Service lifecycle--服務組件的生命周期
A service can be used in two ways--翻譯:服務組件有兩種使用方式:
??? * It can be started and allowed to run until someone stops it or it stops itself. In this mode, it's started by calling Context.startService() and stopped by calling Context.stopService(). It can stop itself by calling Service.stopSelf() or Service.stopSelfResult(). Only one stopService() call is needed to stop the service, no matter how many times startService() was called.
????? 翻譯:第一種方式:服務被啟動后一直將運行到被終止或是服務自行終止。這時候服務是被Context.startService()方法啟動的,服務的終止是被Context.stopService()方法的執(zhí)行被終止的,服務自行終止是通過執(zhí)行Service.stopSelf() 或 Service.stopSelfResult()方法的。無論服務組件實例的startService()方法被執(zhí)行了幾次,只需執(zhí)行 stopService()方法一次,服務就被終止。
??? * It can be operated programmatically using an interface that it defines and exports. Clients establish a connection to the Service object and use that connection to call into the service. The connection is established by calling Context.bindService(), and is closed by calling Context.unbindService(). Multiple clients can bind to the same service. If the service has not already been launched, bindService() can optionally launch it.
????? 翻譯:另一種方式是:借助接口。客戶端代碼創(chuàng)建一個針對服務對象的連接,然后使用該連接來調(diào)用服務。創(chuàng)建連接的方法是:Context.bindService()方法;終止服務可以調(diào)用的方法是Context.unbindService()。多個客戶程序可以綁定到同一個服務上,如果被綁定的服務還沒有啟動,可以使用bindService()方法來啟動服務。
?? The two modes are not entirely separate. You can bind to a service that was started with startService(). For example, a background music service could be started by calling startService() with an Intent object that identifies the music to play. Only later, possibly when the user wants to exercise some control over the player or get information about the current song, would an activity establish a connection to the service by calling bindService(). In cases like this, stopService() will not actually stop the service until the last binding is closed.
翻譯:這兩種方式不是說完全獨立的,我們可以綁定已經(jīng)用startService()方法啟動的服務,例如:可以執(zhí)行startService()方法啟動后臺音樂服務,在該方法的參數(shù)intent中指定了要播放的音樂。稍后,用戶可能在播放器上做些操作或是想看到正在播放的音樂的一些相關(guān)信息,那么可以有一個activity來創(chuàng)建與后臺音樂服務的連接(執(zhí)行bindService()方法),這個場合下,調(diào)用stopService()方法實際上是不能終止該服務的,除非最后一個綁定被解除。
Like an activity, a service has lifecycle methods that you can implement to monitor changes in its state. But they are fewer than the activity methods — only three — and they are public, not protected:
翻譯:和 activity 組件類似, service組件實例也有生命周期方法,可以被實現(xiàn),但是只有三個方法,這三個方法都是public,不是protected方法:
By implementing these methods, you can monitor two nested loops of the service's lifecycle:--翻譯:實現(xiàn)這三個方法可以監(jiān)聽服務組件實例生命周期中的兩個狀態(tài)切換循環(huán)過程:
??? * The entire lifetime of a service happens between the time onCreate() is called and the time onDestroy() returns. Like an activity, a service does its initial setup in onCreate(), and releases all remaining resources in onDestroy(). For example, a music playback service could create the thread where the music will be played in onCreate(), and then stop the thread in onDestroy().
????? 翻譯:整個生命期起于onCreate() 方法被調(diào)用,結(jié)束于 onDestroy()方法被執(zhí)行完成。這兩個方法作用和activity的同名方法類似,一個執(zhí)行初始化操作,一個執(zhí)行資源釋放操作。例如:音樂回放服務可以在 onCreate()方法中創(chuàng)建一個線程來執(zhí)行音樂播放操作,之后, onDestroy()方法中終止該線程。
??? * The active lifetime of a service begins with a call to onStart(). This method is handed the Intent object that was passed to startService(). The music service would open the Intent to discover which music to play, and begin the playback.
????? 翻譯: 激活期起于onStart()方法被執(zhí)行,該方法根據(jù)startService()方法的參數(shù)intent,做onStart相關(guān)的操作(音樂服務根據(jù)intent參數(shù)來查找要播放的音樂),然后執(zhí)行回放。
????? There's no equivalent callback for when the service stops — no onStop() method.
????? 翻譯:要注意的是,服務終止的時候并沒有對應的onStop()回調(diào)方法。
?? The onCreate() and onDestroy() methods are called for all services, whether they're started by Context.startService() or Context.bindService(). However, onStart() is called only for services started by startService().
