并生成的新字符串,并返回結(jié)果。兩字符串相連
def join(self, iterable): # real signature unknown; restored from __doc__
"""
S.join(iterable) -> str
Return a string which is the concatenation of the strings in the
iterable. The separator between elements is S.
"""
return ""
a = "——"
b = "abc"
print(a.join(b)) # a——b——c
24、ljust
ljust()返回一個(gè)長(zhǎng)度為max(len(string),width)的字符串,其開(kāi)頭是當(dāng)前字符串的副本,而末尾是使用fillchar指定的字符(默認(rèn)為空格)填充的。
- width -- 指定字符串長(zhǎng)度。
- fillchar -- 填充字符,默認(rèn)為空格。
def ljust(self, width, fillchar=None): # real signature unknown; restored from __doc__
"""
S.ljust(width[, fillchar]) -> str
Return S left-justified in a Unicode string of length width. Padding is
done using the specified fill character (default is a space).
"""
return ""
a = "hello"
print(a.ljust(10,'c')) # helloccccc
顯示結(jié)果為10個(gè)字符長(zhǎng)度的字符串,在原字符串的基礎(chǔ)上增加到了10(原本是5,增加了5個(gè)字符)。
25、lower
lower()將字符串中所有的字母都轉(zhuǎn)換成小寫(xiě),并返回結(jié)果。
def lower(self): # real signature unknown; restored from __doc__
"""
S.lower() -> str
Return a copy of the string S converted to lowercase.
"""
return ""
a = "HELLO WORLD!"
print(a.lower()) # hello world!
a = "hello world!"
print(a.lower()) # hello world!
26、lstrip
lstrip([chars])將字符串開(kāi)頭所有的chars(默認(rèn)為所有的空白字符,如空格、制表符和換行符)都刪除,并返回結(jié)果。刪除完后自動(dòng)縮進(jìn)定格。
def lstrip(self, chars=None): # real signature unknown; restored from __doc__
"""
S.lstrip([chars]) -> str
Return a copy of the string S with leading whitespace removed.
If chars is given and not None, remove characters in chars instead.
"""
return ""
a = "aaaaaaaaaaaaahello world!"
print(a.lstrip("a")) # hello world!
a = " hello world!"
print(a.lstrip()) # hello world!
27、maketrans
一個(gè)靜態(tài)方法,創(chuàng)建一個(gè)供translate使用的轉(zhuǎn)換表。如果只指定了參數(shù)x,它必須是從字符或序數(shù)到Unicode序數(shù)或None(用于刪除)的映射;也可使用兩個(gè)表示源字符和目標(biāo)字符串調(diào)用它;還可以提供第三個(gè)參數(shù),它指定要?jiǎng)h除的字符。
def maketrans(self, *args, **kwargs): # real signature unknown
"""
Return a translation table usable for str.translate().
If there is only one argument, it must be a dictionary mapping Unicode
ordinals (integers) or characters to Unicode ordinals, strings or None.
Character keys will be then converted to ordinals.
If there are two arguments, they must be strings of equal length, and
in the resulting dictionary, each character in x will be mapped to the
character at the same position in y. If there is a third argument, it
must be a string, whose characters will be mapped to None in the result.
"""
pass
str.maketrans(intab, outtab)
- intab -- 字符串中要替代的字符組成的字符串。
- outtab -- 相應(yīng)的映射字符的字符串。
intab = "aei"
outtab = "123"
deltab = "to"
trantab1 = str.maketrans(intab , outtab) # 創(chuàng)建字符映射轉(zhuǎn)換表
trantab2 = str.maketrans(intab,outtab,deltab) #創(chuàng)建字符映射轉(zhuǎn)換表,并刪除指定字符
test = "this is string example....wow!!!"
print(test.translate(trantab1)) # th3s 3s str3ng 2x1mpl2....wow!!!
print(test.translate(trantab2)) # h3s 3s sr3ng 2x1mpl2....ww!!!
28、partition
在字符串中搜索sep,并返回元祖(sep前面的部分,sep,sep后面的部分)。pycharm給的解釋:
def partition(self, sep): # real signature unknown; restored from __doc__
"""
S.partition(sep) -> (head, sep, tail)
Search for the separator sep in S, and return the part before it,
the separator itself, and the part after it. If the separator is not
found, return S and two empty strings.
