用于記錄自己寫的,或學習期間看到的不錯的,小程序,持續更新......
****************************************************************
【例001】計算:1-2+3-4..+199-200值
#encoding=utf-8?
#計算 1-2+3-4..+199-200值?
#1+3+5+7+...199?
#-2-4-6...-200?
sum1? = 0?
sum2? = 0?
for i in range(1,200,2):????? #計算1+3+5+7...199?
??? sum1 +=i?
print sum1?
?
?
for i in range(-200,0,2):??? #計算-2+(-4)+(-6)...+(-200)?
??? sum2 +=i?
print sum2?
?
?
print "The total of 1-2+3-4..+199-200 is: ", sum1+sum2?
【例002】將兩個文件中相同的部分,寫到一個文件中
#encoding=utf-8?
#Python 2.7.4?
#Purpose:? 將文件1.txt,2.txt中相同的內容放到3.txt中;?
f1 = open("1.txt","r+")?
f2 = open("2.txt","r+")?
f3 = open("3.txt","w+")?
?
?
all1 = f1.readlines()??? #先拿文件1中所有行取出?
all2 = f2.readlines()??? #再拿文件2中所有行取出?
f1.close()?
f2.close()?
?
?
for l1 in all1:?
??? for l2 in all2:?
??????? if l1.strip()==l2.strip():? #比較行中內容是否一樣?
??????????? f3.write(l2)?
??? else:?
??????? continue?
else:?
??? pass?
?????????
print "#"*40?
f3.close()?
【例003】反向讀取文件
假如要讀取的test.txt文件內容如下:?
Python?
Perl?
Java?
Shell?
實現代碼:
file1 = file('test.txt','r')?
list1 = []? #用一個空列表用于存放每行的內容?
while True:?
??? line = file1.readline()?
??? list1.append(line.strip())?
??? if len(line) == 0:?
??????? break?
for l in list1[::-1]: #反向遍歷,然后依次讀取出來?
??? print l?
?
file1.close()?
輸出結果:?
Shell?
Java?
Perl?
Python?
【例004】 往文件中所有添加指定的前綴
比如文中: print是一個函數
文本文件強制二進制編碼
就變成了下面的
01.Python 3.0:? #print是一個函數?
02.Python 3.0:? #文本文件強制二進制編碼?
#coding = gbk???? #中文編碼?
?
f_r = open('test.txt')???? #打開要處理文件?
f_w = open('file.txt','w') #創建要添加文件?
?
i = 0??? #加前綴標志位?
?
while True:?
??? i += 1?
??? line = f_r.readline()?
??? if not line:?
??????? break?
??? f_w.write('%02d'%(i) + '.Python 3.0:? #' + line)#字符串格式化及拼接技巧?
?
f_r.close()?? #關閉打開的文件句柄?
f_w.close()???
【例005】
#coding = gbk?
'''''
下面code.txt文件中內容,將
01 CN Chinese
02 IN India
03 HK HongKang
04 JP Japan
05 DE Germany
06 US United States of America
要文件的內容,每一行文件,寫到一個文件,且文件名前面兩個字段,如
文件名為:01_CN_Chinese.txt
文中內容:01 CN Chinese
知識要點:
1. ''.join 和 split函數
2. 字符的聯合
3. with語句,open文件
4. 遍歷數組
5. 切片操作
'''?
postfix = '.txt'???????????????????? #設置后綴?
?
with open('test.txt') as myfile:???? #with語句打開文件為myfile?
??? while True:????????????????????? #while循環拿文件讀出來?
??????? lines = myfile.readlines()?? #拿所有的行一次性讀取到列表中?
??????? if not lines: break????????? #沒有則中斷?
??????? for line in lines:?????????? #遍歷列表?
??????????? file_out = str('_'.join(line.split()[:])) + postfix #得到01_CN_Chinese.txt文件名?
??????????? open(file_out,'w').write(line)????????????????????? #write(line),將沒行的文件寫入新文件中?
【例006】
#coding = gbk?
'''''
#最終實現下面的過程
foos = [1.0, 2.0, 3.0, 4.0, 5.0]
bars = [100, 200, 300, 400, 500]
?
1.0 [200, 300, 400, 500]
2.0 [100, 300, 400, 500]
3.0 [100, 200, 400, 500]
4.0 [100, 200, 300, 500]
5.0 [100, 200, 300, 400]
#知識點
1. map函數的理解
2. 關鍵是切片函數的應用
?
'''?
?
foos = [1.0, 2.0, 3.0, 4.0, 5.0]?
bars = [100, 200, 300, 400, 500]?
?
def func(foo):?
??? index = foos.index(foo) #foo在foos中的索引,拿她取出來?
??? print foo,bars[:][0:index] + bars[:][index+1:]?
