全文摘自http://www.cnblogs.com/g1mist/p/3227290.html,很好的一個實例。
反射提供了封裝程序集、模塊和類型的對象。您可以使用反射動態地創建類型的實例,將類型綁定到現有對象,或從現有對象中獲取類型。然后,可以調用類型的方法或訪問其字段和屬性。
1.先建立實體類
用戶實體類:
1
2
3
4
5
6
7
8
9
|
public
class
User
????
{
????????
public
int
id {
get
;
set
; }
????????
public
string
UserName {
get
;
set
; }
????????
public
string
Password {
get
;
set
; }
????????
public
int
Age {
get
;
set
; }
????????
public
string
PhoneNumber {
get
;
set
; }
????????
public
string
Address {
get
;
set
; }
????
}
|
書籍實體類:
1
2
3
4
5
6
7
8
|
public
class
Book
???
{
???????
public
int
id {
set
;
get
; }
???????
public
string
BookName {
get
;
set
; }
???????
public
string
ISBN {
set
;
get
; }
???????
public
string
Author {
set
;
get
; }
???????
public
double
Price {
set
;
get
; }
???
}
|
2.通過反射技術來生成Insert語句(舉個例子而已,只生成Insert語句)
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
|
??
/// <summary>
??
/// 泛型方法,反射生成SQLInsert語句
/// </summary>
??
/// <typeparam name="T">實體類型</typeparam>
??
/// <param name="entity">實體對象</param>
??
/// <returns></returns>
??
public
string
CreateInsertSQL<T>(T entity)
??
{
??????
//1.先獲取實體的類型描述
??????
Type type = entity.GetType();
??????
//2.獲得實體的屬性集合
??????
PropertyInfo[] props = type.GetProperties();
?
??????
//實例化一個StringBuilder做字符串的拼接
??
StringBuilder sb =
new
StringBuilder();
?
??????
sb.Append(
"insert into "
+ type.Name +
" ("
);
?
??????
//3.遍歷實體的屬性集合
??????
foreach
(PropertyInfo prop
in
props)
??????
{
??????????
//4.將屬性的名字加入到字符串中
?????
sb.Append(prop.Name +
","
);
??????
}
??????
//**去掉最后一個逗號
???
sb.Remove(sb.Length - 1, 1);
??????
sb.Append(
" ) values("
);
?
??????
//5.再次遍歷,形成參數列表"(@xx,@xx@xx)"的形式
??????
foreach
(PropertyInfo prop
in
props)
??????
{
??????????
sb.Append(
"@"
+ prop.Name +
","
);
??????
}
??????
//**去掉最后一個逗號
??????
sb.Remove(sb.Length - 1, 1);
??????
sb.Append(
")"
);
?
??????
return
sb.ToString();
??
}
|
3.測試
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
|
class
Program
????
{
????????
static
void
Main(
string
[] args)
????????
{
//調用ReflationCreateSQL類中的CreateInsertSQL方法??????????
????????
string
sql =
new
ReflationCreateSQL().CreateInsertSQL(
new
User());
????????????
string
sql1 =
new
ReflationCreateSQL().CreateInsertSQL(
new
Book());
?
????????????
Console.WriteLine(sql.ToString());
????????????
Console.WriteLine(sql1.ToString());
?
????????????
Console.WriteLine(
"Press any key to continue . . ."
);
????????????
Console.ReadLine();
????????
}
????
}
|
結果:
但是,我們發現id是主鍵,假設id是自增長的,我們生成的SQL(Insert)語句中就不應該有id,在這里我用自定義Attribute的方法來解決這個問題。
4.先新建一個類,繼承Attribute類
1
2
3
4
|
public
class
KEYAttribute : Attribute
{
?
}
|
這個類僅此而已就夠了。
5.在實體類中的id這個字段上加上[KEY]標記
1
2
3
4
5
6
7
8
9
|
public
class
Book
????
{
????????
[KEY]
????????
public
int
id {
set
;
get
; }
????????
public
string
BookName {
get
;
set
; }
????????
public
string
ISBN {
set
;
get
; }
????????
public
string
Author {
set
;
get
; }
????????
public
double
Price {
set
;
get
; }
????
}
|
?
1
2
3
4
5
6
7
8
9
10
|
public
class
User
???
{
???????
[KEY]
???????
public
int
id {
get
;
set
; }
???????
public
string
UserName {
get
;
set
; }
???????
public
string
Password {
get
;
set
; }
???????
public
int
Age {
get
;
set
; }
???????
public
string
PhoneNumber {
get
;
set
; }
???????
public
string
Address {
get
;
set
; }
???
}
|
6.加好標記之后,我們只需要這CreateInsertSQL<T>(T entity)這個方法中的兩個foreach循環體中加一些判斷即可
1
2
3
4
5
6
7
8
9
10
11
12
|
foreach
(PropertyInfo prop
in
props)
???????????
{
???????????????
//獲取用戶自定義標記集合
??????????
object
[] attrs = prop.GetCustomAttributes(
typeof
(KEYAttribute),
true
);
???????????????
//如果屬性上有自定義標記KEYAttribute,退出本次循環
???????????
if
(attrs.Length > 0)
???????????????
{
???????????????????
continue
;
???????????????
}
???????????????
//將屬性的名字加入到字符串中
??????????
sb.Append(prop.Name +
","
);
???????????
}
|
1
2
3
4
5
6
7
8
9
|
foreach
(PropertyInfo prop
in
props)
????????????
{
????????????????
object
[] attrs = prop.GetCustomAttributes(
typeof
(KEYAttribute),
true
);
????????????????
if
(attrs.Length > 0)
????????????????
{
????????????????????
continue
;
????????????????
}
????????????????
sb.Append(
"@"
+ prop.Name +
","
);
????????????
}
|
7.測試
更多文章、技術交流、商務合作、聯系博主
微信掃碼或搜索:z360901061
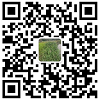
微信掃一掃加我為好友
QQ號聯系: 360901061
您的支持是博主寫作最大的動力,如果您喜歡我的文章,感覺我的文章對您有幫助,請用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點擊下面給點支持吧,站長非常感激您!手機微信長按不能支付解決辦法:請將微信支付二維碼保存到相冊,切換到微信,然后點擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對您有幫助就好】元
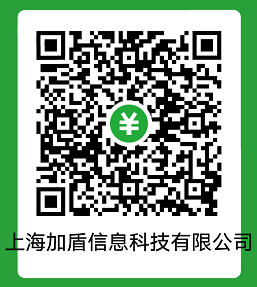