"""
pass
a = "good"
b = a.partition('o')
print(b, type(b)) # ('g', 'o', 'od')
# 記住tuple不能修改
29、replace
將字符串中的子串old替換成new,并返回結(jié)果;還可將最大替換次數(shù)限制為max。S.replace(old, new[, count]) -> str,old為原字符串的子串,new為即將替換的子串。replace在字符串的使用中頻率是比較高的一類。
def replace(self, old, new, count=None): # real signature unknown; restored from __doc__
"""
S.replace(old, new[, count]) -> str
Return a copy of S with all occurrences of substring
old replaced by new. If the optional argument count is
given, only the first count occurrences are replaced.
"""
return ""
print("this is a test".replace('this', 'This')) # This is a test
30、rfind
返回找到的最后一個(gè)子串的索引,如果沒(méi)有找到這樣的子串,就返回-1;還可將搜索范圍限定為string[start : end]。S.rfind(sub[, start[, end]]) -> int,sub為規(guī)定子串,start為開(kāi)始索引,end為結(jié)束索引。
def rfind(self, sub, start=None, end=None): # real signature unknown; restored from __doc__
"""
S.rfind(sub[, start[, end]]) -> int
Return the highest index in S where substring sub is found,
such that sub is contained within S[start:end]. Optional
arguments start and end are interpreted as in slice notation.
Return -1 on failure.
"""
return 0
print("This is a test".rfind('s', 0, 1)) # -1
print("This is a test".rfind('s')) # 12
rfind和find的返回相同,錯(cuò)誤則返回-1,正確的找到則返回滿足要求的索引值。
不同點(diǎn):find 為第一個(gè)子串的索引,rfind為最后一個(gè)子串的索引,r即right(右,從后往前讀)
31、rindex
rindex(sub[, start[, end]])返回找到的 最后 一個(gè)子串sub的索引,如果沒(méi)有找到這樣的子串,將引發(fā)valueError異常;還可將搜索范圍限制為string[start:end]。
def rindex(self, sub, start=None, end=None): # real signature unknown; restored from __doc__
"""
S.rindex(sub[, start[, end]]) -> int
Return the highest index in S where substring sub is found,
such that sub is contained within S[start:end]. Optional
arguments start and end are interpreted as in slice notation.
Raises ValueError when the substring is not found.
"""
return 0
s = "If we just open the door a crack,the light comes pouring in."
print("t字母在{}位置上".format(s.rindex("t"))) # t字母在41位置上
print(s.index("t", 0, 1)) # ValueError: substring not found
rindex和index的返回相同,錯(cuò)誤則返回ValueError異常,正確的找到則返回滿足要求的索引值。
不同點(diǎn):rindex 為第一個(gè)子串的索引,index 為最后一個(gè)子串的索引,r即right(右,從后往前讀)
32、rjust
返回一個(gè)長(zhǎng)度為max(len(String),width)的字符串。其末尾是當(dāng)前字符串的拷貝,而開(kāi)頭是使用fillchar指定的字符(默認(rèn)為空格)填充的。
- width -- 指定字符串長(zhǎng)度。
- fillchar -- 填充字符,默認(rèn)為空格。
def rjust(self, width, fillchar=None): # real signature unknown; restored from __doc__
"""
S.rjust(width[, fillchar]) -> str
Return S right-justified in a string of length width. Padding is
done using the specified fill character (default is a space).
"""
return ""
a = "aaaahello world!aaaa"
print(a.lstrip("a")) # hello world!aaaa
a = " hello world! "
print(a.lstrip()) # hello world!
注意:ljust ——L = left? ? ,? ?rjust —— R = right
33、rpartition
與partition相同,但從右往左搜索。將字符串按照要求切成三段類似于切片。
def rpartition(self, sep): # real signature unknown; restored from __doc__
"""
S.rpartition(sep) -> (head, sep, tail)
Search for the separator sep in S, starting at the end of S, and return
the part before it, the separator itself, and the part after it. If the
separator is not found, return two empty strings and S.
"""
pass
a = "good"
b = a.rpartition('o')
print(b, type(b)) # ('go', 'o', 'd')
# 記住tuple不能修改
34、rsplit
與split相同,但指定了參數(shù)maxsplit,從右往左計(jì)算劃分次數(shù)。
def rsplit(self, sep=None, maxsplit=-1): # real signature unknown; restored from __doc__
"""
S.rsplit(sep=None, maxsplit=-1) -> list of strings
Return a list of the words in S, using sep as the
delimiter string, starting at the end of the string and
working to the front. If maxsplit is given, at most maxsplit
splits are done. If sep is not specified, any whitespace string
is a separator.
