創(chuàng)建類
Python 類使用 class 關(guān)鍵字來(lái)創(chuàng)建。簡(jiǎn)單的類的聲明可以是關(guān)鍵字后緊跟類名:
class ClassName(bases):
??? 'class documentation string' #'類文檔字符串'
??? class_suite #類體
實(shí)例化
通過類名后跟一對(duì)圓括號(hào)實(shí)例化一個(gè)類
mc = MyClass() # instantiate class 初始化類
‘int()'構(gòu)造器
def __int__(self):
??? pass
注意:self類似Java的this關(guān)鍵字作用,它代碼指向自身實(shí)例的引用
類屬性
python的屬性與Java和C++等面向?qū)ο笳Z(yǔ)言不同,python的屬性即包括了數(shù)據(jù)成員還包括函數(shù)元素,通過句點(diǎn)符號(hào)來(lái)訪問.
特殊數(shù)據(jù)內(nèi)建屬性
C.
name
類C的名字(字符串)
C.
doc
類C的文檔字符串
C.
bases
類C的所有父類構(gòu)成的元組
C.
dict
類C的屬性
C.
module
類C定義所在的模塊(1.5 版本新增)
C.
class
實(shí)例C對(duì)應(yīng)的類(僅新式類中)
特殊方法內(nèi)建屬性
dir():獲得類屬性或者實(shí)例屬性名字列表.
靜態(tài)變量屬性
直接在class作用域定義
class C(object):
??? foo = 100
實(shí)例變量屬性
python的實(shí)例屬性與Java和C++等不同.在Java和C++中,實(shí)例屬性必須首先聲明/定義,而python實(shí)例屬性是動(dòng)態(tài)創(chuàng)建。設(shè)置實(shí)例的屬性可以在實(shí)例創(chuàng)建后任意時(shí)間進(jìn)行,也可以在能夠訪問實(shí)例的代碼中進(jìn)行。構(gòu)造
器init()是設(shè)置這些屬性的關(guān)鍵點(diǎn)之一。
??? def __init__(self, name, data):
??????? self.name = name
??????? self.data = "123'
注意:self類似Java的this關(guān)鍵字作用,它代碼指向自身實(shí)例的引用
方法屬性
分為實(shí)例方法和類方法.實(shí)例方法只屬于一個(gè)實(shí)例;而類方法即屬于類所有,也屬于實(shí)例所有.
實(shí)例方法
class MyClass(object):
??? def myNoActionMethod(self):
??? pass
注意:self類似Java的this關(guān)鍵字作用,它代碼指向自身實(shí)例的引用
靜態(tài)方法
靜態(tài)方法是類級(jí)別的方法,不需要實(shí)例化類就可以直接調(diào)用.有兩種方法定義
●裝飾器(常用)
??? @staticmethod??
??? def foo():
??????? print 'call static method'
●內(nèi)建函數(shù)
??? def foo():
??????? print 'call static method'
??? foo = staticmethod(foo) #靜態(tài)方法
類方法
靜態(tài)方法是類級(jí)別的方法, 與靜態(tài)方法不同的是,它必須顯示傳入cls類參數(shù);而且如果還需要調(diào)用類中其他的靜態(tài)方法,或者類方法的函數(shù), 要定義成類方法. 與靜態(tài)方法類似,也有兩種方法定義.
●裝飾器(常用)
??? @classmethod???
??? def bar(cls):
??????? print 'call class method and access static varible(staticVar): ', cls.staticVar
●內(nèi)建函數(shù)
def bar(cls):
??????? print 'call class method and access static varible(staticVar): ', cls.staticVar
??? bar = classmethod(bar)? #類方法
實(shí)例詳解
#!/usr/bin/python
#coding=utf-8
class Target(): #定義類Target
??? 'This is Target definition' #定義__doc__屬性
??? staticVar = 'v1.0'? #定義靜態(tài)變量
??? def __init__(self, name = 'default', data = 0): #定義構(gòu)造函數(shù)
??????? self.name = name??? #實(shí)例變量
??????? self.data = data??? #實(shí)例變量
??????? print "init instance"
??? def main():
??????? print "this is a test function"
??? '''
??? 可以用裝飾器定義靜態(tài)方法
??? @staticmethod??
??? def foo():
??????? print 'call static method'
??? '''
??? def foo():
??????? print 'call static method'
??? foo = staticmethod(foo) #靜態(tài)方法
??? '''
??? 可以用裝飾器定義類方法
??? @classmethod???
??? def bar(cls):
??????? print 'call class method and access static varible(staticVar): ', cls.staticVar
??? '''
??? def bar(cls):
??????? print 'call class method and access static varible(staticVar): ', cls.staticVar
??? bar = classmethod(bar)? #類方法
??? #只有調(diào)用本模塊的時(shí)候main()方法才生效
??? if __name__ == '__main__':
??????? main()
#實(shí)例化
target = Target('aaa', 123)
print 'name is: ', target.name
print 'data is: ', target.data
#打印__doc__屬性
print 'target.__doc__ is: ', target.__doc__
#打印類__dict__屬性
print 'Target.__dict__ is: ', Target.__dict__
#打印靜態(tài)變量
print 'staticVar is: ', Target.staticVar
#打印內(nèi)建函數(shù)dir()
print 'dir() is: ', dir(Target)
#調(diào)用靜態(tài)方法
Target.foo()
#調(diào)用類方法
Target.bar()
輸出
this is a test function
init instance
name is:? aaa
data is:? 123
target.__doc__ is:? This is Target definition
Target.__dict__ is:? {'__module__': '__main__', 'foo':
staticVar is:? v1.0
dir() is:? ['__doc__', '__init__', '__module__', 'bar', 'foo', 'main', 'staticVar']
call static method
call class method and access static varible(staticVar):? v1.0
更多文章、技術(shù)交流、商務(wù)合作、聯(lián)系博主
微信掃碼或搜索:z360901061
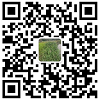
微信掃一掃加我為好友
QQ號(hào)聯(lián)系: 360901061
您的支持是博主寫作最大的動(dòng)力,如果您喜歡我的文章,感覺我的文章對(duì)您有幫助,請(qǐng)用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點(diǎn)擊下面給點(diǎn)支持吧,站長(zhǎng)非常感激您!手機(jī)微信長(zhǎng)按不能支付解決辦法:請(qǐng)將微信支付二維碼保存到相冊(cè),切換到微信,然后點(diǎn)擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對(duì)您有幫助就好】元
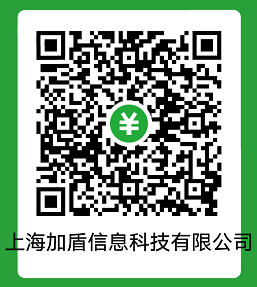