翻譯:無論服務組件實例是通過 Context.startService() 方法還是通過 Context.bindService()方法被啟動的,系統(tǒng)都會調(diào)用到服務實例的 onCreate() 和 onDestroy() 方法。但是onStart()方法的調(diào)用只能針對由startService()啟動的服務。
If a service permits others to bind to it, there are additional callback methods for it to implement:---翻譯:如果某個服務允許被綁定,那么這個服務組件還有其他回調(diào)方法可以被實現(xiàn):
??? The onBind() callback is passed the Intent object that was passed to bindService and onUnbind() is handed the intent that was passed to unbindService(). If the service permits the binding, onBind() returns the communications channel that clients use to interact with the service. The onUnbind() method can ask for onRebind() to be called if a new client connects to the service.
翻譯:onBind() 回調(diào)方法的參數(shù)是一個intent對象,該對象實際上是通過 Context.bindService 方法傳入的, onUnbind()方法的參數(shù)是Context.unbindService()方法傳入的,如果服務允許綁定,那么,onBind() 返回的是客戶端需要與該服務實例交互的通訊通道對象;當有新的客戶需要和該服務連接的時候,可以通過 onUnbind() 方法來執(zhí)行 onRebind() 方法,以完成重新綁定的操作。
?? The following diagram illustrates the callback methods for a service. Although, it separates services that are created via startService from those created by bindService(), keep in mind that any service, no matter how it's started, can potentially allow clients to bind to it, so any service may receive onBind() and onUnbind() calls.
翻譯:下面用兩個圖說明了服務的回調(diào)方法,雖然這里我們是分別基于兩種啟動服務的方式(Context.startService()、Context.bindService())來做說明的,但是要時刻記住:服務組件往往都是允許被綁定,所以即使一個服務組件實例是被Context.startService方法啟動的,這個服務組件實例實際上是有onBind() 、 onUnbind()回調(diào)方法。
A service can be used in two ways--翻譯:服務組件有兩種使用方式:
??? * It can be started and allowed to run until someone stops it or it stops itself. In this mode, it's started by calling Context.startService() and stopped by calling Context.stopService(). It can stop itself by calling Service.stopSelf() or Service.stopSelfResult(). Only one stopService() call is needed to stop the service, no matter how many times startService() was called.
????? 翻譯:第一種方式:服務被啟動后一直將運行到被終止或是服務自行終止。這時候服務是被Context.startService()方法啟動的,服務的終止是被Context.stopService()方法的執(zhí)行被終止的,服務自行終止是通過執(zhí)行Service.stopSelf() 或 Service.stopSelfResult()方法的。無論服務組件實例的startService()方法被執(zhí)行了幾次,只需執(zhí)行 stopService()方法一次,服務就被終止。
??? * It can be operated programmatically using an interface that it defines and exports. Clients establish a connection to the Service object and use that connection to call into the service. The connection is established by calling Context.bindService(), and is closed by calling Context.unbindService(). Multiple clients can bind to the same service. If the service has not already been launched, bindService() can optionally launch it.
????? 翻譯:另一種方式是:借助接口。客戶端代碼創(chuàng)建一個針對服務對象的連接,然后使用該連接來調(diào)用服務。創(chuàng)建連接的方法是:Context.bindService()方法;終止服務可以調(diào)用的方法是Context.unbindService()。多個客戶程序可以綁定到同一個服務上,如果被綁定的服務還沒有啟動,可以使用bindService()方法來啟動服務。
?? The two modes are not entirely separate. You can bind to a service that was started with startService(). For example, a background music service could be started by calling startService() with an Intent object that identifies the music to play. Only later, possibly when the user wants to exercise some control over the player or get information about the current song, would an activity establish a connection to the service by calling bindService(). In cases like this, stopService() will not actually stop the service until the last binding is closed.
翻譯:這兩種方式不是說完全獨立的,我們可以綁定已經(jīng)用startService()方法啟動的服務,例如:可以執(zhí)行startService()方法啟動后臺音樂服務,在該方法的參數(shù)intent中指定了要播放的音樂。稍后,用戶可能在播放器上做些操作或是想看到正在播放的音樂的一些相關(guān)信息,那么可以有一個activity來創(chuàng)建與后臺音樂服務的連接(執(zhí)行bindService()方法),這個場合下,調(diào)用stopService()方法實際上是不能終止該服務的,除非最后一個綁定被解除。
Like an activity, a service has lifecycle methods that you can implement to monitor changes in its state. But they are fewer than the activity methods — only three — and they are public, not protected:
翻譯:和 activity 組件類似, service組件實例也有生命周期方法,可以被實現(xiàn),但是只有三個方法,這三個方法都是public,不是protected方法:
void onCreate() void onStart(Intent intent) void onDestroy()
By implementing these methods, you can monitor two nested loops of the service's lifecycle:--翻譯:實現(xiàn)這三個方法可以監(jiān)聽服務組件實例生命周期中的兩個狀態(tài)切換循環(huán)過程:
??? * The entire lifetime of a service happens between the time onCreate() is called and the time onDestroy() returns. Like an activity, a service does its initial setup in onCreate(), and releases all remaining resources in onDestroy(). For example, a music playback service could create the thread where the music will be played in onCreate(), and then stop the thread in onDestroy().