??? #該索引同樣在bars中相同位置,在切片的時候拿它取出,并拼接這個切片?
??? #大功告成!?
?
print map(func,foos)?
【例007】求 6! + 5! + 4! + 3! + 2! + 1!
def factorial(n):?
??? return reduce(lambda x,y: x* y, range(1,n+1))#求6!
?
print reduce(lambda x,y: x + y, [factorial(i) for i in range(1,6)]) #求6! + 5! + 4! + 3! + 2! + 1!
【例008】 根據輸入打印文件
import sys?
?
helpinfo= '''''\
This program prints files to the standard output.
Any number of files can be specified.
Options include:
--[version|VERSION|V|v]: Prints the version number
--[help?? |HELP?? |H|h]: Display the help
'''?
?
def readfile(filename):?
??? try:?
??????? f = open(filename)?
??????? while True:?
??????????? line = f.readline()?
??????????? if not line:?
??????????????? break?
??????????? print line,?
??? except:?
??????? print 'some error here'?
?
if len(sys.argv) < 2:?
??? print 'No action is needed!'?
??? sys.exit()?
?
if sys.argv[1].startswith('--'):?
??? option = sys.argv[1][2:]?
??? if option in ['version','v','V','VERSION']:?
??????? print 'Version 1.0'?
??? elif option in ['h','H','help','HELP']:?
??????? print helpinfo?
??? else:?
??????? print 'Unknown option.'?
??? sys.exit()?
?????
else:?
??? for filename in sys.argv[1:]:?
??????? readfile(filename)?
【例009】函數中args的用法
def powersum(power,*args):?
??? '''''Print each argument's power'''?
??? total = 0?
??? for item in args:?
??????? total += pow(item,power)?
??? return total?
?
print powersum(2,3,4)? # (3**2) + (4**2)?
print powersum(2,10)?? # 10**2?
print powersum(2)????? # 0**2?
【例010】匿名函數作為返回值
def repeater(n):?
??? print n?
??? return lambda s: s*n?
?
twice = repeater(2)?
?
print twice('Hello')?
print twice(5)?
【例011】備份程序
#!/usr/bin/env python?
?
import os,time?
source???? = ['/home/test/C','/home/test/shell']???????????? #源文件目錄?
target_dir = '/home/test/python'???????????????????????????? #目標文件目錄?
?
today????? = target_dir + time.strftime('%Y%m%d')??????????? #?
now??????? = time.strftime('%H%M%S')?
?
if not os.path.exists(today):???????????????????????????????? #判斷目錄是否存在?
? os.mkdir(today)???????????????????????????????????????????? #不存在的話則新建?
? print 'Successfully created directory', today?
?
target???? = today + os.sep + now + '.zip'??????????????????? #target文件格式?
zip_cmd??? = "zip -qr '%s' %s" % (target, ' '.join(source))?? #-q:安靜模式 -r遞歸模式?
#等價于 zip -qr /home/test/python20141202/142151.zip /home/test/C /home/test/shell?
if os.system(zip_cmd) == 0:???? #判斷命令是否成功執行,成功執行,返回0?
? print 'Successful back to:', target?
else:?????????????????????????? #失敗的話,打印信息?
? print 'Backup FAILED.'?
?
加comment的版本
#!/usr/bin/env python?
?
import os,time?
?
source???? = ['/home/test/C','/home/test/shell']?
target_dir = '/home/test/python'?
?
today????? = target_dir + time.strftime('%Y%m%d')?
now??????? = time.strftime('%H%M%S')?
?
comment??? = raw_input('Enter comments here-->')?? #要輸入的comment?
if len(comment) == 0:????????????????????????????? #如果沒有comment?
? target = today + os.sep + now + '.zip'?????????? #按照上面的操作執行?
else:?
? target = today + os.sep + now + '_' + comment.replace(' ','_') + '.zip'?
#如果有comment,??
?
if not os.path.exists(today):?
? os.mkdir(today)?
? print 'The backup directory created!', today?
?
zip_command = "zip -qr '%s' %s" % (target, ' '.join(source))?
?
if os.system(zip_command) == 0:?
? print 'Scuccessful backup to', target?
else:?
? print 'The backup FAILED'?
?
輸出結果 :
# python backup_ver4.py
Enter comments here-->add new example
The backup directory created! /home/test/python20141202
Scuccessful backup to /home/test/python20141202/145130_add_new_example.zip
【例012】將二進制數轉為10進制數
def func(B):?
??? I = 0?
??? while B:?
??????? I = I * 2 + (ord(B[0])-ord('0'))?
??????? B = B[1:]?
??? return I?
?
b = raw_input('Enter binary here:')?
?
print func(b)?
【例013】將列表中排除重復項并將重復的項找出
def find_duplicate(lst):?
