- package ?test.report; ??
- ??
- import ?java.math.BigDecimal; ??
- import ?java.sql.Connection; ??
- import ?java.sql.DriverManager; ??
- import ?java.sql.PreparedStatement; ??
- import ?java.sql.ResultSet; ??
- import ?java.sql.SQLException; ??
- import ?java.util.HashMap; ??
- ??
- import ?net.sf.jasperreports.engine.JRException; ??
- import ?net.sf.jasperreports.engine.JRResultSetDataSource; ??
- import ?net.sf.jasperreports.engine.JasperCompileManager; ??
- import ?net.sf.jasperreports.engine.JasperExportManager; ??
- import ?net.sf.jasperreports.engine.JasperFillManager; ??
- import ?net.sf.jasperreports.engine.JasperPrint; ??
- import ?net.sf.jasperreports.engine. JasperReport ; ??
- import ?net.sf.jasperreports.engine.util.JRLoader; ??
- ??
- public ? class ?GenerateReport?{ ??
- ??? public ? static ? void ?main(String[]?args)?{ ??
- ?????? try ?{ ??
- ?????????sql4Report(); ??
- ??????}? catch ?(JRException?e)?{ ??
- ?????????e.printStackTrace(); ??
- ??????}? catch ?(SQLException?e)?{ ??
- ?????????e.printStackTrace(); ??
- ??????}? finally ?{ ??
- ?????????System.out.print(? "finish" ?); ??
- ??????} ??
- ???} ??
- ???? ??
- ??? public ? static ? void ?parameter4Report?()? ??
- ?????? throws ?SQLException,?JRException?{ ??
- ?????? //report?parameter? ??
- ??????HashMap<String,?Object>?parameter?=? ??
- ????????? new ?HashMap<String,?Object>(); ??
- ??????parameter.put(? "master_location_id" ,? new ?BigDecimal( 22 )?); ??
- ??
- ??????Connection?con?=?getConnection(); ??
- ?????? JasperReport ? jasperReport ?=? ??
- ?????????JasperCompileManager.compileReport( "reports/Location.jrxml" ); ??
- ??????JasperPrint?jasperPrint?=? ??
- ?????????JasperFillManager.fillReport(? jasperReport ,?parameter,?con?); ??
- ???????? ??
- ??????JasperExportManager.exportReportToPdfFile( ??
- ?????????jasperPrint,? "reports/location_parameter4Report.pdf" );????? ??
- ???} ??
- ???? ??
- ??? public ? static ? void ?sql4Report?()? throws ?SQLException,?JRException?{ ??
- ?????? //create?the?ResultSet? ??
- ??????Connection?con?=?getConnection(); ??
- ??????PreparedStatement?statement?=? ??
- ?????????con.prepareStatement(? ??
- ???????????? "select?*?from?loc_location?" ??
- ??????????+? "where?location_type_cd?=?'ROOM'?" ??
- ??????????+? "order?by?location_type_cd" ? ??
- ?????????); ??
- ??????ResultSet?resultSet?=?statement.executeQuery(); ??
- ???????? ??
- ??????JRResultSetDataSource?result?=? ??
- ????????? new ?JRResultSetDataSource(?resultSet?); ??
- ?????? JasperReport ? jasperReport ?=? ??
- ?????????JasperCompileManager.compileReport( "reports/Location.jrxml" ); ??
- ??????JasperPrint?jasperPrint?=? ??
- ?????????JasperFillManager.fillReport(? ??
- ???????????? jasperReport ,? new ?HashMap(),?result? ??
- ?????????); ??
- ???????? ??
- ??????JasperExportManager.exportReportToPdfFile( ??
- ?????????jasperPrint,? "reports/location_sql4Report.pdf" ??
- ??????); ??
- ???} ??
- ???? ??
- ??? public ? static ? void ?jasper4Report?()? ??
- ?????? throws ?SQLException,?JRException?{ ??
- ?????? //create?the?ResultSet? ??
- ??????Connection?con?=?getConnection(); ??
- ??????PreparedStatement?statement?=? ??
- ?????????con.prepareStatement(? ??
- ???????????? "select?*?from?loc_location?" ??
- ??????????+? "where?location_type_cd?=?'ROOM'?" ??
- ??????????+? "order?by?location_type_cd" ? ??
- ?????????); ??
- ??????ResultSet?resultSet?=?statement.executeQuery(); ??
- ???????? ??
