Remote procedure calls--遠程過程調(diào)用
Android has a lightweight mechanism for remote procedure calls (RPCs) — where a method is called locally, but executed remotely (in another process), with any result returned back to the caller. This entails decomposing the method call and all its attendant data to a level the operating system can understand, transmitting it from the local process and address space to the remote process and address space, and reassembling and reenacting the call there. Return values have to be transmitted in the opposite direction. Android provides all the code to do that work, so that you can concentrate on defining and implementing the RPC interface itself.
翻譯:Android提供了對遠程調(diào)用(RPC)的輕量級支持--RPC從表面上看是一個本地調(diào)用,但是實際是在另外一個進程中執(zhí)行遠程方法,執(zhí)行結(jié)果返回本地調(diào)用者。這個過程要求操作系統(tǒng)能夠?qū)Ψ椒ㄕ{(diào)用、方法參數(shù)及返回值具有解析能力;能夠把數(shù)據(jù)對象從本地進程、本地地址空間傳遞到遠程進程、遠程地址空間;而且返回值能夠逆向傳遞到本地進程中。所有這些,Android都提供了支持,作為開發(fā)者只需要關(guān)注遠程過程調(diào)用(RPC)接口的定義和實現(xiàn)。
An RPC interface can include only methods. By default, all methods are executed synchronously (the local method blocks until the remote method finishes), even if there is no return value.
翻譯:RPC接口可以只是包含方法定義,接口中的方法默認都是同步執(zhí)行(也就是說本地方法的執(zhí)行需要等待遠程方法執(zhí)行完成方可繼續(xù)執(zhí)行,即使遠程方法沒有返回值。)
In brief, the mechanism works as follows: You'd begin by declaring the RPC interface you want to implement using a simple IDL (interface definition language). From that declaration, the aidl tool generates a Java interface definition that must be made available to both the local and the remote process. It contains two inner class, as shown in the following diagram:
翻譯:簡單說,實際過程是:開發(fā)者先聲明RPC接口(使用IDL接口定義語言),然后用aidl 工具生成該接口的Java接口定義,該接口對于本地和遠程進程都必須是可見的,這個Java接口中包含兩個內(nèi)部類,如下圖所示:
The inner classes have all the code needed to administer remote procedure calls for the interface you declared with the IDL. Both inner classes implement the IBinder interface. One of them is used locally and internally by the system; the code you write can ignore it. The other, called Stub, extends the Binder class. In addition to internal code for effectuating the IPC calls, it contains declarations for the methods in the RPC interface you declared. You would subclass Stub to implement those methods, as indicated in the diagram.
翻譯:這兩個內(nèi)部類中提供了所有必要的代碼,用于基于你所定義的接口的遠程過程調(diào)用管理,這兩個類都實現(xiàn)了IBinder接口,其中一個內(nèi)部類用于本地,與操作系統(tǒng)交互。作為遠程方法的開發(fā)者可以不必考慮它,另外一個內(nèi)部類也叫Stub(存根),是Binder的子類,該類除了負責(zé)RPC調(diào)用的相關(guān)代碼外,還包含RPC接口中所聲明的方法聲明,開發(fā)者應(yīng)該把這個Stub(存根)類作為基類擴展出子類,在子類中實現(xiàn)接口定義的方法,請參考上圖。
Typically, the remote process would be managed by a service (because a service can inform the system about the process and its connections to other processes). It would have both the interface file generated by the aidl tool and the Stub subclass implementing the RPC methods. Clients of the service would have only the interface file generated by the aidl tool.
翻譯:通常,遠程進程是由一個服務(wù)來管理的(因為服務(wù)可以向系統(tǒng)通知進程以及和其他進程的連接),這樣的服務(wù)既包含了aidl工具生成的接口文件也包含遠程方法開發(fā)者由Stub類擴展出的子類實現(xiàn)的遠程方法,使用該服務(wù)的客戶端代碼只需要aidl工具生成的接口文件。
Here's how a connection between a service and its clients is set up:
下面是服務(wù)使用方和服務(wù)本身之間連接創(chuàng)建的過程:
??? * Clients of the service (on the local side) would implement onServiceConnected() and onServiceDisconnected() methods so they can be notified when a successful connection to the remote service is established, and when it goes away. They would then call bindService() to set up the connection.