"""
return []
a = 'good\tfood\tnood\taood'
print(a.rsplit()) # ['good', 'food', 'nood', 'aood']
print(a.rsplit("\t",2)) # ['good\tfood', 'nood', 'aood']
35、rstrip
將字符串末尾所有的chars字符(默認(rèn)為所有的空白字符,如空格、制表符、換行符)都刪除,并返回結(jié)果。
def rstrip(self, chars=None): # real signature unknown; restored from __doc__
"""
S.rstrip([chars]) -> str
Return a copy of the string S with trailing whitespace removed.
If chars is given and not None, remove characters in chars instead.
"""
return ""
a = "hello world!!!!"
print(a.rstrip("!")) # hello world
a = "hello world!\t\t\t\n"
print(a.rstrip()) # hello world!
注意:rstrip為從右(right)往左右刪規(guī)定字符。strip為從左(默認(rèn))往右刪除規(guī)定字符。
36、split
split()通過(guò)指定分隔符對(duì)字符串進(jìn)行切片,如果參數(shù) num 有指定值,則分隔 num+1 個(gè)子字符串。
def split(self, sep=None, maxsplit=-1): # real signature unknown; restored from __doc__
"""
S.split(sep=None, maxsplit=-1) -> list of strings
Return a list of the words in S, using sep as the
delimiter string. If maxsplit is given, at most maxsplit
splits are done. If sep is not specified or is None, any
whitespace string is a separator and empty strings are
removed from the result.
"""
return []
a = 'good\tfood\tnood\naood'
print(a.split()) # ['good', 'food', 'nood', 'aood']
print(a.rsplit()) # ['good', 'food', 'nood', 'aood']
print(a.split("\t",2)) # ['good', 'food', 'nood\naood']
split 和rsplit的用法差不多,唯一的區(qū)別就是它們讀取字符串的順序,split默認(rèn)從左向右分開(kāi),rsplit則從右向左分開(kāi)。和strip、rstrip的區(qū)分一樣。
37、splitlines
splitlines按照行('\r', '\r\n', \n')分隔,返回一個(gè)包含各行作為元素的列表,如果參數(shù) keepends 為 False,不包含換行符,如果為 True,則保留換行符。
其中參數(shù)keepends -- 在輸出結(jié)果里是否保留換行符('\r', '\r\n', \n'),默認(rèn)為 False,不包含換行符,如果為 True,則保留換行符。
def splitlines(self, keepends=None): # real signature unknown; restored from __doc__
"""
S.splitlines([keepends]) -> list of strings
Return a list of the lines in S, breaking at line boundaries.
Line breaks are not included in the resulting list unless keepends
is given and true.
"""
return []
a = "abc\n\n\td ef\nghi \tjk"
print(a)
# abc
#
# d ef
# ghi jk
print(a.splitlines()) # ['abc', '', '\td ef', 'ghi \tjk']
b = "abc\n\n\td ef\nghi \tjk"
print(a.splitlines(True)) # ['abc\n', '\n', '\td ef\n', 'ghi \tjk']
38、startswith
startswith() 方法用于檢查字符串是否是以指定子字符串開(kāi)頭,如果是則返回 True,否則返回 False。如果參數(shù) beg 和 end 指定值,則在指定范圍內(nèi)檢查。
startswith 用法 : S.startswith(prefix[, start[, end]]) -> bool
- str -- 檢測(cè)的字符串。
- strbeg -- 可選參數(shù)用于設(shè)置字符串檢測(cè)的起始位置。
- strend -- 可選參數(shù)用于設(shè)置字符串檢測(cè)的結(jié)束位置。
def startswith(self, prefix, start=None, end=None): # real signature unknown; restored from __doc__
"""
S.startswith(prefix[, start[, end]]) -> bool
Return True if S starts with the specified prefix, False otherwise.
With optional start, test S beginning at that position.
With optional end, stop comparing S at that position.
prefix can also be a tuple of strings to try.
"""
return False
a = "Other men live to eat, while I eat to live"
print(a.startswith("Other")) # True
print(a.startswith("O")) # True
print(a.find("men")) # 6
print(a.startswith("men", 6, 9)) # True
39、strip
strip() 方法用于移除字符串頭尾指定的字符(默認(rèn)為空格或換行符)或字符序列。str.strip([chars])中chars -- 移除字符串頭尾指定的字符序列。
def strip(self, chars=None): # real signature unknown; restored from __doc__
"""
S.strip([chars]) -> str
Return a copy of the string S with leading and trailing
whitespace removed.
If chars is given and not None, remove characters in chars instead.