- ??????JRResultSetDataSource?result?=? ??
- ????????? new ?JRResultSetDataSource(?resultSet?); ??
- ?????? //modify? ??
- ?????? JasperReport ? jasperReport ?=? ??
- ?????????( JasperReport )?JRLoader.loadObject( ??
- ???????????? "reports/Location.jasper" ??
- ?????????); ??
- ??????JasperPrint?jasperPrint?=? ??
- ?????????JasperFillManager.fillReport(? ??
- ???????????? jasperReport ,? new ?HashMap(),?result ??
- ?????????); ??
- ???????? ??
- ??????JasperExportManager.exportReportToPdfFile( ??
- ?????????jasperPrint,? "reports/location_jasper4Report.pdf" ??
- ??????); ??
- ???} ??
- ??
- ??? public ? static ? void ?xls4Report?()? throws ?SQLException,?JRException?{ ??
- //????create?the?ResultSet? ??
- ??????Connection?con?=?getConnection(); ??
- ??????PreparedStatement?statement?=? ??
- ?????????con.prepareStatement( ??
- ???????????? "select?*?from?loc_location?" ??
- ??????????+? "where?location_type_cd?=?'ROOM'?" ??
- ??????????+? "order?by?location_type_cd" ? ??
- ?????????); ??
- ??????ResultSet?resultSet?=?statement.executeQuery(); ??
- ???????? ??
- ???????? ??
- ??????JRResultSetDataSource?result?=? ??
- ????????? new ?JRResultSetDataSource(?resultSet?); ??
- //????create? JasperReport ?from?.jasper? ??
- ?????? JasperReport ? jasperReport ?=? ??
- ?????????( JasperReport )?JRLoader.loadObject( "reports/Location.jasper" ); ??
- ??????JasperPrint?jasperPrint?=? ??
- ?????????JasperFillManager.fillReport(? jasperReport ,? new ?HashMap(),?result?); ??
- ???????? ??
- //????????JasperExportManager.exportReportToPdfFile(?jasperPrint,?"reports/location_xls4Report.xls");? ??
- ???????? ??
- ??????JRXlsExporter?xlsExporter?=? new ?JRXlsExporter(); ??
- ??????xlsExporter.setParameter(?JRExporterParameter.JASPER_PRINT,?jasperPrint?); ??
- ??????xlsExporter.setParameter(?JRExporterParameter.OUTPUT_FILE,? new ?File( "reports/location_xls4Report.xls" )?); ??
- ??????xlsExporter.exportReport(); ??
- ???} ??
- ???? ??
- ??? private ? static ?Connection?getConnection?()? throws ?SQLException?{ ??
- ??????DriverManager.registerDriver(? ??
- ????????? new ?oracle.jdbc.driver.OracleDriver() ??
- ??????);?? ??
- ?????? return ?DriverManager.getConnection(?url,?user,?pw?); ??
- ???} ??
- ???? ??
- ??? private ? static ?String?url?=? "jdbc:oracle:thin:@localhost:1521:db" ; ??
- ??? private ? static ?String?user?=? "report" ; ??
- ??? private ? static ?String?pw???=? "report" ; ??
- }??
- package ?test.report;??
- ??
- import ?java.math.BigDecimal;??
- import ?java.sql.Connection;??
- import ?java.sql.DriverManager;??
- import ?java.sql.PreparedStatement;??
- import ?java.sql.ResultSet;??
- import ?java.sql.SQLException;??
- import ?java.util.HashMap;??
- ??
- import ?net.sf.jasperreports.engine.JRException;??
- import ?net.sf.jasperreports.engine.JRResultSetDataSource;??
- import ?net.sf.jasperreports.engine.JasperCompileManager;??
- import ?net.sf.jasperreports.engine.JasperExportManager;??
- import ?net.sf.jasperreports.engine.JasperFillManager;??
- import ?net.sf.jasperreports.engine.JasperPrint;??
- import ?net.sf.jasperreports.engine.<span? class = "hilite1" ><span?style= "background-color:?#ffff00;" >JasperReport</span></span>;??
- import ?net.sf.jasperreports.engine.util.JRLoader;??
- ??
- public ? class ?GenerateReport?{??
- ??? public ? static ? void ?main(String[]?args)?{??
- ?????? try ?{??
- ?????????sql4Report();??
- ??????}? catch ?(JRException?e)?{??
- ?????????e.printStackTrace();??