??? tmp = []?????????????????????????????? #臨時變量,存放排除后的列表?
??? for item in lst:?????????????
??????? if not item in tmp:??????????????? #將不在tmp變量找出?
??????????? tmp.append(item)?
??????? else:?
??????????? print 'The duplicate item is:', item?
??? print 'After remove the duplicate item:',??
??? return tmp?
?
if __name__=='__main__':?
??? test = input("Enter List here:")??????? #input技巧?
??? print find_duplicate(test)?
>>>
Enter List here:[2,1,4,2]
The duplicate item is: 2
After remove the duplicate item: [2, 1, 4]
【例014】用Python中列表中append(),pop()函數實現簡單的堆棧方法:后進先出
l = []?
l.append(1)?
l.append(2)?
l.append(3)?
print l?
print l.pop()?
print l.pop()?
print l.pop()?
?????
【例015】對列表中的單詞按首字母排序
>>> words = ['apple','bat','bar','book','atom']?
>>> tmp?? = {}??????????????? #建個空字典???
>>> for word in words:?
??? letter = word[0]????? #作為字典中的鍵?
??? if letter not in tmp: #判斷首字母是否存在于字典?
??????? tmp[letter] = [word]????? #注意要添加[],很關鍵?
??? else:?
??????? tmp[letter].append(word)? #如果鍵已經存在,值列表添加?
?
?????????
>>> tmp?
{'a': ['apple', 'atom'], 'b': ['bat', 'bar', 'book']}?
【例016】對文件進行整理(除空格、TAB鍵、除#!&?等鍵),假如文本文件全為人名,并讓首字母大寫
? john black?
Jerry!?
&alice?
TOm#?
south carolina###?
mr? smith??
代碼及輸出結果如下:
import re?
?
def clean(strings):?
??? result = []?
??? for value in strings:?
??????? value = value.strip()?
??????? value = re.sub('[#!&?]','',value)?
??????? value = value.title()?
??????? result.append(value)?
??? return result?
?
with open('data.txt','a+') as myfile:?
??? lines = myfile.readlines()?
??? for line in clean(lines):?
??????? print line?
>>>??
John Black?
Jerry?
Alice?
Tom?
South Carolina?
Mr Smith?
【例017】用while循環來判斷某個數是否是質數
y = input('Enter a integer Here:')?
?
x = y / 2?
?
while x > 1:?????
??? if y % x == 0:?
??????? print y, 'has factor', x?
??????? break?
??? x -= 1?
?????
else:?
??? print y, 'is prime'?
【例018】用while實現搜索某個字符串的功能
names = ['Tom','Alice','Wendy','Jerry','Bob','Smith']?
?
while names:?
??? if names[0] == 'Jerry':?
??????? print 'Hi,', names[0]?
??????? break?
??? names = names[1:]?
?????
else:?
??? print 'Not Found!'?
?
【例019】對嵌套的序列進行處理
>>> T = ((1,2),(3,4),(5,6))?
>>> for (a,b) in T:?
...?? print a+100, b+200?
...?
101 202?
103 204?
105 206?
【例020】用for循環實現查找
source = ['sting',(3,4),100,0.1,[1,2]]?
tests? = [(3,4),3.14]?
?
for t in tests:???????????? #先是遍歷小循環?
??? for s in source:??????? #再遍歷外層循環?
??????? if s == t:?
??????????? print t, 'Found it! '?
??????????? break?
??? else:??????????????????? #else語句的位置非常關鍵,?
??????? print t, 'Not Found!'?
等價于下面這種方式
source = ['sting',(3,4),100,0.1,[1,2]]?
tests? = [(3,4),100,3.14]?
?
for t in tests:?
??? if t in source:?
??????? print t, 'Found it.'?
??? else:?
??????? print t, 'Not found.'?
【例021】用for循環來收集兩個序列中相同的部分
seq1 = 'spam'?
seq2 = 'suck'?
?
res? = []?
for s1 in seq1:?
??? if s1 in seq2:?
??????? res.append(s1)?
?
print res?
【例022】隔個取出字符串
S = 'abcdefghijklmn'?
?
for i in range(0,len(S),2):?
??? print S[i],?
?
#或者?
print S[::2]?
【例023】兩個列表,列表中每個元素加100,然后與L1中對應元素相乘,形成列表,再對列表求和
L1 = [1,2,3,4]??
L2 = [5,6,7,8] #L2每個元素加一百,105,106,107?
#(5+100)*1 + (6+100)*2 + (100+7)*3 + (100+8)*4?
# 合計: 1070?
L3 = [x+100 for x in L2]?
L4 = []?
?
for (x,y) in zip(L1,L3):?
??? L4.append(x*y)?
?????
print sum(L4)?
?
