本文實(shí)例講述了Python中itertools模塊用法,分享給大家供大家參考。具體分析如下:
一般來(lái)說(shuō),itertools模塊包含創(chuàng)建有效迭代器的函數(shù),可以用各種方式對(duì)數(shù)據(jù)進(jìn)行循環(huán)操作,此模塊中的所有函數(shù)返回的迭代器都可以與for循環(huán)語(yǔ)句以及其他包含迭代器(如生成器和生成器表達(dá)式)的函數(shù)聯(lián)合使用。
chain(iter1, iter2, ..., iterN):
給出一組迭代器(iter1, iter2, ..., iterN),此函數(shù)創(chuàng)建一個(gè)新迭代器來(lái)將所有的迭代器鏈接起來(lái),返回的迭代器從iter1開(kāi)始生成項(xiàng),知道iter1被用完,然后從iter2生成項(xiàng),這一過(guò)程會(huì)持續(xù)到iterN中所有的項(xiàng)都被用完。
from itertools import chain test = chain('AB', 'CDE', 'F') for el in test: print el A B C D E F
chain.from_iterable(iterables):
一個(gè)備用鏈構(gòu)造函數(shù),其中的iterables是一個(gè)迭代變量,生成迭代序列,此操作的結(jié)果與以下生成器代碼片段生成的結(jié)果相同:
>>> def f(iterables): for x in iterables: for y in x: yield y >>> test = f('ABCDEF') >>> test.next() 'A' >>> from itertools import chain >>> test = chain.from_iterable('ABCDEF') >>> test.next() 'A'
combinations(iterable, r):
創(chuàng)建一個(gè)迭代器,返回iterable中所有長(zhǎng)度為r的子序列,返回的子序列中的項(xiàng)按輸入iterable中的順序排序:
>>> from itertools import combinations >>> test = combinations([1,2,3,4], 2) >>> for el in test: print el (1, 2) (1, 3) (1, 4) (2, 3) (2, 4) (3, 4)
count([n]):
創(chuàng)建一個(gè)迭代器,生成從n開(kāi)始的連續(xù)整數(shù),如果忽略n,則從0開(kāi)始計(jì)算(注意:此迭代器不支持長(zhǎng)整數(shù)),如果超出了sys.maxint,計(jì)數(shù)器將溢出并繼續(xù)從-sys.maxint-1開(kāi)始計(jì)算。
cycle(iterable):
創(chuàng)建一個(gè)迭代器,對(duì)iterable中的元素反復(fù)執(zhí)行循環(huán)操作,內(nèi)部會(huì)生成iterable中的元素的一個(gè)副本,此副本用于返回循環(huán)中的重復(fù)項(xiàng)。
dropwhile(predicate, iterable):
創(chuàng)建一個(gè)迭代器,只要函數(shù)predicate(item)為T(mén)rue,就丟棄iterable中的項(xiàng),如果predicate返回False,就會(huì)生成iterable中的項(xiàng)和所有后續(xù)項(xiàng)。
def dropwhile(predicate, iterable): # dropwhile(lambda x: x<5, [1,4,6,4,1]) --> 6 4 1 iterable = iter(iterable) for x in iterable: if not predicate(x): yield x break for x in iterable: yield x
groupby(iterable [,key]):
創(chuàng)建一個(gè)迭代器,對(duì)iterable生成的連續(xù)項(xiàng)進(jìn)行分組,在分組過(guò)程中會(huì)查找重復(fù)項(xiàng)。
如果iterable在多次連續(xù)迭代中生成了同一項(xiàng),則會(huì)定義一個(gè)組,如果將此函數(shù)應(yīng)用一個(gè)分類(lèi)列表,那么分組將定義該列表中的所有唯一項(xiàng),key(如果已提供)是一個(gè)函數(shù),應(yīng)用于每一項(xiàng),如果此函數(shù)存在返回值,該值將用于后續(xù)項(xiàng)而不是該項(xiàng)本身進(jìn)行比較,此函數(shù)返回的迭代器生成元素(key, group),其中key是分組的鍵值,group是迭代器,生成組成該組的所有項(xiàng)。
ifilter(predicate, iterable):
創(chuàng)建一個(gè)迭代器,僅生成iterable中predicate(item)為T(mén)rue的項(xiàng),如果predicate為None,將返回iterable中所有計(jì)算為T(mén)rue的項(xiàng)。
ifilter(lambda x: x%2, range(10)) --> 1 3 5 7 9
?
