注:本文翻譯自Google官方的Android Developers Training文檔,譯者技術一般,由于喜愛安卓而產生了翻譯的念頭,純屬個人興趣愛好。
原文鏈接: http://developer.android.com/training/graphics/opengl/shapes.html
在一個OpenGL ES視圖的上下文中定義形狀,是創建你的杰作所需要的第一步。在不知道關于OpenGL ES如何期望你來定義圖形對象的基本知識的時候,通過OpenGL ES 繪圖可能會有些困難。
這節課將解釋OpenGL ES相對于Android設備屏幕的坐標系,定義形狀和形狀表面的基本知識,如定義一個三角形和一個矩形。
一). 定義一個三角形
OpenGL ES允許你使用三維空間的坐標來定義繪畫對象。所以在你能畫三角形之前,你必須先定義它的坐標。在OpenGL 中,典型的辦法是以浮點數的形式為坐標定義一個頂點數組。為了讓效率最大化,你可以將坐標寫入一個 ByteBuffer ,它將會傳入OpenGl ES的圖形處理流程中:
public class Triangle { private FloatBuffer vertexBuffer; // number of coordinates per vertex in this array static final int COORDS_PER_VERTEX = 3 ; static float triangleCoords[] = { // in counterclockwise order: 0.0f, 0.622008459f, 0.0f, // top -0.5f, -0.311004243f, 0.0f, // bottom left 0.5f, -0.311004243f, 0.0f // bottom right }; // Set color with red, green, blue and alpha (opacity) values float color[] = { 0.63671875f, 0.76953125f, 0.22265625f, 1.0f }; public Triangle() { // initialize vertex byte buffer for shape coordinates ByteBuffer bb = ByteBuffer.allocateDirect( // (number of coordinate values * 4 bytes per float) triangleCoords.length * 4 ); // use the device hardware's native byte order bb.order(ByteOrder.nativeOrder()); // create a floating point buffer from the ByteBuffer vertexBuffer = bb.asFloatBuffer(); // add the coordinates to the FloatBuffer vertexBuffer.put(triangleCoords); // set the buffer to read the first coordinate vertexBuffer.position(0 ); } }
默認情況下,OpenGL ES會假定一個坐標系,在這個坐標系中,[0, 0, 0](X, Y, Z)對應的是
GLSurfaceView
框架的中心。[1, 1, 0]對應的是框架的右上角,[-1, -1, 0]對應的則是左下角。如果想要看此坐標系的插圖說明,可以閱讀
OpenGL ES
開發手冊。
注意到這個形狀的坐標是以逆時針順序定義的。繪制的順序非常關鍵,因為它定義了那一面是形狀的正面(你希望繪制的一面),以及背面(你可以使用OpenGL ES的 cull face特性來讓它不要繪制 )。更多關于該方面的信息, 可以閱讀 OpenGL ES 開發手冊。
二). 定義一個矩形
在OpenGL中定義三角形非常簡單,那么你是否想要增加一些復雜性呢?比如,定義一個矩形?有很多方法可以用來定義矩形,不過在OpenGL ES中最典型的辦法是使用兩個三角形拼接在一起:
圖1. 使用兩個三角形畫一個矩形
再一次地,你需要以逆時針的形式為三角形頂點定義坐標來表現這個圖形,并將值放入一個
ByteBuffer
中。為了避免由兩個三角形共享的頂點被重復定義,可以使用一個繪制列表來告訴OpenGL ES圖形處理流程應該如何畫這些頂點。下面是代碼樣例:
public class Square { private FloatBuffer vertexBuffer; private ShortBuffer drawListBuffer; // number of coordinates per vertex in this array static final int COORDS_PER_VERTEX = 3 ; static float squareCoords[] = { -0.5f, 0.5f, 0.0f, // top left -0.5f, -0.5f, 0.0f, // bottom left 0.5f, -0.5f, 0.0f, // bottom right 0.5f, 0.5f, 0.0f }; // top right private short drawOrder[] = { 0, 1, 2, 0, 2, 3 }; // order to draw vertices public Square() { // initialize vertex byte buffer for shape coordinates ByteBuffer bb = ByteBuffer.allocateDirect( // (# of coordinate values * 4 bytes per float) squareCoords.length * 4 ); bb.order(ByteOrder.nativeOrder()); vertexBuffer = bb.asFloatBuffer(); vertexBuffer.put(squareCoords); vertexBuffer.position( 0 ); // initialize byte buffer for the draw list ByteBuffer dlb = ByteBuffer.allocateDirect( // (# of coordinate values * 2 bytes per short) drawOrder.length * 2 ); dlb.order(ByteOrder.nativeOrder()); drawListBuffer = dlb.asShortBuffer(); drawListBuffer.put(drawOrder); drawListBuffer.position( 0 ); } }
該樣例給了你一個如何使用OpenGL創建復雜圖形的啟發,通常來說,你需要使用三角形的集合來繪制對象。在下一節課中,你將學習如何在屏幕上畫這些形狀。
更多文章、技術交流、商務合作、聯系博主
微信掃碼或搜索:z360901061
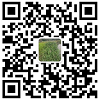
微信掃一掃加我為好友
QQ號聯系: 360901061
您的支持是博主寫作最大的動力,如果您喜歡我的文章,感覺我的文章對您有幫助,請用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點擊下面給點支持吧,站長非常感激您!手機微信長按不能支付解決辦法:請將微信支付二維碼保存到相冊,切換到微信,然后點擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對您有幫助就好】元
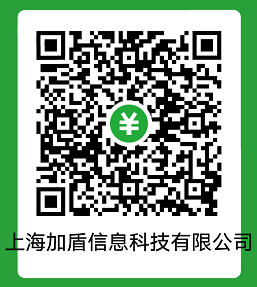