#或者用下面精簡方式,只是剛看到有點頭痛!?
print sum([x*y for x,y in [T for T in zip(L1,[x+100 for x in L2])]])
【例024】對列表進行,合并,去重,取交集等操作
def func(seq1, seq2=None, opra=None):?
??? res = []????
??? if opra?? == '-':?
??????? for item1 in seq1:?
??????????? if item1 not in seq2:?
??????????????? res.append(item1)?
?????????????????
??? elif opra == '&':?
??????? for item1 in seq1:?
??????????? if item1 in seq2:?
??????????????? res.append(item1)?
?????????????????
??? elif opra == '|':?
??????? tmp = seq1[:]?
??????? for item1 in seq2:?
??????????? if item1 not in seq1:?
??????????????? tmp.append(item1)?
??????? return tmp?
?????
??? elif opra == '^':?
?
??????? for i in seq1:?
??????????? if i not in seq2:?
??????????????? res.append(i)?
??????? for i in seq2:?
??????????? if i not in seq1:?
??????????????? res.append(i)????????????
??????? return res?
?????
??? else:?
??????? print 'Need list as input!'?
?
??? return res?
?
L1 = [1,2,3,4]?
L2 = [3,4,5,6]?
?
print '[L1 - L2]:',func(L1,L2,'-')?
print '[L1 & L2]:',func(L1,L2,'&')?
print '[L1 | L2]:',func(L1,L2,'|')?
print '[L1 ^ L2]:',func(L1,L2,'^')?
?
?
def list_remove(seq):?
??? res = []?
??? for i in seq:?
??????? if i not in res:?
??????????? res.append(i)?
??? return res?
?
L1 = [3,1,2,3,8]?
print list_remove(L1)?
?
?
def find_duplicate(seq):?
??? res = []?
??? for i in range(len(seq)):?
??????? if seq.count(seq[i]) >= 2:?
??????????? print 'Found %s'% seq[i], 'The index is:', i?
??????????? res.append(seq[i])?
??? return res?
?
L1 = [3,1,2,3,8]?
print find_duplicate(L1)?
結果如下:
>>>??
[L1 - L2]: [1, 2]?
[L1 & L2]: [3, 4]?
[L1 | L2]: [1, 2, 3, 4, 5, 6]?
[L1 ^ L2]: [1, 2, 5, 6]?
[3, 1, 2, 8]?
Found 3 The index is: 0?
Found 3 The index is: 3?
[3, 3]?
【例025】通過函數改變全局變量的三種方式
var = 99?
?
def local():?
??? var = 0?
?
def glob1():?
??? global var?
??? var += 1?
?
def glob2():?
??? var = 0?
??? import Learn?
??? Learn.var += 1?
?
def glob3():?
??? var = 0?
??? import sys?
??? glob = sys.modules['Learn']?
??? glob.var += 1?
?
def test():?
??? print var?
??? local();glob1();glob2();glob3()?
??? print var?
【例026】求range(10)中每個元素的立方
def func():?
??? res = []?
??? for i in range(10):?
??????? res.append(lambda x, i=i: i ** x) #i=i這是關鍵,否則i默認記憶最后一個值:9?
??? return res?
?
>>> res = func()?
>>> for i in range(10):?
??? res[i](3)????
0?
1?
8?
27?
64?
125?
216?
343?
512?
729?
【例027】求最小值
def min1(*args):?
??? mini = args[0]?
??? for arg in args[1:]:?
??????? if arg < mini:?
??????????? mini = arg?
??? return mini?
?
def min2(first,*rest):?
??? mini? = first?
??? for arg in rest:?
??????? if arg < first:?
??????????? mini = arg?
??? return mini?
?
def min3(*args):?
??? res = list(args)?
??? res.sort()?
??? return res[0]?
?
print min1('c','a','b')?
print min2(3,1,4)?
print min3(1,'a',78,'c')?
def func(test, *args):?
??? res = args[0]?
??? for arg in args[1:]:?
??????? if test(arg, res):?
??????????? res = arg?
??? return res?
?????????
def lessthan(x, y): return x < y?
def morethan(x, y): return x > y?
?
print func(lessthan, 4,3,1,2,9)?
print func(morethan, 4,3,1,2,9)?
【例028】求多個集合的交集及合集
def intersect(*args):?
??? res = []?
??? for x in args[0]:?
??????? for other in args[1:]:?
??????????? if x not in other:?
??????????????? break?
??????????? else:?
??????????????? res.append(x)?
??? return set(res)??????? #去除重復的部分?
?
print intersect('SPAM','SCAM','SLAM')?
?????
def union(*args):?
??? res = []?
??? for seq in args:?
??????? for item in seq:?
??????????? if not item in res:?
??????????????? res.append(item)?
??? return res?
?
print union('SA','SB','SC')?
def intersect(*args):?
??? res = []?
??? for x in args[0]:?