ifilterfalse(predicate, iterable):
創(chuàng)建一個(gè)迭代器,僅生成iterable中predicate(item)為False的項(xiàng),如果predicate為None,則返回iterable中所有計(jì)算為False的項(xiàng)。
ifilterfalse(lambda x: x%2, range(10)) --> 0 2 4 6 8?
imap(function, iter1, iter2, iter3, ..., iterN)
創(chuàng)建一個(gè)迭代器,生成項(xiàng)function(i1, i2, ..., iN),其中i1,i2...iN分別來(lái)自迭代器iter1,iter2 ... iterN,如果function為None,則返回(i1, i2, ..., iN)形式的元組,只要提供的一個(gè)迭代器不再生成值,迭代就會(huì)停止。
>>> from itertools import * >>> d = imap(pow, (2,3,10), (5,2,3)) >>> for i in d: print i 32 9 1000 #### >>> d = imap(pow, (2,3,10), (5,2)) >>> for i in d: print i 32 9 #### >>> d = imap(None, (2,3,10), (5,2)) >>> for i in d : print i (2, 5) (3, 2)
islice(iterable, [start, ] stop [, step]):
創(chuàng)建一個(gè)迭代器,生成項(xiàng)的方式類(lèi)似于切片返回值: iterable[start : stop : step],將跳過(guò)前start個(gè)項(xiàng),迭代在stop所指定的位置停止,step指定用于跳過(guò)項(xiàng)的步幅。與切片不同,負(fù)值不會(huì)用于任何start,stop和step,如果省略了start,迭代將從0開(kāi)始,如果省略了step,步幅將采用1.
def islice(iterable, *args): # islice('ABCDEFG', 2) --> A B # islice('ABCDEFG', 2, 4) --> C D # islice('ABCDEFG', 2, None) --> C D E F G # islice('ABCDEFG', 0, None, 2) --> A C E G s = slice(*args) it = iter(xrange(s.start or 0, s.stop or sys.maxint, s.step or 1)) nexti = next(it) for i, element in enumerate(iterable): if i == nexti: yield element nexti = next(it) #If start is None, then iteration starts at zero. If step is None, then the step defaults to one. #Changed in version 2.5: accept None values for default start and step.
izip(iter1, iter2, ... iterN):
創(chuàng)建一個(gè)迭代器,生成元組(i1, i2, ... iN),其中i1,i2 ... iN 分別來(lái)自迭代器iter1,iter2 ... iterN,只要提供的某個(gè)迭代器不再生成值,迭代就會(huì)停止,此函數(shù)生成的值與內(nèi)置的zip()函數(shù)相同。
def izip(*iterables): # izip('ABCD', 'xy') --> Ax By iterables = map(iter, iterables) while iterables: yield tuple(map(next, iterables))
izip_longest(iter1, iter2, ... iterN, [fillvalue=None]):
與izip()相同,但是迭代過(guò)程會(huì)持續(xù)到所有輸入迭代變量iter1,iter2等都耗盡為止,如果沒(méi)有使用fillvalue關(guān)鍵字參數(shù)指定不同的值,則使用None來(lái)填充已經(jīng)使用的迭代變量的值。
def izip_longest(*args, **kwds): # izip_longest('ABCD', 'xy', fillvalue='-') --> Ax By C- D- fillvalue = kwds.get('fillvalue') def sentinel(counter = ([fillvalue]*(len(args)-1)).pop): yield counter() # yields the fillvalue, or raises IndexError fillers = repeat(fillvalue) iters = [chain(it, sentinel(), fillers) for it in args] try: for tup in izip(*iters): yield tup except IndexError: pass
permutations(iterable [,r]):
創(chuàng)建一個(gè)迭代器,返回iterable中所有長(zhǎng)度為r的項(xiàng)目序列,如果省略了r,那么序列的長(zhǎng)度與iterable中的項(xiàng)目數(shù)量相同:
def permutations(iterable, r=None): # permutations('ABCD', 2) --> AB AC AD BA BC BD CA CB CD DA DB DC # permutations(range(3)) --> 012 021 102 120 201 210 pool = tuple(iterable) n = len(pool) r = n if r is None else r if r > n: return indices = range(n) cycles = range(n, n-r, -1) yield tuple(pool[i] for i in indices[:r]) while n: for i in reversed(range(r)): cycles[i] -= 1 if cycles[i] == 0: indices[i:] = indices[i+1:] + indices[i:i+1] cycles[i] = n - i else: j = cycles[i] indices[i], indices[-j] = indices[-j], indices[i] yield tuple(pool[i] for i in indices[:r]) break else: return
