python基礎學習筆記(六)
2013-04-21 22:52 ?蟲師 閱讀( ... ) 評論( ... ) 編輯 收藏?
學到這里已經很不耐煩了,前面的數據結構什么的看起來都挺好,但還是沒法用它們做什么實際的事。
?
基本語句的更多用法
?
使用逗號輸出
>>> print ' age: ' ,25 age: 25
如果想要同時輸出文本和變量值,卻又不希望使用字符串格式化的話,那這個特性就非常有用了:
>>> name = ' chongshi ' >>> salutation = ' Mr ' >>> greeting = ' Hello. ' >>> print greeting,salutation,name Hello. Mr chongshi
?
?
模塊導入函數
從模塊導入函數的時候,可以使用
import?somemodule
或者
form?somemodule?immport??somefunction
或者
from?somemodule?import?somefunction.anotherfunction.yetanotherfunction
或者
from?somemodule?import?*??
最后一個版本只有確定自己想要從給定的模塊導入所有功能進。
如果兩個模塊都有 open 函數,可以像下面這樣使用函數:
module.open(...)
module.open(...)
當然還有別的選擇:可以在語句末尾增加一個 as 子句,在該子句后給出名字。
>>> import math as foobar # 為整個模塊提供別名 >>> foobar.sqrt(4 ) 2.0 >>> from math import sqrt as foobar # 為函數提供別名 >>> foobar(4 ) 2.0
?
賦值語句
序列解包
>>> x,y,z = 1,2,3 >>> print x,y,z 1 2 3 >>> x,y= y,x >>> print x,y,z 2 1 3
可以獲取或刪除字典中任意的鍵 - 值對,可以使用 popitem 方
>>> scoundrel ={ ' name ' : ' robin ' , ' girlfriend ' : ' marion ' } >>> key,value = scoundrel.popitem() >>> key ' name ' >>> value ' robin '
鏈式賦值
鏈式賦值是將同一個值賦給多個變量的捷徑。
>>> x = y = 42 # 同下效果: >>> y = 42 >>> x = y >>> x 42
增理賦值
>>> x = 2 >>> x += 1 # (x=x+1) >>> x *= 2 # (x=x*2) >>> x 6
?
?
控制語句
?if? 語句:
name = raw_input( ' what is your name? ' ) if name.endswith( ' chongshi ' ): print ' hello.mr.chongshi ' # 輸入 >>> what is your name?chongshi # 這里輸入錯誤將沒有任何結果,因為程序不健壯 # 輸出 hello.mr.chongshi
?
else 子句
name = raw_input( ' what is your name? ' ) if name.endswith( ' chongshi ' ): print ' hello.mr.chongshi ' else : print ' hello,strager ' # 輸入 >>> what is your name?hh # 這里輸和錯誤 # 輸出 hello,strager
?
elif? 子句
它是“ else?if ”的簡寫
num = input( ' enter a numer: ' ) if num > 0: print ' the numer is positive ' elif num < 0: print ' the number is negative ' else : print ' the nuber is zero ' # 輸入 >>> enter a numer: -1 # 輸出 the number is negative
?
嵌套
下面看一下 if 嵌套的例子( python 是以縮進表示換行的)
name = raw_input( ' what is your name? ' ) if name.endswith( ' zhangsan ' ): if name.startswith( ' mr. ' ): print ' hello.mr.zhangsan ' elif name.startswith( ' mrs. ' ): print ' hello.mrs.zhangsan ' else : print ' hello.zhangsan ' else : print ' hello.stranger '
如果輸入的是“ mr.zhangsan ”輸出第一個 print 的內容;輸入 mrs.zhangshan ,輸出第二個 print 的內容;如果輸入“ zhangsan ” , 輸出第三個 print 的內容;如果輸入的是別的什么名,則輸出的將是最后一個結果( hello.stranger )
?
斷言
如果需要確保程序中的某個條件一定為真才能讓程序正常工作的話, assert? 語句可以在程序中設置檢查點。
>>> age = 10 >>> assert 0 < age < 100 >>> age = -1 >>> assert 0 < age < 100 , ' the age must be realistic ' Traceback (most recent call last): File "" , line 1, in assert 0 < age < 100 , ' the age must be realistic ' AssertionError: the age must be realistic
?
?
循環語句
?打印 1 到 100 的數( while 循環)
x= 1 while x <= 100 : print x x += 1 # 輸出 1 2 3 4 . . 100
再看下面的例子( while 循環),用一循環保證用戶名字的輸入:
name = '' while not name: name = raw_input( ' please enter your name: ' ) print ' hello.%s! ' % name # 輸入 >>> please enter your name:huhu # 輸出 hello.huhu!
打印 1 到 100 的數( for? 循環)
for number in range(1,101 ): print number # 輸出 1 2 3 4 . . 100
是不是比 while? 循環更簡潔,但根據我們以往學習其它語言的經驗, while 的例子更容易理解。
?
一個簡單 for? 語句就能循環字典的所有鍵:
d = { ' x ' :1, ' y ' :2, ' z ' :3 } for key in d: print key, ' corresponds to ' ,d[key] # 輸出 >>> y corresponds to 2 x corresponds to 1 z corresponds to 3
?
break 語句
break? 用來結束循環,假設找 100 以內最大平方數,那么程序可以從 100 往下迭代到 0 ,步長為 -1
from math import sqrt for n in range(99,0,-1 ): root = sqrt(n) if root == int(root): print n break # 輸出 >>> 81
?
continue? 語句
continue 結束當前的迭代,“跳”到下一輪循環執行。
while True: s =raw_input( ' enter something: ' ) if s == ' quit ' : break if len(s) < 3 : continue print ' Input is of sufficient length ' # 輸入 >>> enter something:huzhiheng # 輸入長度大于3,提示信息 Input is of sufficient length enter something:ha # 輸入長度小于3,要求重輸 enter something:hah # 輸入長度等于3,提示信息 Input is of sufficient length enter something:quit # 輸入內容等于quit,結果
?
?
?
更多文章、技術交流、商務合作、聯系博主
微信掃碼或搜索:z360901061
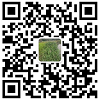
微信掃一掃加我為好友
QQ號聯系: 360901061
您的支持是博主寫作最大的動力,如果您喜歡我的文章,感覺我的文章對您有幫助,請用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點擊下面給點支持吧,站長非常感激您!手機微信長按不能支付解決辦法:請將微信支付二維碼保存到相冊,切換到微信,然后點擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對您有幫助就好】元
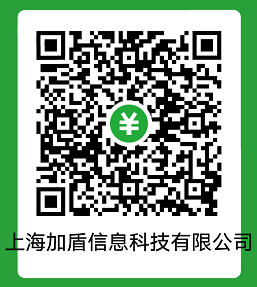