所有工具類
在日常開發(fā)中,我們經(jīng)常需要通過http協(xié)議去調(diào)用網(wǎng)絡(luò)內(nèi)容,雖然java自身提供了net相關(guān)工具包,但是其靈活性和功能總是不如人意,于是有人專門搞出一個httpclient類庫,來方便進(jìn)行Http操作。對于httpcore的源碼研究,我們可能并沒有達(dá)到這種層次,在日常開發(fā)中也只是需要的時候,在網(wǎng)上百度一下,然后進(jìn)行調(diào)用就行。在項(xiàng)目中對于這個工具類庫也許沒有進(jìn)行很好的封裝。在哪里使用就寫在哪些,很多地方用到,就在多個地方寫。反正是復(fù)制粘貼,很方便,但是這樣就會導(dǎo)致項(xiàng)目中代碼冗余。所以這里簡單的對httpcient的簡單操作封裝成一個工具類,統(tǒng)一放在項(xiàng)目的工具包中,在使用的時候直接從工具包中調(diào)用,不需要寫冗余代碼。
HttpClientUtil
package zj.http.util; import org.apache.commons.httpclient.Header; import org.apache.commons.httpclient.HttpClient; import org.apache.commons.httpclient.methods.GetMethod; import org.apache.commons.httpclient.methods.PostMethod; import org.apache.commons.httpclient.methods.StringRequestEntity; import org.apache.log4j.Logger; import zj.common.exception.ServiceException; import zj.http.bean.ICallBackMethod; /** * httpClient工具類 * * @version 1.00 (2014.09.15) * @author SHNKCS 張軍 {@link <a target=_blank href="http://m.eyofj.com">張軍個人網(wǎng)站</a> <a target=_blank href="http://user.qzone.qq.com/360901061/">張軍QQ空間</a>} */ public class HttpClientUtil { private static transient final Logger logger = Logger.getLogger(HttpClientUtil.class); /** * get請求 * * @param url * 請求地址 * @return 響應(yīng)數(shù)據(jù) * @throws Exception */ public static String get(String url) { return get(url, null); } /** * get請求 * * @param url * 請求地址 * @param callBack * 設(shè)置參數(shù)s * @return 響應(yīng)數(shù)據(jù) * @throws Exception */ public static String get(String url, ICallBackMethod callBack) { String resultString = null; HttpClient httpClient = new HttpClient(); GetMethod method = new GetMethod(url); try { // method.setRequestHeader(new Header("", "")); if (callBack != null) { callBack.setMethod(method); } int resultCode = httpClient.executeMethod(method); if (resultCode == 200) { resultString = method.getResponseBodyAsString(); } logger.debug("調(diào)用方法返回結(jié)果[" + resultCode + "]:" + resultString); return resultString; } catch (Exception e) { throw new ServiceException(e); } finally { if (method != null) { try { method.releaseConnection(); } catch (Exception e2) { } } if (httpClient != null) { // 上面的httpPost.releaseConnection()只是把連接歸還client,沒有關(guān)閉 // 加上下面這一句,才真正把不用的連接關(guān)閉 // 如果不手動關(guān)閉連接,每個連接都是保持180秒后才會自動斷開,會占用大量的連接。 try { httpClient.getHttpConnectionManager().closeIdleConnections(0); } catch (Exception e) { logger.info(e.getMessage(), e); } } // try { // method.releaseConnection(); // ((SimpleHttpConnectionManager) httpClient.getHttpConnectionManager()).shutdown(); // } catch (Exception e) { // e.printStackTrace(); // } } } /** * XML.post請求 * * @param url * 請求地址 * @param content * 請求內(nèi)容 * @return 響應(yīng)數(shù)據(jù) * @throws Exception */ public static String postXML(String url, String content) { return post(url, content, "application/xml", "UTF-8"); } /** * JSON.post請求 * * @param url * 請求地址 * @param content * 請求內(nèi)容 * @return 響應(yīng)數(shù)據(jù) * @throws Exception */ public static String postJSON(String url, String content) { return post(url, content, "application/json", "UTF-8"); } /** * post請求 * * @param url * 請求地址 * @param content * 請求內(nèi)容 * @param contentType * 請求類型 * @see contentType值說明 * @see application/x-www-form-urlencoded:窗體數(shù)據(jù)被編碼為名稱/值對。