- ??????}? catch ?(SQLException?e)?{??
- ?????????e.printStackTrace();??
- ??????}? finally ?{??
- ?????????System.out.print(? "finish" ?);??
- ??????}??
- ???}??
- ??????
- ??? public ? static ? void ?parameter4Report?()???
- ?????? throws ?SQLException,?JRException?{??
- ?????? //report?parameter ??
- ??????HashMap<String,?Object>?parameter?=???
- ????????? new ?HashMap<String,?Object>();??
- ??????parameter.put(? "master_location_id" ,? new ?BigDecimal( 22 )?);??
- ??
- ??????Connection?con?=?getConnection();??
- ??????<span? class = "hilite1" ><span?style= "background-color:?#ffff00;" >JasperReport</span></span>?<span? class = "hilite1" ><span?style= "background-color:?#ffff00;" >jasperReport</span></span>?=???
- ?????????JasperCompileManager.compileReport( "reports/Location.jrxml" );??
- ??????JasperPrint?jasperPrint?=???
- ?????????JasperFillManager.fillReport(?<span? class = "hilite1" ><span?style= "background-color:?#ffff00;" >jasperReport</span></span>,?parameter,?con?);??
- ??????????
- ??????JasperExportManager.exportReportToPdfFile(??
- ?????????jasperPrint,? "reports/location_parameter4Report.pdf" );???????
- ???}??
- ??????
- ??? public ? static ? void ?sql4Report?()? throws ?SQLException,?JRException?{??
- ?????? //create?the?ResultSet ??
- ??????Connection?con?=?getConnection();??
- ??????PreparedStatement?statement?=???
- ?????????con.prepareStatement(???
- ???????????? "select?*?from?loc_location?" ??
- ??????????+? "where?location_type_cd?=?'ROOM'?" ??
- ??????????+? "order?by?location_type_cd" ???
- ?????????);??
- ??????ResultSet?resultSet?=?statement.executeQuery();??
- ??????????
- ??????JRResultSetDataSource?result?=???
- ????????? new ?JRResultSetDataSource(?resultSet?);??
- ??????<span? class = "hilite1" ><span?style= "background-color:?#ffff00;" >JasperReport</span></span>?<span? class = "hilite1" ><span?style= "background-color:?#ffff00;" >jasperReport</span></span>?=???
- ?????????JasperCompileManager.compileReport( "reports/Location.jrxml" );??
- ??????JasperPrint?jasperPrint?=???
- ?????????JasperFillManager.fillReport(???
- ????????????<span? class = "hilite1" ><span?style= "background-color:?#ffff00;" >jasperReport</span></span>,? new ?HashMap(),?result???
- ?????????);??
- ??????????
- ??????JasperExportManager.exportReportToPdfFile(??
- ?????????jasperPrint,? "reports/location_sql4Report.pdf" ??
- ??????);??
- ???}??
- ??????
- ??? public ? static ? void ?jasper4Report?()???
- ?????? throws ?SQLException,?JRException?{??
- ?????? //create?the?ResultSet ??
- ??????Connection?con?=?getConnection();??
- ??????PreparedStatement?statement?=???
- ?????????con.prepareStatement(???
- ???????????? "select?*?from?loc_location?" ??
- ??????????+? "where?location_type_cd?=?'ROOM'?" ??
- ??????????+? "order?by?location_type_cd" ???
- ?????????);??
- ??????ResultSet?resultSet?=?statement.executeQuery();??
- ??????????
- ??????JRResultSetDataSource?result?=???
- ????????? new ?JRResultSetDataSource(?resultSet?);??
- ?????? //modify ??
- ??????<span? class = "hilite1" ><span?style= "background-color:?#ffff00;" >JasperReport</span></span>?<span? class = "hilite1" ><span?style= "background-color:?#ffff00;" >jasperReport</span></span>?=???
- ?????????(<span? class = "hilite1" ><span?style= "background-color:?#ffff00;" >JasperReport</span></span>)?JRLoader.loadObject(??
- ???????????? "reports/Location.jasper" ??
- ?????????);??
- ??????JasperPrint?jasperPrint?=???
- ?????????JasperFillManager.fillReport(???
- ????????????<span? class = "hilite1" ><span?style= "background-color:?#ffff00;" >jasperReport</span></span>,? new ?HashMap(),?result??
- ?????????);??
- ??????????
- ??????JasperExportManager.exportReportToPdfFile(??