??????? for other in args[1:]:?
??????????? if x not in other:?
??????????????? break?
??????????? else:?
??????????????? res.append(x)?
??? #為了交互['S','S','A','A','M','M']?
??? tmp = []?
??? [tmp.append(i) for i in res if i not in tmp]?
??? return tmp?
?
print intersect('SCAM','SPAM','SLAM')?
【例029】字典的拷貝及添加
def copyDict(old):?
??? new = {}?
??? for key in old:?
??????? new[key] = old[key]?
??? return new?
?
def addDict(d1,d2):?
??? new = {}?
??? for key in d1.keys():?
??????? new[key] = d1[key]?
??? for key in d2:?
??????? new[key] = d2[key]?
??? return new?
【例030】求質數
def isPrime(y):?
??? if y < 1:?
??????? print y, 'not prime'?
??? else:?
??????? x = y // 2?
??????? while x>1:?
??????????? if y % x == 0:?
??????????????? print y, 'has factor', x?
??????????????? break?
??????????? x -= 1?
??????? else:?
??????????? print y, 'is prime!'?
【例031】比較多個值的大小
def min_max(func,*args):?
??? res = args[0]?
??? for arg in args[1:]:?
??????? if func(arg,res):?
??????????? res = arg?
??? return res?
?
def min_func(x,y): return x < y?
def max_func(x,y): return x > y?
?
if __name__=='__main__':?
??? print "The min value is:", min_max(min_func,4,3,2,1,7,6,9)?
??? print "The max value is:", min_max(max_func,4,3,2,1,7,6,9)?
?????
# 輸出結果:???????
>>>??
The min value is: 1?
The max value is: 9??
【例032】寫一個小函數實現內置函數dir的功能
#Filename: mydir.py?
?
tag = 1?
?
def listing(module):?
??? if tag:?
??????? print '-'*30?
??????? print 'name:', module.__name__,'file:', module.__file__?
??????? print '-'*30?
?
??? count = 0?
??? for attr in module.__dict__.keys():?
??????? if attr[0:2] == '__':?
??????????? print '%02d) %s' % (count, attr)?
??????? else:?
??????????? print getattr(module,attr)?
??????? count = count + 1?
?
??? if tag:?
??????? print '-'*30?
??????? print module.__name__, 'has %d names.' % count?
??????? print '-'*30?
?
if __name__=='__main__':?
??? import mydir?
??? listing(mydir)?
【例033】求分數平均值
'''''Filename: grades.txt?? 求該文件中第二列的平均值
Jerry? 78
Alice? 45
Wendy 96
Tom??? 56
Bob?? 85
'''?
?
temp = []?
for line in open('grades.txt'):?
??? a = line.strip().split()?
??? if a:?
??????? temp.append(a[1])?
?????????
#['78', '45', '96', '56', '85']?
total = 0?
for i in temp:?
??? total += int(i)?
?
print 'The total grade is:', total, 'The average is:', total/len(tmp)
【例034】一個實際類的例子
class GenericDisplay:?
??? def gatherAttrs(self):?
??????? attrs = '\n'?
??????? for key in self.__dict__:?
??????????? attrs += '\t%s=%s\n' % (key, self.__dict__[key])?
??????? return attrs?
??? def __str__(self):?
??????? return '<%s: %s>' % (self.__class__.__name__, self.gatherAttrs())?
?????????
class Person(GenericDisplay):?
??? def __init__(self, name, age):?
??????? self.name = name?
??????? self.age? = age?
??? def lastName(self):?
??????? return self.name.split()[-1]?
??? def birthDay(self):?
??????? self.age += 1?
?
class Employee(Person):?
??? def __init__(self, name, age, job=None, pay=0):?
??????? Person.__init__(self, name, age)?
??????? self.job = job?
??????? self.pay = pay?
??? def birthDay(self):?
??????? self.age += 2?
??? def giveRaise(self, percent):?
??????? self.pay *= (1.0 + percent)?
?
if __name__ == '__main__':?
??? bob = Person('Bob Smith', 40)?
??? print bob?
??? print bob.lastName()?
??? bob.birthDay()?
??? print bob?
?
??? sue = Employee('Sue Jones', 44, job='dev', pay=100000)?
??? print sue?
??? print sue.lastName?
??? sue.birthDay()?
??? sue.giveRaise(.10)?
??? print sue?
【例035】根據給定的年月日以數字方式打印出日期(February 27th, 2015)
# coding = UTF-8?
#根據給定的年月日以數字形式打印出日期?
months = [?
??? 'January' ,?
??? 'February',?
??? 'March'?? ,?
??? 'April'?? ,?
??? 'May'???? ,?
??? 'June'??? ,?
??? 'July'??? ,?
??? 'August'? ,?
??? 'September',?
??? 'October'? ,?