????? 翻譯:整個生命期起于onCreate() 方法被調(diào)用,結(jié)束于 onDestroy()方法被執(zhí)行完成。這兩個方法作用和activity的同名方法類似,一個執(zhí)行初始化操作,一個執(zhí)行資源釋放操作。例如:音樂回放服務可以在 onCreate()方法中創(chuàng)建一個線程來執(zhí)行音樂播放操作,之后, onDestroy()方法中終止該線程。
??? * The active lifetime of a service begins with a call to onStart(). This method is handed the Intent object that was passed to startService(). The music service would open the Intent to discover which music to play, and begin the playback.
????? 翻譯: 激活期起于onStart()方法被執(zhí)行,該方法根據(jù)startService()方法的參數(shù)intent,做onStart相關(guān)的操作(音樂服務根據(jù)intent參數(shù)來查找要播放的音樂),然后執(zhí)行回放。
????? There's no equivalent callback for when the service stops — no onStop() method.
????? 翻譯:要注意的是,服務終止的時候并沒有對應的onStop()回調(diào)方法。
?? The onCreate() and onDestroy() methods are called for all services, whether they're started by Context.startService() or Context.bindService(). However, onStart() is called only for services started by startService().
翻譯:無論服務組件實例是通過 Context.startService() 方法還是通過 Context.bindService()方法被啟動的,系統(tǒng)都會調(diào)用到服務實例的 onCreate() 和 onDestroy() 方法。但是onStart()方法的調(diào)用只能針對由startService()啟動的服務。
If a service permits others to bind to it, there are additional callback methods for it to implement:---翻譯:如果某個服務允許被綁定,那么這個服務組件還有其他回調(diào)方法可以被實現(xiàn):
IBinder onBind(Intent intent) boolean onUnbind(Intent intent) void onRebind(Intent intent)
??? The onBind() callback is passed the Intent object that was passed to bindService and onUnbind() is handed the intent that was passed to unbindService(). If the service permits the binding, onBind() returns the communications channel that clients use to interact with the service. The onUnbind() method can ask for onRebind() to be called if a new client connects to the service.
翻譯:onBind() 回調(diào)方法的參數(shù)是一個intent對象,該對象實際上是通過 Context.bindService 方法傳入的, onUnbind()方法的參數(shù)是Context.unbindService()方法傳入的,如果服務允許綁定,那么,onBind() 返回的是客戶端需要與該服務實例交互的通訊通道對象;當有新的客戶需要和該服務連接的時候,可以通過 onUnbind() 方法來執(zhí)行 onRebind() 方法,以完成重新綁定的操作。
?? The following diagram illustrates the callback methods for a service. Although, it separates services that are created via startService from those created by bindService(), keep in mind that any service, no matter how it's started, can potentially allow clients to bind to it, so any service may receive onBind() and onUnbind() calls.
翻譯:下面用兩個圖說明了服務的回調(diào)方法,雖然這里我們是分別基于兩種啟動服務的方式(Context.startService()、Context.bindService())來做說明的,但是要時刻記住:服務組件往往都是允許被綁定,所以即使一個服務組件實例是被Context.startService方法啟動的,這個服務組件實例實際上是有onBind() 、 onUnbind()回調(diào)方法。

更多文章、技術(shù)交流、商務合作、聯(lián)系博主
微信掃碼或搜索:z360901061
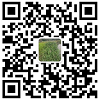
微信掃一掃加我為好友
QQ號聯(lián)系: 360901061
您的支持是博主寫作最大的動力,如果您喜歡我的文章,感覺我的文章對您有幫助,請用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點擊下面給點支持吧,站長非常感激您!手機微信長按不能支付解決辦法:請將微信支付二維碼保存到相冊,切換到微信,然后點擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對您有幫助就好】元
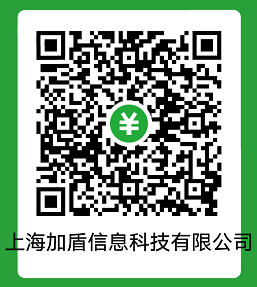