????? 翻譯:服務(wù)客戶端 (運行在本地) 需要實現(xiàn) onServiceConnected() 和 onServiceDisconnected() 方法,這兩個方法在與遠程服務(wù)連接建立完成后或是連接斷開時被系統(tǒng)調(diào)用。一旦連接建立,onServiceConnected()方法繼續(xù)調(diào)用 bindService() 方法。
???
?? * The service's onBind() method would be implemented to either accept or reject the connection, depending on the intent it receives (the intent passed to bindService()). If the connection is accepted, it returns an instance of the Stub subclass.
????? 翻譯:服務(wù)的bindService()的作用要么是接受要么是拒絕連接,具體取決于方法的參數(shù)intent,如果接受連接,那么該方法返回Stub類的子類實例對象(IBinder接口的實現(xiàn)類)。
??? * If the service accepts the connection, Android calls the client's onServiceConnected() method and passes it an IBinder object, a proxy for the Stub subclass managed by the service. Through the proxy, the client can make calls on the remote service.
????? 翻譯:如果服務(wù)接受了連接,Android將立刻調(diào)用客戶端的onServiceConnected()方法,同時為該方法傳遞一個IBinder對象,這個IBinder對象是一個Stub類的子類代理的概念,由服務(wù)負責(zé)管理它,借助這個代理實例,客戶端就可以對遠程服務(wù)做調(diào)用。
This brief description omits some details of the RPC mechanism. For more information, see Designing a Remote Interface Using AIDL and the IBinder class description.
翻譯:以上簡要描述的過程中忽視了RPC過程的一些細節(jié),更多信息請查閱Designing a Remote Interface Using AIDL and the IBinder class description.
Android has a lightweight mechanism for remote procedure calls (RPCs) — where a method is called locally, but executed remotely (in another process), with any result returned back to the caller. This entails decomposing the method call and all its attendant data to a level the operating system can understand, transmitting it from the local process and address space to the remote process and address space, and reassembling and reenacting the call there. Return values have to be transmitted in the opposite direction. Android provides all the code to do that work, so that you can concentrate on defining and implementing the RPC interface itself.
翻譯:Android提供了對遠程調(diào)用(RPC)的輕量級支持--RPC從表面上看是一個本地調(diào)用,但是實際是在另外一個進程中執(zhí)行遠程方法,執(zhí)行結(jié)果返回本地調(diào)用者。這個過程要求操作系統(tǒng)能夠?qū)Ψ椒ㄕ{(diào)用、方法參數(shù)及返回值具有解析能力;能夠把數(shù)據(jù)對象從本地進程、本地地址空間傳遞到遠程進程、遠程地址空間;而且返回值能夠逆向傳遞到本地進程中。所有這些,Android都提供了支持,作為開發(fā)者只需要關(guān)注遠程過程調(diào)用(RPC)接口的定義和實現(xiàn)。
An RPC interface can include only methods. By default, all methods are executed synchronously (the local method blocks until the remote method finishes), even if there is no return value.
翻譯:RPC接口可以只是包含方法定義,接口中的方法默認都是同步執(zhí)行(也就是說本地方法的執(zhí)行需要等待遠程方法執(zhí)行完成方可繼續(xù)執(zhí)行,即使遠程方法沒有返回值。)
In brief, the mechanism works as follows: You'd begin by declaring the RPC interface you want to implement using a simple IDL (interface definition language). From that declaration, the aidl tool generates a Java interface definition that must be made available to both the local and the remote process. It contains two inner class, as shown in the following diagram:
翻譯:簡單說,實際過程是:開發(fā)者先聲明RPC接口(使用IDL接口定義語言),然后用aidl 工具生成該接口的Java接口定義,該接口對于本地和遠程進程都必須是可見的,這個Java接口中包含兩個內(nèi)部類,如下圖所示:

The inner classes have all the code needed to administer remote procedure calls for the interface you declared with the IDL. Both inner classes implement the IBinder interface. One of them is used locally and internally by the system; the code you write can ignore it. The other, called Stub, extends the Binder class. In addition to internal code for effectuating the IPC calls, it contains declarations for the methods in the RPC interface you declared. You would subclass Stub to implement those methods, as indicated in the diagram.
