Published: May 2010
This programming task shows how to use the? UpdateListItems ?method of the? Lists ?Web service to update items in a list through a Microsoft Windows Forms application.
Use an? XmlElement ?object to create a? Batch ?element in? Collaborative Application Markup Language (CAML) ?that can contain multiple? Method ?elements, and use the? UpdateListItems(String, XmlNode) ?method to post the methods and update items.
Note |
---|
The number of list items that you can modify through the? UpdateListItems ?method in a single batch is limited to 160. |
The? Cmd ?attribute of each? Method ?element determines the operation that is performed on an item by specifying one of the following values:
-
Delete ?-- Delete an item.
-
New ?-- Create an item.
-
Update ?-- Modify an item.
-
Move ?-- Move an item.
Before you begin, create a Windows Forms application in Microsoft Visual Studio. For information about setting a Web reference to a SharePoint Foundation Web service, see? Web Service Guidelines .
To add code to update list items
-
Open? Form1 ?in Design view, open the? Toolbox , and then drag a? Button ?control onto the form.
-
Double-click the? Button ?control to display the? Code Editor , and then add the following lines of code to the Button1_Click ?event handler.
?'Declare and initialize a variable for the Lists Web service. Dim listService As New sitesWebServiceLists.Lists() 'Authenticate the current user by passing their default 'credentials to the Web service from the system credential cache. listService.Credentials = System.Net.CredentialCache.DefaultCredentials 'Set the Url property of the service for the path to a subsite. listService.Url = "http://MyServer/sites/MySiteCollection/_vti_bin/Lists.asmx" 'Get Name attribute values (GUIDs) for list and view. Dim ndListView As System.Xml.XmlNode = listService.GetListAndView( "MyList" , "" ) Dim strListID As String = ndListView.ChildNodes(0).Attributes( "Name" ).Value Dim strViewID As String = ndListView.ChildNodes(1).Attributes( "Name" ).Value 'Create an XmlDocument object and construct a Batch element and its 'attributes. Note that an empty ViewName parameter causes the method 'to use the default view. Dim doc As New System.Xml.XmlDocument() Dim batchElement As System.Xml.XmlElement = doc.CreateElement( "Batch" ) batchElement.SetAttribute( "OnError" , "Continue" ) batchElement.SetAttribute( "ListVersion" , "1" ) batchElement.SetAttribute( "ViewName" , strViewID) 'Specify methods for the batch post using CAML. To update or delete, 'specify the ID of the item, and to update or add, specify 'the value to place in the specified columns. batchElement.InnerXml = "<Method ID='1' Cmd='Update'>" + "<Field Name='ID'>6</Field>" + "<Field Name='Title'>Modified sixth item</Field></Method>" + "<Method ID='2' Cmd='Update'><Field Name='ID'>7</Field>" + "<Field Name='Title'>Modified seventh item</Field></Method>" + "<Method ID='3' Cmd='Delete'><Field Name='ID'>5</Field>" + "</Method><Method ID='4' Cmd='New'>" + "<Field Name='Title'>Added item</Field></Method>" 'Update list items. This example uses the list GUID, 'which is recommended, but the list display name will also work. listService.UpdateListItems(strListID, batchElement)
?
?/*Declare and initialize a variable for the Lists Web service.*/ sitesWebServiceLists.Lists listService = new sitesWebServiceLists.Lists(); /*Authenticate the current user by passing their default credentials to the Web service from the system credential cache.*/ listService.Credentials = System.Net.CredentialCache.DefaultCredentials; /*Set the Url property of the service for the path to a subsite.*/ listService.Url = "http://MyServer/sites/MySiteCollection/_vti_bin/Lists.asmx" ; /*Get Name attribute values (GUIDs) for list and view. */ System.Xml.XmlNode ndListView = listService.GetListAndView( "MyList" , "" ); string strListID = ndListView.ChildNodes[0].Attributes[ "Name" ].Value; string strViewID = ndListView.ChildNodes[1].Attributes[ "Name" ].Value; /*Create an XmlDocument object and construct a Batch element and its attributes. Note that an empty ViewName parameter causes the method to use the default view. */ System.Xml.XmlDocument doc = new System.Xml.XmlDocument(); System.Xml.XmlElement batchElement = doc.CreateElement( "Batch" ); batchElement.SetAttribute( "OnError" , "Continue" ); batchElement.SetAttribute( "ListVersion" , "1" ); batchElement.SetAttribute( "ViewName" , strViewID); /*Specify methods for the batch post using CAML. To update or delete, specify the ID of the item, and to update or add, specify the value to place in the specified column.*/ batchElement.InnerXml = "<Method ID='1' Cmd='Update'>" + "<Field Name='ID'>6</Field>" + "<Field Name='Title'>Modified sixth item</Field></Method>" + "<Method ID='2' Cmd='Update'><Field Name='ID'>7</Field>" + "<Field Name='Title'>Modified seventh item</Field></Method>" + "<Method ID='3' Cmd='Delete'><Field Name='ID'>5</Field>" + "</Method><Method ID='4' Cmd='New'>" + "<Field Name='Title'>Added item</Field></Method>" ; /*Update list items. This example uses the list GUID, which is recommended, but the list display name will also work.*/ try { listService.UpdateListItems(strListID, batchElement); } catch (SoapServerException ex) { MessageBox.Show(ex.Message); }
Note Posting the? UpdateListItems ?method silently fails if a specified item does not exist. The identifier (ID) for a deleted item is maintained after a delete operation. Consequently, the example deletes the fifth item in the list, but number 5 is not reassigned as the ID for another item.
-
On the? Debug ?menu, click? Start Debugging , or press? F5 , to test the form.
?
更多文章、技術交流、商務合作、聯系博主
微信掃碼或搜索:z360901061
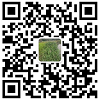
微信掃一掃加我為好友
QQ號聯系: 360901061
您的支持是博主寫作最大的動力,如果您喜歡我的文章,感覺我的文章對您有幫助,請用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點擊下面給點支持吧,站長非常感激您!手機微信長按不能支付解決辦法:請將微信支付二維碼保存到相冊,切換到微信,然后點擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對您有幫助就好】元
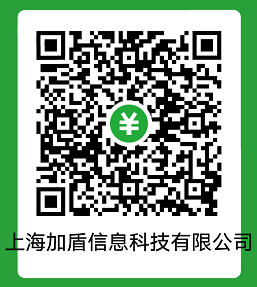