正則表達式語法及常用元字符:
正則表達式有元字符及不同組合來構成,通過巧妙的構造正則表達式可以匹配任意字符串,并完成復雜的字符串處理任務。
常用的元字符有:
其中在使用反斜線時要注意:如果以‘\'開頭的元字符與轉義字符相同,則需要使用‘\\'或者原始字符串,在字符串前面加上字符‘r'或‘R'。原始字符串可以減少用戶的輸入,主要用于‘\\',主要用于正則表達式和文件路徑字符串,如果字符串以一個‘\'結束,則需要多加一個斜線,以‘\\'結束。
\? :將下一個字符標記為一個特殊字符、或一個原義字符、或一個 向后引用、或一個八進制轉義符。例如,'n' 匹配字符 "n"。'\n' 匹配一個換行符。序列 '\\' 匹配 "\" 而 "\(" 則匹配 "("。
常用正則表達式的寫法:
‘[a-zA-Z0-9]':匹配字母或數字
‘[^abc]':匹配除abc之外的字母
‘p(ython|erl)'匹配Python和perl
‘(pattern)*'匹配0次或多次
‘(pattern)+'匹配1次或多次
‘(pattern){m,n}'匹配m_n次
‘(a|b)*c'匹配0-n次a或b后面緊跟c
‘^[a-zA-Z]{1}([a-zA-Z0-9\._]){4,19}$'匹配20個字符以字母開始
‘^(\w){6,20}$'匹配6-20個單詞字符
‘^\d{1,3}\.\d{1,3}\.\d{1,3}\.\d{1,3}$'匹配IP
‘^[a-zA-Z]+$'檢查字符中只包含英文字母
‘\w+@(\w+\.)\w+$'匹配郵箱
‘[\u4e00-\u9fa5]'匹配漢字
‘^\d{18|\d{15}$'匹配身份證
‘\d{4}-\d{1,2}-\d{1,2}'匹配時間
‘^(?=.*[a-z])(?=.*[A-Z])(?=.*\d)(?=.*[,._]).{8,}$)'判斷是否為強密碼
‘(.)\\1+'匹配任意字符的一次或多次出現
re模塊常用方法介紹:
compile(pattern[,flags])創建模式對象
search(pattern,string[,flags])在整個字符中尋找模式,返回match對象或者None
match(pattern,string[,flags])從字符串的開始處于匹配模式,返回匹配對象
findall(pattern,string[,flags]):列出匹配模式中的所有匹配項
split(pattern,string[,maxsplit=0])根據匹配模式分割字符串
sub(pat,repl,string[,count=0])將字符串中所有pat匹配項用repl替換
escape(string)將字符中的所有特殊正則表達式字符轉義
match,search,findall區別
match在字符串開頭或指定位置進行搜索,模式必須出現在開頭或指定位置;
search方法在整個字符串或指定位置進行搜索;
findall在字符串中查找所有符合正則表達式的字符串并以列表返回。
子模式與match對象
正則表達式中match和search方法匹配成功后都會返回match對象,其中match對象的主要方法有group()(返回匹配的一個或多個子模式內容),groups()(方法返回一個包含匹配所有子模式內容的元組),groupdict()(方法返回一個包含匹配所有子模式內容的字典),start()(返回子模式內容的起始位置),end()(返回子模式內容的結束位置)span()(返回包含指定子模式內容起始位置和結束位置前一個位置的元組)
代碼演示
>>> import re >>> m = re.match(r'(\w+) (\w+)','Isaac Newton,physicist') >>> m.group(0) 'Isaac Newton' >>> m.group(1) 'Isaac' >>> m.group(2) 'Newton' >>> m.group(1,2) ('Isaac', 'Newton') >>>m=re.match(r'(?P\w+)(?P \w+)','Malcolm Reynolds') >>> m.group('first_name') 'Malcolm' >>> m.group('last_name') 'Reynolds' >>> m.groups() ('Malcolm', 'Reynolds') >>> m.groupdict() {'first_name': 'Malcolm', 'last_name': 'Reynolds'}
驗證并理解子模式擴展語法的功能
>>> import re >>> exampleString = '''There should be one--and preferably only one--obvious way to do it. Although that way may not be obvious at first unless you're Dutch. Now is better than never. Although never never is often better than right now.''' >>> pattern = re.compile(r'(?<=\w\s)never(?=\s\w)') >>> matchResult = pattern.search(exampleString) >>> matchResult.span() (171, 176) >>> pattern = re.compile(r'(?<=\w\s)never') >>> matchResult = pattern.search(exampleString) >>> matchResult.span() (154, 159) >>> pattern = re.compile(r'(?:is\s)better(\sthan)') >>> matchResult = pattern.search(exampleString) >>> matchResult.span() (139, 153) >>> matchResult.group(0) 'is better than' >>> matchResult.group(1) ' than' >>> pattern = re.compile(r'\b(?i)n\w+\b') >>> index = 0 >>> while True: matchResult = pattern.search(exampleString,index) if not matchResult: break print(matchResult.group(0),':',matchResult.span(0)) index = matchResult.end(0) not : (90, 93) Now : (135, 138) never : (154, 159) never : (171, 176) never : (177, 182) now : (210, 213) >>> pattern = re.compile(r'(?>> index = 0 >>> while True: matchResult = pattern.search(exampleString,index) if not matchResult: break print(matchResult.group(0),':',matchResult.span(0)) index = matchResult.end(0) be : (13, 15) >>> exampleString[13:20] 'be one-' >>> pattern = re.compile(r'(\b\w*(?P\w+)(?P=f)\w*\b)') >>> index = 0 >>> while True: matchResult = pattern.search(exampleString,index) if not matchResult: break print(matchResult.group(0),':',matchResult.group(2)) index = matchResult.end(0)+1 unless : s better : t better : t >>> s = 'aabc abbcd abccd abbcd abcdd' >>> p = re.compile(r'(\b\w*(?P \w+)(?P=f)\w*\b)') >>> p.findall(s) [('aabc', 'a'), ('abbcd', 'b'), ('abccd', 'c'), ('abbcd', 'b'), ('abcdd', 'd')]
以上就是關于python正則表達式的相關內容,更多資料請查看腳本之家以前的文章。?
更多文章、技術交流、商務合作、聯系博主
微信掃碼或搜索:z360901061
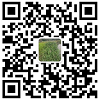
微信掃一掃加我為好友
QQ號聯系: 360901061
您的支持是博主寫作最大的動力,如果您喜歡我的文章,感覺我的文章對您有幫助,請用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點擊下面給點支持吧,站長非常感激您!手機微信長按不能支付解決辦法:請將微信支付二維碼保存到相冊,切換到微信,然后點擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對您有幫助就好】元
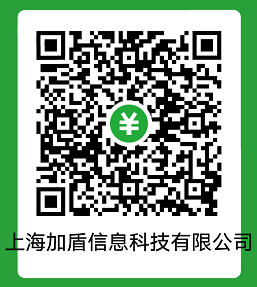