0x01 OpCode
opcode又稱為操作碼,是將python源代碼進(jìn)行編譯之后的結(jié)果,python虛擬機(jī)無法直接執(zhí)行human-readable的源代碼,因此python編譯器第一步先將源代碼進(jìn)行編譯,以此得到opcode。例如在執(zhí)行python程序時(shí)一般會(huì)先生成一個(gè)pyc文件,pyc文件就是編譯后的結(jié)果,其中含有opcode序列。
如何查看一個(gè)函數(shù)的OpCode?
def a(): if 1 == 2: print("flag{****}") print "Opcode of a():",a.__code__.co_code.encode('hex')
通過此方法我們可以得到a函數(shù)的OpCode
Opcode of a(): 6401006402006b020072140064030047486e000064000053
我們可以通過dis庫(kù)獲得相應(yīng)的解析結(jié)果。
import dis dis.dis('6401006402006b020072140064030047486e000064000053'.decode('hex'))
得到反編譯的結(jié)果
0 LOAD_CONST????????? 1 (1)
????? 3 LOAD_CONST????????? 2 (2)
????? 6 COMPARE_OP????????? 2 (==)
????? 9 POP_JUMP_IF_FALSE??? 20
???? 12 LOAD_CONST????????? 3 (3)
???? 15 LOAD_BUILD_CLASS
???? 16 YIELD_FROM????
???? 17 JUMP_FORWARD??????? 0 (to 20)
>>?? 20 LOAD_CONST????????? 0 (0)
???? 23 RETURN_VALUE
常見的字節(jié)碼指令
為了進(jìn)一步研究OpCode,我們可以對(duì)dis的disassemble_string函數(shù)進(jìn)行patch
在124行加入
print hex(op).ljust(6),
可以查看具體的字節(jié)碼。
0 LOAD_CONST?????????? 0x64?????? 1 (1)
????? 3 LOAD_CONST?????????? 0x64?????? 2 (2)
????? 6 COMPARE_OP?????????? 0x6b?????? 2 (==)
????? 9 POP_JUMP_IF_FALSE??? 0x72????? 20
???? 12 LOAD_CONST?????????? 0x64?????? 3 (3)
???? 15 LOAD_BUILD_CLASS???? 0x47?
???? 16 YIELD_FROM?????????? 0x48?
???? 17 JUMP_FORWARD???????? 0x6e?????? 0 (to 20)
>>?? 20 LOAD_CONST?????????? 0x64?????? 0 (0)
???? 23 RETURN_VALUE???????? 0x53
變量
指令名 | 操作 |
---|---|
LOAD_GLOBAL | 讀取全局變量 |
STORE_GLOBAL | 給全局變量賦值 |
LOAD_FAST | 讀取局部變量 |
STORE_FAST | 給局部變量賦值 |
LOAD_CONST | 讀取常量 |
IF
指令名 | 操作 |
---|---|
POP_JUMP_IF_FALSE | 當(dāng)條件為假的時(shí)候跳轉(zhuǎn) |
JUMP_FORWARD | 直接跳轉(zhuǎn) |
CMP_OP
cmp_op = ('<', '<=', '==', '!=', '>', '>=', 'in', 'not in', 'is','is not', 'exception match', 'BAD')
其余的指令參考OpCode源碼
0x02 利用OpCode改變程序運(yùn)行邏輯
在Python中,我們可以對(duì)任意函數(shù)的__code__參數(shù)進(jìn)行賦值,通過對(duì)其進(jìn)行賦值,我們可以改變程序運(yùn)行邏輯。
Example1
def a(): if 1 == 2: print("flag{****}")
在沙箱環(huán)境中我們需要調(diào)用這個(gè)函數(shù),但是此函數(shù)我們無法執(zhí)行到print語句。因此我們需要通過某種方法得到flag
Solution 1
我們直接獲取a.__code__.co_consts,查看所有的常量。即可知道flag
(None, 1, 2, 'flag{****}')
Solution 2
更改程序運(yùn)行邏輯
CodeType構(gòu)造函數(shù)
def __init__(self, argcount, nlocals, stacksize, flags, code, consts, names, varnames, filename, name, firstlineno, lnotab, freevars=None, cellvars=None):
上述函數(shù)其余參數(shù)均可通過__code.__.co_xxx獲得
因此我們
def a(): if 1 == 2: print("flag{****}") for name in dir(a.__code__): print name,getattr(a.__code__,name)
輸出
co_argcount 0
co_cellvars ()
co_code ddkrdGHndS
co_consts (None, 1, 2, 'flag{****}')
co_filename example1.py
co_firstlineno 1
co_flags 67
co_freevars ()
co_lnotabco_name a
co_names ()
co_nlocals 0
co_stacksize 2
co_varnames ()
構(gòu)造相應(yīng)目標(biāo)代碼
def a(): if 1 != 2: print("flag{****}") print "Opcode of a():",a.__code__.co_code.encode('hex')
得到code
6401006402006b030072140064030047486e000064000053
構(gòu)造payload
def a(): if 1 == 2: print("flag{****}") newcode = type(a.__code__) code = "6401006402006b030072140064030047486e000064000053".decode('hex') code = newcode(0,0,2,67,code,(None, 1, 2, 'flag{****}'),(),(),"xxx","a",1,"") a.__code__ = code a()
即可輸出flag
Example 2
def target(flag): def printflag(): if flag == "": print flag return printflag flag = target("flag{*******}")
這一次因?yàn)槭峭ㄟ^變量傳入?yún)?shù),我們無法通過上一次讀co_consts獲得變量。但是我們這次依舊可以通過重寫code獲得flag。
構(gòu)造替代函數(shù)
def target(flag): def printflag(): if flag != "": print flag return printflag a = target("xxx") import types code = a.__code__.co_code.encode('hex') print code
EXP
newcode = type(flag.__code__) code = "8800006401006b030072140088000047486e000064000053".decode('hex') code = newcode(0,0,2,19,code,(None, ''),(),(),"example2.py","printflag",2,"",('flag',),()) flag.__code__ = code flag()
?? python example2exp.py
8800006401006b030072140088000047486e000064000053
?? python example2.py??
flag{*******}
總結(jié)
以上就是這篇文章的全部?jī)?nèi)容了,希望本文的內(nèi)容對(duì)大家的學(xué)習(xí)或者工作具有一定的參考學(xué)習(xí)價(jià)值,謝謝大家對(duì)腳本之家的支持。
更多文章、技術(shù)交流、商務(wù)合作、聯(lián)系博主
微信掃碼或搜索:z360901061
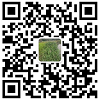
微信掃一掃加我為好友
QQ號(hào)聯(lián)系: 360901061
您的支持是博主寫作最大的動(dòng)力,如果您喜歡我的文章,感覺我的文章對(duì)您有幫助,請(qǐng)用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點(diǎn)擊下面給點(diǎn)支持吧,站長(zhǎng)非常感激您!手機(jī)微信長(zhǎng)按不能支付解決辦法:請(qǐng)將微信支付二維碼保存到相冊(cè),切換到微信,然后點(diǎn)擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對(duì)您有幫助就好】元
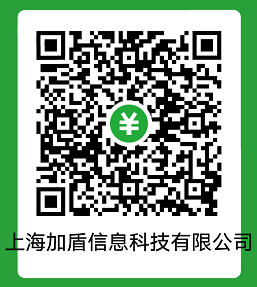