翻譯:這兩個內(nèi)部類中提供了所有必要的代碼,用于基于你所定義的接口的遠程過程調(diào)用管理,這兩個類都實現(xiàn)了IBinder接口,其中一個內(nèi)部類用于本地,與操作系統(tǒng)交互。作為遠程方法的開發(fā)者可以不必考慮它,另外一個內(nèi)部類也叫Stub(存根),是Binder的子類,該類除了負責(zé)RPC調(diào)用的相關(guān)代碼外,還包含RPC接口中所聲明的方法聲明,開發(fā)者應(yīng)該把這個Stub(存根)類作為基類擴展出子類,在子類中實現(xiàn)接口定義的方法,請參考上圖。
Typically, the remote process would be managed by a service (because a service can inform the system about the process and its connections to other processes). It would have both the interface file generated by the aidl tool and the Stub subclass implementing the RPC methods. Clients of the service would have only the interface file generated by the aidl tool.
翻譯:通常,遠程進程是由一個服務(wù)來管理的(因為服務(wù)可以向系統(tǒng)通知進程以及和其他進程的連接),這樣的服務(wù)既包含了aidl工具生成的接口文件也包含遠程方法開發(fā)者由Stub類擴展出的子類實現(xiàn)的遠程方法,使用該服務(wù)的客戶端代碼只需要aidl工具生成的接口文件。
Here's how a connection between a service and its clients is set up:
下面是服務(wù)使用方和服務(wù)本身之間連接創(chuàng)建的過程:
??? * Clients of the service (on the local side) would implement onServiceConnected() and onServiceDisconnected() methods so they can be notified when a successful connection to the remote service is established, and when it goes away. They would then call bindService() to set up the connection.
????? 翻譯:服務(wù)客戶端 (運行在本地) 需要實現(xiàn) onServiceConnected() 和 onServiceDisconnected() 方法,這兩個方法在與遠程服務(wù)連接建立完成后或是連接斷開時被系統(tǒng)調(diào)用。一旦連接建立,onServiceConnected()方法繼續(xù)調(diào)用 bindService() 方法。
???
?? * The service's onBind() method would be implemented to either accept or reject the connection, depending on the intent it receives (the intent passed to bindService()). If the connection is accepted, it returns an instance of the Stub subclass.
????? 翻譯:服務(wù)的bindService()的作用要么是接受要么是拒絕連接,具體取決于方法的參數(shù)intent,如果接受連接,那么該方法返回Stub類的子類實例對象(IBinder接口的實現(xiàn)類)。
??? * If the service accepts the connection, Android calls the client's onServiceConnected() method and passes it an IBinder object, a proxy for the Stub subclass managed by the service. Through the proxy, the client can make calls on the remote service.
????? 翻譯:如果服務(wù)接受了連接,Android將立刻調(diào)用客戶端的onServiceConnected()方法,同時為該方法傳遞一個IBinder對象,這個IBinder對象是一個Stub類的子類代理的概念,由服務(wù)負責(zé)管理它,借助這個代理實例,客戶端就可以對遠程服務(wù)做調(diào)用。
This brief description omits some details of the RPC mechanism. For more information, see Designing a Remote Interface Using AIDL and the IBinder class description.
翻譯:以上簡要描述的過程中忽視了RPC過程的一些細節(jié),更多信息請查閱Designing a Remote Interface Using AIDL and the IBinder class description.
Application Fundamentals--Remote procedure calls(遠程過程調(diào)用)
更多文章、技術(shù)交流、商務(wù)合作、聯(lián)系博主
微信掃碼或搜索:z360901061
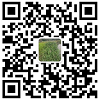
微信掃一掃加我為好友
QQ號聯(lián)系: 360901061
您的支持是博主寫作最大的動力,如果您喜歡我的文章,感覺我的文章對您有幫助,請用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點擊下面給點支持吧,站長非常感激您!手機微信長按不能支付解決辦法:請將微信支付二維碼保存到相冊,切換到微信,然后點擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對您有幫助就好】元
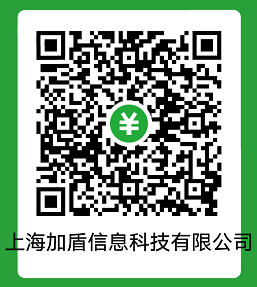