??? 'November' ,?
??? 'December'?
??? ]?
#以1~31的數字作為結尾的列表?
endings = ['st','nd','rd'] + 17 * ['th'] + \?
????????? ['st','nd','rd'] + 07 * ['th'] + \?
????????????????????????????????? ['st']?
?
year? = raw_input('Year: ')?
month = raw_input('Month(1-12): ')?
day?? = raw_input('Day(1-31): ')?
?
month_number = int(month)?
day_number?? = int(day)?
#月份和天數減1來獲得正確的索引?
month_name?? = months[month_number - 1]?
ordinal????? = day + endings[day_number - 1]?
?
print month_name + ' ' + ordinal + ', ' + year?
?
#輸出結果?
>>>??
Year: 2015?
Month(1-12): 2?
Day(1-31): 27?
February 27th, 2015?
【例036】在居中的盒子里打印一條語句
sentence = raw_input("Sentence: ")?
?
screen_width = 80?
text_width?? = len(sentence)?
box_width??? = text_width + 6?
left_margin? = (screen_width - box_width) // 2?
?
print?
print ' '*left_margin + '+'? + '-'*(box_width-4) + '+'?
print ' '*left_margin + '| ' + ' '*(text_width)? +' |'?
print ' '*left_margin + '| ' +????? sentence???? +' |'?
print ' '*left_margin + '| ' + ' '*(text_width)? +' |'?
print ' '*left_margin + '+'? + '-'*(box_width-4) + '+'?
print?
?
#輸出結果?
>>>??
Sentence: Welcome To Beijing!?
?
?????????????????????????? +---------------------+?
?????????????????????????? |???????????????????? |?
?????????????????????????? | Welcome To Beijing! |?
?????????????????????????? |???????????????????? |?
?????????????????????????? +---------------------+?
【例037】簡單小數據庫驗證
database = [?
??? ['Bob', '1234'],?
??? ['Tom', '2345'],?
??? ['Foo', '1478']?
??? ]?
usr = raw_input('Enter username: ')?
pwd = raw_input('Enter password: ')?
?
if [usr, pwd] in database:?
??? print 'Access Granted!'?
else:?
??? print 'Access Deny!'?
【例038】使用給定的寬度打印格式化后的價格列表
width = input('Please enter width: ')?
?
price_width = 10?
item_width? = width - price_width?
?
header_format = '%-*s%*s'?
format??????? = '%-*s%*.2f'?
?
print '=' * width?
?
print header_format % (item_width, 'Item', price_width, 'Price')?
?
print '-' * width?
?
print format % (item_width, 'Apples', price_width, 0.4)?
print format % (item_width, 'Sweets', price_width, 0.5)?
print format % (item_width, 'Pepper', price_width, 12.94)?
print format % (item_width, 'Tender', price_width, 42)?
?
print '-' * width?
輸出格式:
>>>??
Please enter width: 30?
==============================?
Item???????????????????? Price?
------------------------------?
Apples??????????????????? 0.40?
Sweets??????????????????? 0.50?
Pepper?????????????????? 12.94?
Tender?????????????????? 42.00?
------------------------------?
【例039】遍歷兩個對應列表
names?? = ['Alice', 'Bob' , 'Cherry', 'David']?
numbers = ['0000' , '1111', '2222'? , '3333' ]?
?
for index,name in enumerate(names):?
??? print '%-7s=> %s' % (name, numbers[index])?
?
#輸出結果?
>>>??
Alice? => 0000?
Bob??? => 1111?
Cherry => 2222?
David? => 3333?
當然也可以采用如下通常的做法:
names = ['Alice','Bob', 'John', 'Fred']?
ages? = [27, 23, 31, 29]?
for i in range(len(ages)):?
??? print names[i],' is ', ages[i], ' years old!'?
?
#輸出結果:?
>>>??
Alice? is? 27? years old!?
Bob? is? 23? years old!?
John? is? 31? years old!?
Fred? is? 29? years old!?
【例040】對存儲在小字典中數據進行查詢
peoples = {?
??? 'Alice':{?
????????????? 'phone'??? : '0948',?
????????????? 'address'? : 'aaaa'?
??????????? },?
??? 'Wendy':{?
????????????? 'phone'??? : '4562',?
????????????? 'address'? : 'bbbb'?
??????????? },?
??? 'David':{?
????????????? 'phone'??? : '4562',?
????????????? 'address'? : 'bbbb'?
??????????? }?
??? }?
#字典使用人名作為鍵。每個人用另外一個字典來表示,其鍵'phone'和'addr'分別表示他們的電話號碼和地址?
?
?
labels = {?
??? 'phone'?? : 'phone number',?
??? 'address' : 'address'?
??? }?
#針對電話號碼和地址使用描述性標簽,會在打印輸出時用到。?
key = ''?
name = raw_input('Name: ')?
