目前我們已經會使用Lock去對公共資源進行互斥訪問了,也探討了同一線程可以使用RLock去重入鎖,但是盡管如此我們只不過才處理了一些程序中簡單的同步現象,我們甚至還不能很合理的去解決使用Lock鎖帶來的死鎖問題。所以我們得學會使用更深層的解決同步問題。
Python提供的Condition對象提供了對復雜線程同步問題的支持。Condition被稱為條件變量,除了提供與Lock類似的acquire和release方法外,還提供了wait和notify方法。
使用Condition的主要方式為:線程首先acquire一個條件變量,然后判斷一些條件。如果條件不滿足則wait;如果條件滿足,進行一些處理改變條件后,通過notify方法通知其他線程,其他處于wait狀態的線程接到通知后會重新判斷條件。不斷的重復這一過程,從而解決復雜的同步問題。
下面我們通過很著名的“生產者-消費者”模型來來演示下,在Python中使用Condition實現復雜同步。
'''
Created on 2012-9-8
?
@author: walfred
@module: thread.TreadTest7
'''?
?
import threading?
import time?
?
condition = threading.Condition()?
products = 0?
?
class Producer(threading.Thread):?
??? def __init__(self):?
??????? threading.Thread.__init__(self)?
?
??? def run(self):?
??????? global condition, products?
??????? while True:?
??????????? if condition.acquire():?
??????????????? if products < 10:?
??????????????????? products += 1;?
??????????????????? print "Producer(%s):deliver one, now products:%s" %(self.name, products)?
??????????????????? condition.notify()?
??????????????? else:?
??????????????????? print "Producer(%s):already 10, stop deliver, now products:%s" %(self.name, products)?
??????????????????? condition.wait();?
??????????????? condition.release()?
??????????????? time.sleep(2)?
?
class Consumer(threading.Thread):?
??? def __init__(self):?
??????? threading.Thread.__init__(self)?
?
??? def run(self):?
??????? global condition, products?
??????? while True:?
??????????? if condition.acquire():?
??????????????? if products > 1:?
??????????????????? products -= 1?
??????????????????? print "Consumer(%s):consume one, now products:%s" %(self.name, products)?
??????????????????? condition.notify()?
??????????????? else:?
??????????????????? print "Consumer(%s):only 1, stop consume, products:%s" %(self.name, products)?
??????????????????? condition.wait();?
??????????????? condition.release()?
??????????????? time.sleep(2)?
?
if __name__ == "__main__":?
??? for p in range(0, 2):?
??????? p = Producer()?
??????? p.start()?
?
??? for c in range(0, 10):?
??????? c = Consumer()?
??????? c.start()
代碼中主要實現了生產者和消費者線程,雙方將會圍繞products來產生同步問題,首先是2個生成者生產products ,而接下來的10個消費者將會消耗products,代碼運行如下:
Producer(Thread-1):deliver one, now products:1
Producer(Thread-2):deliver one, now products:2
Consumer(Thread-3):consume one, now products:1
Consumer(Thread-4):only 1, stop consume, products:1
Consumer(Thread-5):only 1, stop consume, products:1
Consumer(Thread-6):only 1, stop consume, products:1
Consumer(Thread-7):only 1, stop consume, products:1
Consumer(Thread-8):only 1, stop consume, products:1
Consumer(Thread-10):only 1, stop consume, products:1
Consumer(Thread-9):only 1, stop consume, products:1
Consumer(Thread-12):only 1, stop consume, products:1
Consumer(Thread-11):only 1, stop consume, products:1
另外:Condition對象的構造函數可以接受一個Lock/RLock對象作為參數,如果沒有指定,則Condition對象會在內部自行創建一個RLock;除了notify方法外,Condition對象還提供了notifyAll方法,可以通知waiting池中的所有線程嘗試acquire內部鎖。由于上述機制,處于waiting狀態的線程只能通過notify方法喚醒,所以notifyAll的作用在于防止有線程永遠處于沉默狀態。
更多文章、技術交流、商務合作、聯系博主
微信掃碼或搜索:z360901061
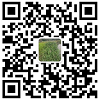
微信掃一掃加我為好友
QQ號聯系: 360901061
您的支持是博主寫作最大的動力,如果您喜歡我的文章,感覺我的文章對您有幫助,請用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點擊下面給點支持吧,站長非常感激您!手機微信長按不能支付解決辦法:請將微信支付二維碼保存到相冊,切換到微信,然后點擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對您有幫助就好】元
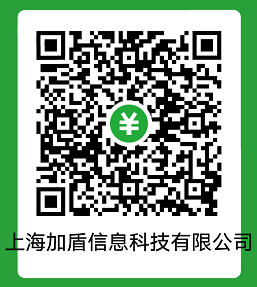