product(iter1, iter2, ... iterN, [repeat=1]):
創(chuàng)建一個(gè)迭代器,生成表示item1,item2等中的項(xiàng)目的笛卡爾積的元組,repeat是一個(gè)關(guān)鍵字參數(shù),指定重復(fù)生成序列的次數(shù)。
def product(*args, **kwds): # product('ABCD', 'xy') --> Ax Ay Bx By Cx Cy Dx Dy # product(range(2), repeat=3) --> 000 001 010 011 100 101 110 111 pools = map(tuple, args) * kwds.get('repeat', 1) result = [[]] for pool in pools: result = [x+[y] for x in result for y in pool] for prod in result: yield tuple(prod)
repeat(object [,times]):
創(chuàng)建一個(gè)迭代器,重復(fù)生成object,times(如果已提供)指定重復(fù)計(jì)數(shù),如果未提供times,將無(wú)止盡返回該對(duì)象。
def repeat(object, times=None): # repeat(10, 3) --> 10 10 10 if times is None: while True: yield object else: for i in xrange(times): yield object
starmap(func [, iterable]):
創(chuàng)建一個(gè)迭代器,生成值func(*item),其中item來(lái)自iterable,只有當(dāng)iterable生成的項(xiàng)適用于這種調(diào)用函數(shù)的方式時(shí),此函數(shù)才有效。
def starmap(function, iterable): # starmap(pow, [(2,5), (3,2), (10,3)]) --> 32 9 1000 for args in iterable: yield function(*args)
takewhile(predicate [, iterable]):
創(chuàng)建一個(gè)迭代器,生成iterable中predicate(item)為T(mén)rue的項(xiàng),只要predicate計(jì)算為False,迭代就會(huì)立即停止。
def takewhile(predicate, iterable): # takewhile(lambda x: x<5, [1,4,6,4,1]) --> 1 4 for x in iterable: if predicate(x): yield x else: break
tee(iterable [, n]):
從iterable創(chuàng)建n個(gè)獨(dú)立的迭代器,創(chuàng)建的迭代器以n元組的形式返回,n的默認(rèn)值為2,此函數(shù)適用于任何可迭代的對(duì)象,但是,為了克隆原始迭代器,生成的項(xiàng)會(huì)被緩存,并在所有新創(chuàng)建的迭代器中使用,一定要注意,不要在調(diào)用tee()之后使用原始迭代器iterable,否則緩存機(jī)制可能無(wú)法正確工作。
def tee(iterable, n=2): it = iter(iterable) deques = [collections.deque() for i in range(n)] def gen(mydeque): while True: if not mydeque: # when the local deque is empty newval = next(it) # fetch a new value and for d in deques: # load it to all the deques d.append(newval) yield mydeque.popleft() return tuple(gen(d) for d in deques) #Once tee() has made a split, the original iterable should not be used anywhere else; otherwise, the iterable could get advanced without the tee objects being informed. #This itertool may require significant auxiliary storage (depending on how much temporary data needs to be stored). In general, if one iterator uses most or all of the data before another iterator starts, it is faster to use list() instead of tee().
相信本文所述對(duì)大家Python程序設(shè)計(jì)的學(xué)習(xí)有一定的借鑒價(jià)值。
更多文章、技術(shù)交流、商務(wù)合作、聯(lián)系博主
微信掃碼或搜索:z360901061
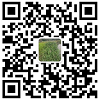
微信掃一掃加我為好友
QQ號(hào)聯(lián)系: 360901061
您的支持是博主寫(xiě)作最大的動(dòng)力,如果您喜歡我的文章,感覺(jué)我的文章對(duì)您有幫助,請(qǐng)用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點(diǎn)擊下面給點(diǎn)支持吧,站長(zhǎng)非常感激您!手機(jī)微信長(zhǎng)按不能支付解決辦法:請(qǐng)將微信支付二維碼保存到相冊(cè),切換到微信,然后點(diǎn)擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對(duì)您有幫助就好】元
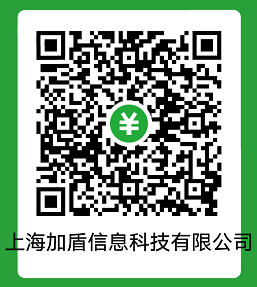