?
?
if name in peoples:?
??? request = raw_input('Enter (p) or (a): ')?
??? if request == 'p':?
??????? key = 'phone'?
??? elif request == 'a':?
??????? key = 'address'?
??? else:?
??????? print 'Please input p(phone) an a(address)!'?
??? print "%s's %s is %s" % (name, labels[key],peoples[name][key])?
?
else:?
??? print 'Not Found!'?
或者使用字典的get()方法,更好些。完整代碼如下:
#字典使用人名作為鍵。每個人用另外一個字典來表示,其鍵'phone'和'addr'分別表示他們的電話號碼和地址??
peoples = {???
??? 'Alice':{???
????????????? 'phone'??? : '0948',???
????????????? 'address'? : 'aaaa'???
??????????? },???
??? 'Wendy':{???
????????????? 'phone'??? : '4562',???
????????????? 'address'? : 'bbbb'???
??????????? },???
??? 'David':{???
????????????? 'phone'??? : '4562',???
????????????? 'address'? : 'bbbb'???
??????????? }???
??? }???
??
???
#針對電話號碼和地址使用描述性標簽,會在打印輸出時用到。????
labels = {???
??? 'phone'?? : 'phone number',???
??? 'addr'??? : 'address'???
??? }???
?
name = raw_input('Name: ')?
#查找電話號碼還是地址??
request = raw_input('Phone number (p) or address (a)? ')?
?
#查找正確的鍵?
key = request???? #如果請求即不是p也不是a?
if request == 'p': key = 'phone'?
if request == 'a': key = 'addr'?
?
#使用get()函數提供默認值?
person = peoples.get(name,{})?
label? = labels.get(key, key)?
result = person.get(key, 'not available')?
?
print "%s's %s is %s." % (name, label, result)?
【例041】字典格式化字符串例子
template='''''
%(title)s
'''?
?
data = {'title':'My Home Page','text':'Welcome to my home page!'}?
print template % data?
?
#輸出結果:?
>>>??
?
My Home Page
??
?
【例042】需找100以內的最大平方數
from math import sqrt?
#從100開始往下找,找到即停止,最大為: 81?
for n in range(99, 0, -1):?
??? root = sqrt(n)?
??? if root == int(root):?
??????? print n?
??????? break?
【例043】用while/True, break控制輸入
while True: #一直進行下去,除非break?
??? word = raw_input('Please Enter a word: ')?
??? if not word: break?? #輸入為空的話,中斷循環?
??? print 'The word was: ' + word?
【例044】將兩個列表中首字母相同的提取出來
#將兩個列表中首字母相同的羅列在一起?
girls = ['alice', 'bernice', 'clarice']?
boys? = ['chris', 'arnold', 'bob']?
#列表推導:??
print [b+'+'+g for b in boys for g in girls if b[0] == g[0]]?
#輸出結果:?
>>>??
['chris+clarice', 'arnold+alice', 'bob+bernice']?
【例045】斐波那契數列求指定數字的列表
def fibs(x):?
??? fibs = [0, 1] # 初始值?
??? for i in range(x):?
??????? # fibs[-2]+fibs[-1]:最新一個值是前面兩個值之和?
??????? # 并用append方法將其添加在后面?
??????? fibs.append(fibs[-2]+fibs[-1])??
??? print fibs?
?????
if __name__=='__main__':?
??? num? = input('How many Fibonacci numbers do you want? ')?
??? fibs(num)?
或者用普通方法實現:
>>> def fib(max):?
??? n, a, b? = 0, 0, 1?
??? tmp_list = []?
??? while n < max:?
??????? tmp_list.append(a)?
??????? a, b = b, a+b?
??????? n += 1?
??? return tmp_list?
?
>>> fib(8)?
[0, 1, 1, 2, 3, 5, 8, 13]?
【例046】寫一個自定義列表類,讓它支持盡可能多的支持操作符號
class MyList:?
??? def __init__(self, start):?
??????? self.wrapped = [] # Make sure it's a list here?
??????? for x in start:?
??????????? self.wrapped.append(x)?
??? def __add__(self, other):?
??????? return MyList(self.wrapped + other)?
??? def __mul__(self, time):?
??????? return MyList(self.wrapped * time)?
??? def __getitem__(self, offset):?
??????? return self.wrapped[offset]?
??? def __len__(self):?
??????? return len(self.wrapped)?
??? def __getslice__(self, low, high):?
??????? return MyList(self.wrapped[low:high])?
??? def append(self, node):?
??????? self.wrapped.append(node)?
??? def __getattr__(self, name): # Other members: sort/reverse/etc?
??????? return getattr(self.wrapped, name)?
??? def __repr__(self):?
??????? return repr(self.wrapped)?