- ?????????jasperPrint,? "reports/location_jasper4Report.pdf" ??
- ??????);??
- ???}??
- ??
- ??? public ? static ? void ?xls4Report?()? throws ?SQLException,?JRException?{??
- //????create?the?ResultSet ??
- ??????Connection?con?=?getConnection();??
- ??????PreparedStatement?statement?=???
- ?????????con.prepareStatement(??
- ???????????? "select?*?from?loc_location?" ??
- ??????????+? "where?location_type_cd?=?'ROOM'?" ??
- ??????????+? "order?by?location_type_cd" ???
- ?????????);??
- ??????ResultSet?resultSet?=?statement.executeQuery();??
- ??????????
- ??????????
- ??????JRResultSetDataSource?result?=???
- ????????? new ?JRResultSetDataSource(?resultSet?);??
- //????create?<span?class="hilite1"><span?style="background-color:?#ffff00;">JasperReport</span></span>?from?.jasper ??
- ??????<span? class = "hilite1" ><span?style= "background-color:?#ffff00;" >JasperReport</span></span>?<span? class = "hilite1" ><span?style= "background-color:?#ffff00;" >jasperReport</span></span>?=???
- ?????????(<span? class = "hilite1" ><span?style= "background-color:?#ffff00;" >JasperReport</span></span>)?JRLoader.loadObject( "reports/Location.jasper" );??
- ??????JasperPrint?jasperPrint?=???
- ?????????JasperFillManager.fillReport(?<span? class = "hilite1" ><span?style= "background-color:?#ffff00;" >jasperReport</span></span>,? new ?HashMap(),?result?);??
- ??????????
- //????????JasperExportManager.exportReportToPdfFile(?jasperPrint,?"reports/location_xls4Report.xls"); ??
- ??????????
- ??????JRXlsExporter?xlsExporter?=? new ?JRXlsExporter();??
- ??????xlsExporter.setParameter(?JRExporterParameter.JASPER_PRINT,?jasperPrint?);??
- ??????xlsExporter.setParameter(?JRExporterParameter.OUTPUT_FILE,? new ?File( "reports/location_xls4Report.xls" )?);??
- ??????xlsExporter.exportReport();??
- ???}??
- ??????
- ??? private ? static ?Connection?getConnection?()? throws ?SQLException?{??
- ??????DriverManager.registerDriver(???
- ????????? new ?oracle.jdbc.driver.OracleDriver()??
- ??????);????
- ?????? return ?DriverManager.getConnection(?url,?user,?pw?);??
- ???}??
- ??????
- ??? private ? static ?String?url?=? "jdbc:oracle:thin:@localhost:1521:db" ;??
- ??? private ? static ?String?user?=? "report" ;??
- ??? private ? static ?String?pw???=? "report" ;??
- }??
需要的包:?
jasperreports-3.1.2.jar?
jasperreports-3.1.2-applet.jar?
jasperreports-3.1.2-javaflow.jar?
commons-collections.jar?
commons-digester.jar?
commons-logging-1.0.4.jar?
commons-logging-api.jar?
commons-beanutils.jar?
itext-1.3.1.jar?
classes12.jar?
poi-3.0.1.jar?
poi-contrib-3.0.1-FINAL-20070705.jar?
poi-scratchpad-3.0.1-FINAL-20070705.jar?
文件結(jié)構(gòu):?
+test?
++report?
+++GenerateReport.java?
+reports?
++Location.jrxml?
++Location.jasper?
數(shù)據(jù)表要和Location.jrmal或Location.jasper(即report的設(shè)計一致就行了
更多文章、技術(shù)交流、商務(wù)合作、聯(lián)系博主
微信掃碼或搜索:z360901061
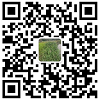
微信掃一掃加我為好友
QQ號聯(lián)系: 360901061
您的支持是博主寫作最大的動力,如果您喜歡我的文章,感覺我的文章對您有幫助,請用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點擊下面給點支持吧,站長非常感激您!手機(jī)微信長按不能支付解決辦法:請將微信支付二維碼保存到相冊,切換到微信,然后點擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對您有幫助就好】元
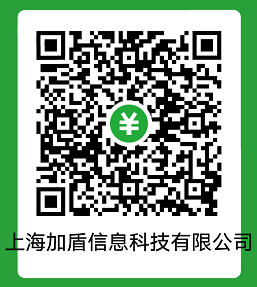