這是標(biāo)準(zhǔn)的編碼格式。這是默認(rèn)的方式 * @see multipart/form-data:窗體數(shù)據(jù)被編碼為一條消息,頁上的每個控件對應(yīng)消息中的一個部分。二進(jìn)制數(shù)據(jù)傳輸方式,主要用于上傳文件 * @see text/html * @see text/xml * @param charset * 請求編碼 * @return 響應(yīng)數(shù)據(jù) * @throws Exception */ public static String post(String url, String content, String contentType, String charset) { return post(url, content, contentType, charset, null); } /** * post請求 * * @param url * 請求地址 * @param content * 請求內(nèi)容 * @see application/x-www-form-urlencoded:窗體數(shù)據(jù)被編碼為名稱/值對。這是標(biāo)準(zhǔn)的編碼格式。這是默認(rèn)的方式 * @see multipart/form-data:窗體數(shù)據(jù)被編碼為一條消息,頁上的每個控件對應(yīng)消息中的一個部分。二進(jìn)制數(shù)據(jù)傳輸方式,主要用于上傳文件 * @see text/html * @see text/xml * @param callBack * 設(shè)置參數(shù) * @return 響應(yīng)數(shù)據(jù) * @throws Exception */ public static String postJSON(String url, String content, ICallBackMethod callBack) { return post(url, content, "application/json", "UTF-8", callBack); } /** * post請求 * * @param url * 請求地址 * @param content * 請求內(nèi)容 * @param contentType * 請求類型 * @see contentType值說明 * @see application/x-www-form-urlencoded:窗體數(shù)據(jù)被編碼為名稱/值對。這是標(biāo)準(zhǔn)的編碼格式。這是默認(rèn)的方式 * @see multipart/form-data:窗體數(shù)據(jù)被編碼為一條消息,頁上的每個控件對應(yīng)消息中的一個部分。二進(jìn)制數(shù)據(jù)傳輸方式,主要用于上傳文件 * @see text/html * @see text/xml * @param charset * 請求編碼 * @param callBack * 設(shè)置參數(shù) * @return 響應(yīng)數(shù)據(jù) * @throws Exception */ public static String post(String url, String content, String contentType, String charset, ICallBackMethod callBack) { String resultString = null; HttpClient httpClient = new HttpClient(); PostMethod method = new PostMethod(url); try { if (callBack == null) { // 默認(rèn)設(shè)置請求類型 method.setRequestHeader(new Header("Content-Type", contentType)); } else { callBack.setMethod(method); } // method.getParams().setParameter(HttpMethodParams.HTTP_CONTENT_CHARSET, ""); // 禁用重試 // DefaultHttpMethodRetryHandler retryhandler = new DefaultHttpMethodRetryHandler(0, false); // method.getParams().setParameter(HttpMethodParams.RETRY_HANDLER, retryhandler);// 重試次數(shù)0次 // httpClient.setParams(new HttpClientParams() { // private static final long serialVersionUID = 1458469810048748607L; // { // // setSoTimeout(uploadTimeOut); // // 連接超時時間 // setSoTimeout(120000); // } // }); // contentType值說明 // application/x-www-form-urlencoded:窗體數(shù)據(jù)被編碼為名稱/值對。這是標(biāo)準(zhǔn)的編碼格式。這是默認(rèn)的方式 // multipart/form-data:窗體數(shù)據(jù)被編碼為一條消息,頁上的每個控件對應(yīng)消息中的一個部分。