?
if __name__ == '__main__':?
??? x = MyList('spam')?
??? print x?
??? print x[2]?
??? print x[1:]?
??? print x + ['eggs']?
??? print x * 3?
??? x.append('a')?
??? x.sort()?
??? for c in x:?
??????? print c,?
?
# 輸出結果如下:?
>>>??
['s', 'p', 'a', 'm']?
a?
['p', 'a', 'm']?
['s', 'p', 'a', 'm', 'eggs']?
['s', 'p', 'a', 'm', 's', 'p', 'a', 'm', 's', 'p', 'a', 'm']?
a a m p s?
【例047】用urllib模塊來批量下載圖片
import re?
import urllib?
?
def getHtml(url):?
??? page = urllib.urlopen(url)?
??? html = page.read()?
??? return html?
?
def getImg(html):?
??? reg = r'src="(.*?\.jpg)" width'?
??? imgre = re.compile(reg)?
??? imgList = re.findall(imgre, html)?
??? x = 0????
??? for imgurl in imgList:?
??????? urllib.urlretrieve(imgurl, '%s.img' % x)?? # urlretrieve()下載圖片?
??????? x += 1?
?????????
html = getHtml("http://www.w3cschool.cc/")?
print getImg(html)?
【例048】用PyQt4實現簡單的按鈕事件(Hello,World!)
# -*- coding: utf-8 -*-?
?
import sys?
from PyQt4 import QtCore, QtGui?
?
# 自定義的窗口類?
class TestWindow(QtGui.QWidget):?
??? # 窗口初始化?
??? def __init__(self, parent = None):?
??????? super(TestWindow, self).__init__(parent)?
??????? self.setWindowTitle(u'胡桃夾子')?
?
??????? # 創建按鈕?
??????? self.pushButton = QtGui.QPushButton(u'測試按鈕')?
?
??????? # 創建文本框?
??????? self.textEdit = QtGui.QTextEdit()?
?
??????? # 創建垂直布局?
??????? layout = QtGui.QVBoxLayout()?
?
??????? # 將控件添加到布局中?
??????? layout.addWidget(self.textEdit)?
??????? layout.addWidget(self.pushButton)?
?
??????? # 設置窗口布局?
??????? self.setLayout(layout)?
?
??????? # 設置按鈕單擊動作?
??????? self.pushButton.clicked.connect(self.sayHello)?
?
??? # 按鈕動作處理?
??? def sayHello(self):?
??????? self.textEdit.setText('Hello World!')?
?
# 程序主入口?
if __name__=='__main__':?
??? app = QtGui.QApplication(sys.argv)?
??? mainWindow = TestWindow()?
??? mainWindow.show()?
??? sys.exit(app.exec_())?
【例049】用python解析文本的一個例子
假如配置文件如下, 要確保配置文件行為tablename后面的level中值給讀取出來
#FileName: data.conf?
(tablename? "A__employee__id.sql")?
(userid???? "000001")?
(number???? "100001")?
(level????? "P1")?
?
?
(tablename? "B__employee__id.sql")?
(userid???? "100001")?
(number???? "100000")?
(level????? "P2")?
?
?
(tablename? "C_other_table.sql")?
(userid???? "000001")?
(number???? "100001")?
(level????? "P2")?
?
?
(tablename? "C__employee__table.sql")?
(userid???? "000001")?
(number???? "100001")?
(level????? "P3")
代碼如下:
def parse_File(filename):?
??? tmp = []?
??? fh = open(filename)?
??? line = fh.readline()?
??? flag = 0?
??? while line:?
??????? if "tablename" in line and "__employee__" in line:?
??????????? count = 0?
??????????? flag? = 1?
??????? if flag == 1:?
??????????? count += 1?
??????????? if count == 4 and "level" in line:?
??????????????? value = line.split(r'"')[1]?
??????????????? tmp.append(value)?
??????? line = fh.readline()?
??? return tmp?
?
?
if __name__ == '__main__':?
??? print parse_File('data.conf')?
?
更多文章、技術交流、商務合作、聯系博主
微信掃碼或搜索:z360901061
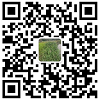
微信掃一掃加我為好友
QQ號聯系: 360901061
您的支持是博主寫作最大的動力,如果您喜歡我的文章,感覺我的文章對您有幫助,請用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點擊下面給點支持吧,站長非常感激您!手機微信長按不能支付解決辦法:請將微信支付二維碼保存到相冊,切換到微信,然后點擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對您有幫助就好】元
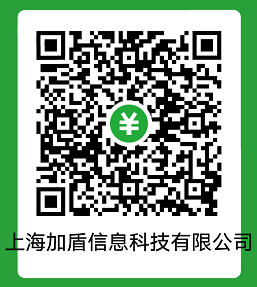