"""
return ""
a = "123apple123"
print(a.strip("123")) # apple
a = "123ap123ple123"
print(a.strip("123")) # ap123ple
結(jié)果顯示:strip只能刪除頭尾指定的字符串,對(duì)字符串中的字符刪除不了。
40、swapcase
sqapcase()用于對(duì)字符串的大小寫(xiě)字母進(jìn)行轉(zhuǎn)換。
def swapcase(self): # real signature unknown; restored from __doc__
"""
S.swapcase() -> str
Return a copy of S with uppercase characters converted to lowercase
and vice versa.
"""
return ""
a = "Life is short, just ues Python."
print(a.swapcase()) # lIFE IS SHORT, JUST UES pYTHON.
a = "hello WORLD!\n你好世界!"
print(a.swapcase())
# HELLO world!
# 你好世界!
41、title
title() 方法返回"標(biāo)題化"的字符串,就是說(shuō)所有單詞都是以大寫(xiě)開(kāi)始,其余字母均為小寫(xiě)(見(jiàn) istitle())。
def title(self): # real signature unknown; restored from __doc__
"""
S.title() -> str
Return a titlecased version of S, i.e. words start with title case
characters, all remaining cased characters have lower case.
"""
return ""
a = "life is short, just use Python"
print(a.title()) # Life Is Short, Just Use Python
print((a.title()).istitle()) # True
istitle是來(lái)判斷每個(gè)單詞是不是大寫(xiě)開(kāi)頭,那么我拿到后先用title轉(zhuǎn)換成每個(gè)單詞首字母大寫(xiě),再用istitle自然滿足istitle的判斷要求并輸出True。
42、translate
translate() 方法根據(jù)參數(shù)table給出的表(包含 256 個(gè)字符)轉(zhuǎn)換字符串的字符, 要過(guò)濾掉的字符放到 del 參數(shù)中。
translate()方法語(yǔ)法:str.translate(table[, deletechars]);
- table -- 翻譯表,翻譯表是通過(guò)maketrans方法轉(zhuǎn)換而來(lái)。
-
deletechars -- 字符串中要過(guò)濾的字符列表。
def translate(self, table): # real signature unknown; restored from __doc__ """ S.translate(table) -> str Return a copy of the string S in which each character has been mapped through the given translation table. The table must implement lookup/indexing via __getitem__, for instance a dictionary or list, mapping Unicode ordinals to Unicode ordinals, strings, or None. If this operation raises LookupError, the character is left untouched. Characters mapped to None are deleted. """ return ""
43、upper
upper()方法將字符串中的小寫(xiě)字母轉(zhuǎn)為大寫(xiě)字母。
def upper(self): # real signature unknown; restored from __doc__
"""
S.upper() -> str
Return a copy of S converted to uppercase.
"""
return ""
a = "hello world!"
print(a.upper()) # HELLO WORLD!
44、zfill
zfill()方法是最后一個(gè)方法,陸陸續(xù)續(xù)的寫(xiě)終于寫(xiě)到了最后一個(gè)方法。 zfill() 方法返回指定長(zhǎng)度的字符串,原字符串右對(duì)齊,前面填充0。
def zfill(self, width): # real signature unknown; restored from __doc__
"""
S.zfill(width) -> str
Pad a numeric string S with zeros on the left, to fill a field
of the specified width. The string S is never truncated.
"""
return ""
a = "Life is short , just use Python"
print(a.zfill(40))
# 000000000Life is short , just use Python
字符串的方法基本上全部介紹完畢, 下面一章介紹list列表的方法。
?
?
?
?
?
?
?
?
?
?
?
?
?
?
?
?
?
更多文章、技術(shù)交流、商務(wù)合作、聯(lián)系博主
微信掃碼或搜索:z360901061
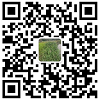
微信掃一掃加我為好友
QQ號(hào)聯(lián)系: 360901061
您的支持是博主寫(xiě)作最大的動(dòng)力,如果您喜歡我的文章,感覺(jué)我的文章對(duì)您有幫助,請(qǐng)用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點(diǎn)擊下面給點(diǎn)支持吧,站長(zhǎng)非常感激您!手機(jī)微信長(zhǎng)按不能支付解決辦法:請(qǐng)將微信支付二維碼保存到相冊(cè),切換到微信,然后點(diǎn)擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對(duì)您有幫助就好】元
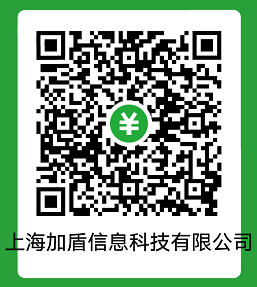