二進(jìn)制數(shù)據(jù)傳輸方式,主要用于上傳文件 // text/plain:窗體數(shù)據(jù)以純文本形式進(jìn)行編碼,其中不含任何控件或格式字符。 method.setRequestEntity(new StringRequestEntity(content, contentType, charset)); int resultCode = httpClient.executeMethod(method); if (resultCode == 200) { resultString = method.getResponseBodyAsString(); } logger.debug("調(diào)用方法返回結(jié)果[" + resultCode + "]:" + resultString); return resultString; } catch (Exception e) { throw new ServiceException(e); } finally { if (method != null) { try { method.releaseConnection(); } catch (Exception e2) { } } if (httpClient != null) { // 上面的httpPost.releaseConnection()只是把連接歸還client,沒有關(guān)閉 // 加上下面這一句,才真正把不用的連接關(guān)閉 // 如果不手動關(guān)閉連接,每個連接都是保持180秒后才會自動斷開,會占用大量的連接。 try { httpClient.getHttpConnectionManager().closeIdleConnections(0); } catch (Exception e) { logger.info(e.getMessage(), e); } } // try { // ((SimpleHttpConnectionManager) httpClient.getHttpConnectionManager()).shutdown(); // } catch (Exception e) { // e.printStackTrace(); // } } } }
ICallBackMethod
package zj.http.bean; import org.apache.commons.httpclient.HttpMethodBase; /** * httpClient工具類回調(diào) * * @version 1.00 (2014.09.15) * @author SHNKCS 張軍 {@link <a target=_blank href="http://m.eyofj.com">張軍個人網(wǎng)站</a> <a target=_blank href="http://user.qzone.qq.com/360901061/">張軍QQ空間</a>} */ public interface ICallBackMethod { /** * 設(shè)置方法參數(shù) * * @param method */ public void setMethod(HttpMethodBase method); }
CallBackMethod
package zj.http.bean; import org.apache.commons.httpclient.HttpMethodBase; /** * httpClient工具類回調(diào) * * @version 1.00 (2014.09.15) * @author SHNKCS 張軍 {@link <a target=_blank href="http://m.eyofj.com">張軍個人網(wǎng)站</a> <a target=_blank href="http://user.qzone.qq.com/360901061/">張軍QQ空間</a>} */ public class CallBackMethod implements ICallBackMethod{ @Override public void setMethod(HttpMethodBase method) { } }
測試類
@Test public void get測試() throws Exception { try { String newUrl = "http://www.baidu.com"; String r = HttpClientUtil.get(newUrl); System.out.println(r); } catch (Exception e) { e.printStackTrace(); } } @Test public void post測試() throws Exception { try { Map<String, Object> jsonMap = new HashMap<String, Object>(); jsonMap.put("name", "8779001"); jsonMap.put("password", "123"); String json = JSON.toJSONString(jsonMap); String r = HttpClientUtil.postJSON("https://agent.ybagent9.com/login", json); System.out.println(r); } catch (Exception e) { e.printStackTrace(); } }
本文為張軍原創(chuàng)文章,轉(zhuǎn)載無需和我聯(lián)系,但請注明來自張軍的軍軍小站,個人博客http://m.eyofj.com
更多文章、技術(shù)交流、商務(wù)合作、聯(lián)系博主
微信掃碼或搜索:z360901061
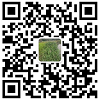
微信掃一掃加我為好友
QQ號聯(lián)系: 360901061
您的支持是博主寫作最大的動力,如果您喜歡我的文章,感覺我的文章對您有幫助,請用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點(diǎn)擊下面給點(diǎn)支持吧,站長非常感激您!手機(jī)微信長按不能支付解決辦法:請將微信支付二維碼保存到相冊,切換到微信,然后點(diǎn)擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對您有幫助就好】元
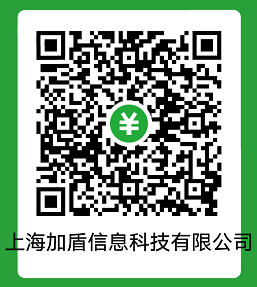